
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
lwip_snmpv3_dummy.c
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * Dummy SNMPv3 functions. 00004 */ 00005 00006 /* 00007 * Copyright (c) 2016 Elias Oenal. 00008 * All rights reserved. 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. The name of the author may not be used to endorse or promote products 00019 * derived from this software without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00022 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00023 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00024 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00025 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00026 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00027 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00028 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00029 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00030 * OF SUCH DAMAGE. 00031 * 00032 * Author: Elias Oenal <lwip@eliasoenal.com> 00033 * Dirk Ziegelmeier <dirk@ziegelmeier.net> 00034 */ 00035 00036 #include "lwip/apps/snmpv3.h" 00037 #include "snmpv3_priv.h" 00038 #include <string.h> 00039 #include "lwip/err.h" 00040 00041 #if LWIP_SNMP && LWIP_SNMP_V3 00042 00043 /** 00044 * @param username is a pointer to a string. 00045 * @param auth_algo is a pointer to u8_t. The implementation has to set this if user was found. 00046 * @param auth_key is a pointer to a pointer to a string. Implementation has to set this if user was found. 00047 * @param priv_algo is a pointer to u8_t. The implementation has to set this if user was found. 00048 * @param priv_key is a pointer to a pointer to a string. Implementation has to set this if user was found. 00049 */ 00050 err_t 00051 snmpv3_get_user (const char* username, u8_t *auth_algo, u8_t *auth_key, u8_t *priv_algo, u8_t *priv_key) 00052 { 00053 const char* engine_id; 00054 u8_t engine_id_len; 00055 00056 if(strlen(username) == 0) { 00057 return ERR_OK; 00058 } 00059 00060 if(memcmp(username, "lwip", 4) != 0) { 00061 return ERR_VAL; 00062 } 00063 00064 snmpv3_get_engine_id(&engine_id, &engine_id_len); 00065 00066 if(auth_key != NULL) { 00067 snmpv3_password_to_key_sha((const u8_t*)"maplesyrup", 10, 00068 (const u8_t*)engine_id, engine_id_len, 00069 auth_key); 00070 *auth_algo = SNMP_V3_AUTH_ALGO_SHA; 00071 } 00072 if(priv_key != NULL) { 00073 snmpv3_password_to_key_sha((const u8_t*)"maplesyrup", 10, 00074 (const u8_t*)engine_id, engine_id_len, 00075 priv_key); 00076 *priv_algo = SNMP_V3_PRIV_ALGO_DES; 00077 } 00078 return ERR_OK; 00079 } 00080 00081 /** 00082 * Get engine ID from persistence 00083 * @param id 00084 * @param len 00085 */ 00086 void 00087 snmpv3_get_engine_id(const char **id, u8_t *len) 00088 { 00089 *id = "\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x02"; 00090 *len = 12; 00091 } 00092 00093 /** 00094 * Store engine ID in persistence 00095 * @param id 00096 * @param len 00097 */ 00098 err_t 00099 snmpv3_set_engine_id(const char *id, u8_t len) 00100 { 00101 LWIP_UNUSED_ARG(id); 00102 LWIP_UNUSED_ARG(len); 00103 return ERR_OK; 00104 } 00105 00106 /** 00107 * Get engine boots from persistence. Must be increased on each boot. 00108 * @return 00109 */ 00110 u32_t 00111 snmpv3_get_engine_boots(void) 00112 { 00113 return 0; 00114 } 00115 00116 /** 00117 * Store engine boots in persistence 00118 * @param boots 00119 */ 00120 void 00121 snmpv3_set_engine_boots(u32_t boots) 00122 { 00123 LWIP_UNUSED_ARG(boots); 00124 } 00125 00126 /** 00127 * RFC3414 2.2.2. 00128 * Once the timer reaches 2147483647 it gets reset to zero and the 00129 * engine boot ups get incremented. 00130 */ 00131 u32_t 00132 snmpv3_get_engine_time(void) 00133 { 00134 return 0; 00135 } 00136 00137 /** 00138 * Reset current engine time to 0 00139 */ 00140 void 00141 snmpv3_reset_engine_time(void) 00142 { 00143 } 00144 00145 #endif /* LWIP_SNMP && LWIP_SNMP_V3 */
Generated on Sun Jul 17 2022 08:25:25 by
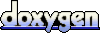