
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
lwip_snmp_mib2_icmp.c
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * Management Information Base II (RFC1213) ICMP objects and functions. 00004 */ 00005 00006 /* 00007 * Copyright (c) 2006 Axon Digital Design B.V., The Netherlands. 00008 * All rights reserved. 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. The name of the author may not be used to endorse or promote products 00019 * derived from this software without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00022 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00023 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00024 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00025 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00026 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00027 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00028 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00029 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00030 * OF SUCH DAMAGE. 00031 * 00032 * Author: Dirk Ziegelmeier <dziegel@gmx.de> 00033 * Christiaan Simons <christiaan.simons@axon.tv> 00034 */ 00035 00036 #include "lwip/snmp.h" 00037 #include "lwip/apps/snmp.h" 00038 #include "lwip/apps/snmp_core.h" 00039 #include "lwip/apps/snmp_mib2.h" 00040 #include "lwip/apps/snmp_table.h" 00041 #include "lwip/apps/snmp_scalar.h" 00042 #include "lwip/icmp.h" 00043 #include "lwip/stats.h" 00044 00045 #if LWIP_SNMP && SNMP_LWIP_MIB2 && LWIP_ICMP 00046 00047 #if SNMP_USE_NETCONN 00048 #define SYNC_NODE_NAME(node_name) node_name ## _synced 00049 #define CREATE_LWIP_SYNC_NODE(oid, node_name) \ 00050 static const struct snmp_threadsync_node node_name ## _synced = SNMP_CREATE_THREAD_SYNC_NODE(oid, &node_name.node, &snmp_mib2_lwip_locks); 00051 #else 00052 #define SYNC_NODE_NAME(node_name) node_name 00053 #define CREATE_LWIP_SYNC_NODE(oid, node_name) 00054 #endif 00055 00056 /* --- icmp .1.3.6.1.2.1.5 ----------------------------------------------------- */ 00057 00058 static s16_t 00059 icmp_get_value(const struct snmp_scalar_array_node_def *node, void *value) 00060 { 00061 u32_t *uint_ptr = (u32_t*)value; 00062 00063 switch (node->oid) { 00064 case 1: /* icmpInMsgs */ 00065 *uint_ptr = STATS_GET(mib2.icmpinmsgs); 00066 return sizeof(*uint_ptr); 00067 case 2: /* icmpInErrors */ 00068 *uint_ptr = STATS_GET(mib2.icmpinerrors); 00069 return sizeof(*uint_ptr); 00070 case 3: /* icmpInDestUnreachs */ 00071 *uint_ptr = STATS_GET(mib2.icmpindestunreachs); 00072 return sizeof(*uint_ptr); 00073 case 4: /* icmpInTimeExcds */ 00074 *uint_ptr = STATS_GET(mib2.icmpintimeexcds); 00075 return sizeof(*uint_ptr); 00076 case 5: /* icmpInParmProbs */ 00077 *uint_ptr = STATS_GET(mib2.icmpinparmprobs); 00078 return sizeof(*uint_ptr); 00079 case 6: /* icmpInSrcQuenchs */ 00080 *uint_ptr = STATS_GET(mib2.icmpinsrcquenchs); 00081 return sizeof(*uint_ptr); 00082 case 7: /* icmpInRedirects */ 00083 *uint_ptr = STATS_GET(mib2.icmpinredirects); 00084 return sizeof(*uint_ptr); 00085 case 8: /* icmpInEchos */ 00086 *uint_ptr = STATS_GET(mib2.icmpinechos); 00087 return sizeof(*uint_ptr); 00088 case 9: /* icmpInEchoReps */ 00089 *uint_ptr = STATS_GET(mib2.icmpinechoreps); 00090 return sizeof(*uint_ptr); 00091 case 10: /* icmpInTimestamps */ 00092 *uint_ptr = STATS_GET(mib2.icmpintimestamps); 00093 return sizeof(*uint_ptr); 00094 case 11: /* icmpInTimestampReps */ 00095 *uint_ptr = STATS_GET(mib2.icmpintimestampreps); 00096 return sizeof(*uint_ptr); 00097 case 12: /* icmpInAddrMasks */ 00098 *uint_ptr = STATS_GET(mib2.icmpinaddrmasks); 00099 return sizeof(*uint_ptr); 00100 case 13: /* icmpInAddrMaskReps */ 00101 *uint_ptr = STATS_GET(mib2.icmpinaddrmaskreps); 00102 return sizeof(*uint_ptr); 00103 case 14: /* icmpOutMsgs */ 00104 *uint_ptr = STATS_GET(mib2.icmpoutmsgs); 00105 return sizeof(*uint_ptr); 00106 case 15: /* icmpOutErrors */ 00107 *uint_ptr = STATS_GET(mib2.icmpouterrors); 00108 return sizeof(*uint_ptr); 00109 case 16: /* icmpOutDestUnreachs */ 00110 *uint_ptr = STATS_GET(mib2.icmpoutdestunreachs); 00111 return sizeof(*uint_ptr); 00112 case 17: /* icmpOutTimeExcds */ 00113 *uint_ptr = STATS_GET(mib2.icmpouttimeexcds); 00114 return sizeof(*uint_ptr); 00115 case 18: /* icmpOutParmProbs: not supported -> always 0 */ 00116 *uint_ptr = 0; 00117 return sizeof(*uint_ptr); 00118 case 19: /* icmpOutSrcQuenchs: not supported -> always 0 */ 00119 *uint_ptr = 0; 00120 return sizeof(*uint_ptr); 00121 case 20: /* icmpOutRedirects: not supported -> always 0 */ 00122 *uint_ptr = 0; 00123 return sizeof(*uint_ptr); 00124 case 21: /* icmpOutEchos */ 00125 *uint_ptr = STATS_GET(mib2.icmpoutechos); 00126 return sizeof(*uint_ptr); 00127 case 22: /* icmpOutEchoReps */ 00128 *uint_ptr = STATS_GET(mib2.icmpoutechoreps); 00129 return sizeof(*uint_ptr); 00130 case 23: /* icmpOutTimestamps: not supported -> always 0 */ 00131 *uint_ptr = 0; 00132 return sizeof(*uint_ptr); 00133 case 24: /* icmpOutTimestampReps: not supported -> always 0 */ 00134 *uint_ptr = 0; 00135 return sizeof(*uint_ptr); 00136 case 25: /* icmpOutAddrMasks: not supported -> always 0 */ 00137 *uint_ptr = 0; 00138 return sizeof(*uint_ptr); 00139 case 26: /* icmpOutAddrMaskReps: not supported -> always 0 */ 00140 *uint_ptr = 0; 00141 return sizeof(*uint_ptr); 00142 default: 00143 LWIP_DEBUGF(SNMP_MIB_DEBUG,("icmp_get_value(): unknown id: %"S32_F"\n", node->oid)); 00144 break; 00145 } 00146 00147 return 0; 00148 } 00149 00150 00151 static const struct snmp_scalar_array_node_def icmp_nodes[] = { 00152 { 1, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00153 { 2, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00154 { 3, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00155 { 4, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00156 { 5, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00157 { 6, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00158 { 7, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00159 { 8, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00160 { 9, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00161 {10, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00162 {11, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00163 {12, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00164 {13, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00165 {14, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00166 {15, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00167 {16, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00168 {17, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00169 {18, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00170 {19, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00171 {20, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00172 {21, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00173 {22, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00174 {23, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00175 {24, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00176 {25, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY}, 00177 {26, SNMP_ASN1_TYPE_COUNTER, SNMP_NODE_INSTANCE_READ_ONLY} 00178 }; 00179 00180 const struct snmp_scalar_array_node snmp_mib2_icmp_root = SNMP_SCALAR_CREATE_ARRAY_NODE(5, icmp_nodes, icmp_get_value, NULL, NULL); 00181 00182 #endif /* LWIP_SNMP && SNMP_LWIP_MIB2 && LWIP_ICMP */
Generated on Sun Jul 17 2022 08:25:25 by
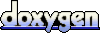