
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
etharp.h
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * Ethernet output function - handles OUTGOING ethernet level traffic, implements 00004 * ARP resolving. 00005 * To be used in most low-level netif implementations 00006 */ 00007 00008 /* 00009 * Copyright (c) 2001-2003 Swedish Institute of Computer Science. 00010 * Copyright (c) 2003-2004 Leon Woestenberg <leon.woestenberg@axon.tv> 00011 * Copyright (c) 2003-2004 Axon Digital Design B.V., The Netherlands. 00012 * All rights reserved. 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. The name of the author may not be used to endorse or promote products 00023 * derived from this software without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00026 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00027 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00028 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00029 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00030 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00031 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00032 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00033 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00034 * OF SUCH DAMAGE. 00035 * 00036 * This file is part of the lwIP TCP/IP stack. 00037 * 00038 * Author: Adam Dunkels <adam@sics.se> 00039 * 00040 */ 00041 00042 #ifndef LWIP_HDR_NETIF_ETHARP_H 00043 #define LWIP_HDR_NETIF_ETHARP_H 00044 00045 #include "lwip/opt.h" 00046 00047 #if LWIP_ARP || LWIP_ETHERNET /* don't build if not configured for use in lwipopts.h */ 00048 00049 #include "lwip/pbuf.h" 00050 #include "lwip/ip4_addr.h" 00051 #include "lwip/netif.h" 00052 #include "lwip/ip4.h" 00053 #include "lwip/prot/lwip_ethernet.h" 00054 00055 #ifdef __cplusplus 00056 extern "C" { 00057 #endif 00058 00059 #if LWIP_IPV4 && LWIP_ARP /* don't build if not configured for use in lwipopts.h */ 00060 00061 #include "lwip/prot/etharp.h" 00062 00063 /** 1 seconds period */ 00064 #define ARP_TMR_INTERVAL 1000 00065 00066 #if ARP_QUEUEING 00067 /** struct for queueing outgoing packets for unknown address 00068 * defined here to be accessed by memp.h 00069 */ 00070 struct etharp_q_entry { 00071 struct etharp_q_entry *next; 00072 struct pbuf *p; 00073 }; 00074 #endif /* ARP_QUEUEING */ 00075 00076 #define etharp_init() /* Compatibility define, no init needed. */ 00077 void etharp_tmr(void); 00078 s8_t etharp_find_addr(struct netif *netif, const ip4_addr_t *ipaddr, 00079 struct eth_addr **eth_ret, const ip4_addr_t **ip_ret); 00080 u8_t etharp_get_entry(u8_t i, ip4_addr_t **ipaddr, struct netif **netif, struct eth_addr **eth_ret); 00081 err_t etharp_output(struct netif *netif, struct pbuf *q, const ip4_addr_t *ipaddr); 00082 err_t etharp_query(struct netif *netif, const ip4_addr_t *ipaddr, struct pbuf *q); 00083 err_t etharp_request(struct netif *netif, const ip4_addr_t *ipaddr); 00084 /** For Ethernet network interfaces, we might want to send "gratuitous ARP"; 00085 * this is an ARP packet sent by a node in order to spontaneously cause other 00086 * nodes to update an entry in their ARP cache. 00087 * From RFC 3220 "IP Mobility Support for IPv4" section 4.6. */ 00088 #define etharp_gratuitous(netif) etharp_request((netif), netif_ip4_addr(netif)) 00089 void etharp_cleanup_netif(struct netif *netif); 00090 00091 #if ETHARP_SUPPORT_STATIC_ENTRIES 00092 err_t etharp_add_static_entry(const ip4_addr_t *ipaddr, struct eth_addr *ethaddr); 00093 err_t etharp_remove_static_entry(const ip4_addr_t *ipaddr); 00094 #endif /* ETHARP_SUPPORT_STATIC_ENTRIES */ 00095 00096 #endif /* LWIP_IPV4 && LWIP_ARP */ 00097 00098 void etharp_input(struct pbuf *p, struct netif *netif); 00099 00100 #ifdef __cplusplus 00101 } 00102 #endif 00103 00104 #endif /* LWIP_ARP || LWIP_ETHERNET */ 00105 00106 #endif /* LWIP_HDR_NETIF_ETHARP_H */
Generated on Sun Jul 17 2022 08:25:22 by
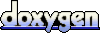