
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
lcp.h
00001 /* 00002 * lcp.h - Link Control Protocol definitions. 00003 * 00004 * Copyright (c) 1984-2000 Carnegie Mellon University. All rights reserved. 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * 2. Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in 00015 * the documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * 3. The name "Carnegie Mellon University" must not be used to 00019 * endorse or promote products derived from this software without 00020 * prior written permission. For permission or any legal 00021 * details, please contact 00022 * Office of Technology Transfer 00023 * Carnegie Mellon University 00024 * 5000 Forbes Avenue 00025 * Pittsburgh, PA 15213-3890 00026 * (412) 268-4387, fax: (412) 268-7395 00027 * tech-transfer@andrew.cmu.edu 00028 * 00029 * 4. Redistributions of any form whatsoever must retain the following 00030 * acknowledgment: 00031 * "This product includes software developed by Computing Services 00032 * at Carnegie Mellon University (http://www.cmu.edu/computing/)." 00033 * 00034 * CARNEGIE MELLON UNIVERSITY DISCLAIMS ALL WARRANTIES WITH REGARD TO 00035 * THIS SOFTWARE, INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY 00036 * AND FITNESS, IN NO EVENT SHALL CARNEGIE MELLON UNIVERSITY BE LIABLE 00037 * FOR ANY SPECIAL, INDIRECT OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES 00038 * WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN 00039 * AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING 00040 * OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE. 00041 * 00042 * $Id: lcp.h,v 1.20 2004/11/14 22:53:42 carlsonj Exp $ 00043 */ 00044 00045 #include "netif/ppp/ppp_opts.h" 00046 #if PPP_SUPPORT /* don't build if not configured for use in lwipopts.h */ 00047 00048 #ifndef LCP_H 00049 #define LCP_H 00050 00051 #include "ppp.h" 00052 00053 /* 00054 * Options. 00055 */ 00056 #define CI_VENDOR 0 /* Vendor Specific */ 00057 #define CI_MRU 1 /* Maximum Receive Unit */ 00058 #define CI_ASYNCMAP 2 /* Async Control Character Map */ 00059 #define CI_AUTHTYPE 3 /* Authentication Type */ 00060 #define CI_QUALITY 4 /* Quality Protocol */ 00061 #define CI_MAGICNUMBER 5 /* Magic Number */ 00062 #define CI_PCOMPRESSION 7 /* Protocol Field Compression */ 00063 #define CI_ACCOMPRESSION 8 /* Address/Control Field Compression */ 00064 #define CI_FCSALTERN 9 /* FCS-Alternatives */ 00065 #define CI_SDP 10 /* Self-Describing-Pad */ 00066 #define CI_NUMBERED 11 /* Numbered-Mode */ 00067 #define CI_CALLBACK 13 /* callback */ 00068 #define CI_MRRU 17 /* max reconstructed receive unit; multilink */ 00069 #define CI_SSNHF 18 /* short sequence numbers for multilink */ 00070 #define CI_EPDISC 19 /* endpoint discriminator */ 00071 #define CI_MPPLUS 22 /* Multi-Link-Plus-Procedure */ 00072 #define CI_LDISC 23 /* Link-Discriminator */ 00073 #define CI_LCPAUTH 24 /* LCP Authentication */ 00074 #define CI_COBS 25 /* Consistent Overhead Byte Stuffing */ 00075 #define CI_PREFELIS 26 /* Prefix Elision */ 00076 #define CI_MPHDRFMT 27 /* MP Header Format */ 00077 #define CI_I18N 28 /* Internationalization */ 00078 #define CI_SDL 29 /* Simple Data Link */ 00079 00080 /* 00081 * LCP-specific packet types (code numbers). 00082 */ 00083 #define PROTREJ 8 /* Protocol Reject */ 00084 #define ECHOREQ 9 /* Echo Request */ 00085 #define ECHOREP 10 /* Echo Reply */ 00086 #define DISCREQ 11 /* Discard Request */ 00087 #define IDENTIF 12 /* Identification */ 00088 #define TIMEREM 13 /* Time Remaining */ 00089 00090 /* Value used as data for CI_CALLBACK option */ 00091 #define CBCP_OPT 6 /* Use callback control protocol */ 00092 00093 #if 0 /* moved to ppp_opts.h */ 00094 #define DEFMRU 1500 /* Try for this */ 00095 #define MINMRU 128 /* No MRUs below this */ 00096 #define MAXMRU 16384 /* Normally limit MRU to this */ 00097 #endif /* moved to ppp_opts.h */ 00098 00099 /* An endpoint discriminator, used with multilink. */ 00100 #define MAX_ENDP_LEN 20 /* maximum length of discriminator value */ 00101 struct epdisc { 00102 unsigned char class_; /* -- The word "class" is reserved in C++. */ 00103 unsigned char length; 00104 unsigned char value[MAX_ENDP_LEN]; 00105 }; 00106 00107 /* 00108 * The state of options is described by an lcp_options structure. 00109 */ 00110 typedef struct lcp_options { 00111 unsigned int passive :1; /* Don't die if we don't get a response */ 00112 unsigned int silent :1; /* Wait for the other end to start first */ 00113 #if 0 /* UNUSED */ 00114 unsigned int restart :1; /* Restart vs. exit after close */ 00115 #endif /* UNUSED */ 00116 unsigned int neg_mru :1; /* Negotiate the MRU? */ 00117 unsigned int neg_asyncmap :1; /* Negotiate the async map? */ 00118 #if PAP_SUPPORT 00119 unsigned int neg_upap :1; /* Ask for UPAP authentication? */ 00120 #endif /* PAP_SUPPORT */ 00121 #if CHAP_SUPPORT 00122 unsigned int neg_chap :1; /* Ask for CHAP authentication? */ 00123 #endif /* CHAP_SUPPORT */ 00124 #if EAP_SUPPORT 00125 unsigned int neg_eap :1; /* Ask for EAP authentication? */ 00126 #endif /* EAP_SUPPORT */ 00127 unsigned int neg_magicnumber :1; /* Ask for magic number? */ 00128 unsigned int neg_pcompression :1; /* HDLC Protocol Field Compression? */ 00129 unsigned int neg_accompression :1; /* HDLC Address/Control Field Compression? */ 00130 #if LQR_SUPPORT 00131 unsigned int neg_lqr :1; /* Negotiate use of Link Quality Reports */ 00132 #endif /* LQR_SUPPORT */ 00133 unsigned int neg_cbcp :1; /* Negotiate use of CBCP */ 00134 #ifdef HAVE_MULTILINK 00135 unsigned int neg_mrru :1; /* negotiate multilink MRRU */ 00136 #endif /* HAVE_MULTILINK */ 00137 unsigned int neg_ssnhf :1; /* negotiate short sequence numbers */ 00138 unsigned int neg_endpoint :1; /* negotiate endpoint discriminator */ 00139 00140 u16_t mru; /* Value of MRU */ 00141 #ifdef HAVE_MULTILINK 00142 u16_t mrru; /* Value of MRRU, and multilink enable */ 00143 #endif /* MULTILINK */ 00144 #if CHAP_SUPPORT 00145 u8_t chap_mdtype; /* which MD types (hashing algorithm) */ 00146 #endif /* CHAP_SUPPORT */ 00147 u32_t asyncmap; /* Value of async map */ 00148 u32_t magicnumber; 00149 u8_t numloops; /* Number of loops during magic number neg. */ 00150 #if LQR_SUPPORT 00151 u32_t lqr_period; /* Reporting period for LQR 1/100ths second */ 00152 #endif /* LQR_SUPPORT */ 00153 struct epdisc endpoint; /* endpoint discriminator */ 00154 } lcp_options; 00155 00156 void lcp_open(ppp_pcb *pcb); 00157 void lcp_close(ppp_pcb *pcb, const char *reason); 00158 void lcp_lowerup(ppp_pcb *pcb); 00159 void lcp_lowerdown(ppp_pcb *pcb); 00160 void lcp_sprotrej(ppp_pcb *pcb, u_char *p, int len); /* send protocol reject */ 00161 00162 extern const struct protent lcp_protent; 00163 00164 #if 0 /* moved to ppp_opts.h */ 00165 /* Default number of times we receive our magic number from the peer 00166 before deciding the link is looped-back. */ 00167 #define DEFLOOPBACKFAIL 10 00168 #endif /* moved to ppp_opts.h */ 00169 00170 #endif /* LCP_H */ 00171 #endif /* PPP_SUPPORT */
Generated on Sun Jul 17 2022 08:25:24 by
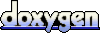