
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ip6tos_test.cpp
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "CppUTest/TestHarness.h" 00017 #include "ip6string.h" 00018 #include <stdlib.h> 00019 #include <string.h> 00020 #include <stdint.h> 00021 00022 TEST_GROUP(ip6tos) 00023 { 00024 void setup() { 00025 } 00026 00027 void teardown() { 00028 } 00029 }; 00030 00031 TEST(ip6tos, ip6_prefix_tos_func) 00032 { 00033 char prefix_str[45] = {0}; 00034 char str_len = 0; 00035 char *expected; 00036 00037 uint8_t prefix[] = { 0x14, 0x6e, 0x0a, 0x00, 0x00, 0x00, 0x00, 0x00 }; 00038 int prefix_len = 64; 00039 expected = "146e:a00::/64"; 00040 str_len = ip6_prefix_tos(prefix, prefix_len, prefix_str); 00041 CHECK(str_len == 13); 00042 STRCMP_EQUAL(expected, prefix_str); 00043 00044 memset(prefix_str, 0, 45); 00045 str_len = 0; 00046 expected = "::/0"; 00047 str_len = ip6_prefix_tos(NULL, 0, prefix_str); 00048 CHECK(str_len == 4); 00049 STRCMP_EQUAL(expected, prefix_str); 00050 00051 str_len = 0; 00052 uint8_t prefix_2[16]; 00053 memset(prefix_2, 0x88, 16); 00054 expected = "8888:8888:8888:8888:8888:8888:8888:8888/128"; 00055 str_len = ip6_prefix_tos(prefix_2, 128, prefix_str); 00056 CHECK(str_len == 43); 00057 STRCMP_EQUAL(expected, prefix_str); 00058 00059 memset(prefix_str, 0, 45); 00060 str_len = ip6_prefix_tos(prefix, 130, prefix_str); 00061 CHECK(str_len == 0); 00062 } 00063 00064 /***********************************************************/ 00065 /* Second test group for the old tests that were once lost */ 00066 00067 const char string_addr[][40] = 00068 { 00069 "2001:db8::1:0:0:1", // 1 00070 "2001:db8:aaaa:bbbb:cccc:dddd:eeee:1", // 2 00071 "2001:db8::1", // 3 00072 "2001:db8::2:1", // 4 00073 "2001:db8:aaaa:bbbb:cccc:dddd:0:1", // 5 00074 "2001:db8::aaaa:0:0:1", // 6 00075 "2001:0:0:1::1", // 7 00076 "2001:0:0:1::", // 8 00077 "2001:db8::", // 9 00078 "::aaaa:0:0:1", // 10 00079 "::1", // 11 00080 "::", // 12 00081 }; 00082 00083 00084 const uint8_t hex_addr[][16] = 00085 { 00086 { 0x20, 0x01, 0xd, 0xb8, 0,0,0,0,0,1,0,0,0,0,0,1 }, 00087 { 0x20, 0x01, 0xd, 0xb8, 0xaa, 0xaa, 0xbb, 0xbb, 0xcc, 0xcc, 0xdd, 0xdd, 0xee, 0xee, 0x00, 0x01 }, 00088 { 0x20, 0x01, 0xd, 0xb8, 0,0,0,0,0,0,0,0,0,0,0,1 }, 00089 { 0x20, 0x01, 0xd,0xb8, 0,0,0,0,0,0,0,0, 0,2,0,1 }, 00090 { 0x20, 0x01, 0xd, 0xb8, 0xaa, 0xaa, 0xbb, 0xbb, 0xcc, 0xcc, 0xdd, 0xdd, 0,0, 0x00, 0x01 }, 00091 { 0x20, 0x01, 0xd, 0xb8, 0,0,0,0,0xaa,0xaa,0,0,0,0,0,1 }, 00092 { 0x20, 0x01, 0,0 ,0,0 ,0,1,0,0,0,0,0,0,0,1 }, 00093 { 0x20, 0x01, 0,0 ,0,0 ,0,1,0,0,0,0,0,0,0,0 }, 00094 { 0x20, 0x01, 0xd, 0xb8 }, 00095 { 0,0,0,0,0,0,0,0,0xaa,0xaa,0,0,0,0,0,1 }, 00096 { 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1 }, 00097 { 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0 }, 00098 }; 00099 00100 char buf[40]; 00101 int i = 0; 00102 00103 TEST_GROUP(ip6tos_2) 00104 { 00105 void setUp(void) 00106 { 00107 memset(buf, 0, 40); 00108 } 00109 00110 void tearDown(void) 00111 { 00112 i++; 00113 } 00114 }; 00115 00116 TEST(ip6tos_2, test_1) 00117 { 00118 CHECK(strlen(string_addr[i]) == ip6tos(hex_addr[i], buf)); 00119 STRCMP_EQUAL(string_addr[i], buf); 00120 } 00121 00122 TEST(ip6tos_2, test_2) 00123 { 00124 CHECK(strlen(string_addr[i]) == ip6tos(hex_addr[i], buf)); 00125 STRCMP_EQUAL(string_addr[i], buf); 00126 } 00127 TEST(ip6tos_2, test_3) 00128 { 00129 CHECK(strlen(string_addr[i]) == ip6tos(hex_addr[i], buf)); 00130 STRCMP_EQUAL(string_addr[i], buf); 00131 } 00132 TEST(ip6tos_2, test_4) 00133 { 00134 CHECK(strlen(string_addr[i]) == ip6tos(hex_addr[i], buf)); 00135 STRCMP_EQUAL(string_addr[i], buf); 00136 } 00137 TEST(ip6tos_2, test_5) 00138 { 00139 CHECK(strlen(string_addr[i]) == ip6tos(hex_addr[i], buf)); 00140 STRCMP_EQUAL(string_addr[i], buf); 00141 } 00142 TEST(ip6tos_2, test_6) 00143 { 00144 CHECK(strlen(string_addr[i]) == ip6tos(hex_addr[i], buf)); 00145 STRCMP_EQUAL(string_addr[i], buf); 00146 } 00147 TEST(ip6tos_2, test_7) 00148 { 00149 CHECK(strlen(string_addr[i]) == ip6tos(hex_addr[i], buf)); 00150 STRCMP_EQUAL(string_addr[i], buf); 00151 } 00152 TEST(ip6tos_2, test_8) 00153 { 00154 CHECK(strlen(string_addr[i]) == ip6tos(hex_addr[i], buf)); 00155 STRCMP_EQUAL(string_addr[i], buf); 00156 } 00157 TEST(ip6tos_2, test_9) 00158 { 00159 CHECK(strlen(string_addr[i]) == ip6tos(hex_addr[i], buf)); 00160 STRCMP_EQUAL(string_addr[i], buf); 00161 } 00162 TEST(ip6tos_2, test_10) 00163 { 00164 CHECK(strlen(string_addr[i]) == ip6tos(hex_addr[i], buf)); 00165 STRCMP_EQUAL(string_addr[i], buf); 00166 } 00167 TEST(ip6tos_2, test_11) 00168 { 00169 CHECK(strlen(string_addr[i]) == ip6tos(hex_addr[i], buf)); 00170 STRCMP_EQUAL(string_addr[i], buf); 00171 } 00172 TEST(ip6tos_2, test_12) 00173 { 00174 CHECK(strlen(string_addr[i]) == ip6tos(hex_addr[i], buf)); 00175 STRCMP_EQUAL(string_addr[i], buf); 00176 }
Generated on Sun Jul 17 2022 08:25:24 by
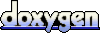