
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ip6_addr.h
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * 00004 * IPv6 addresses. 00005 */ 00006 00007 /* 00008 * Copyright (c) 2010 Inico Technologies Ltd. 00009 * All rights reserved. 00010 * 00011 * Redistribution and use in source and binary forms, with or without modification, 00012 * are permitted provided that the following conditions are met: 00013 * 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. The name of the author may not be used to endorse or promote products 00020 * derived from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00023 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00024 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00025 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00026 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00027 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00028 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00029 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00030 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00031 * OF SUCH DAMAGE. 00032 * 00033 * This file is part of the lwIP TCP/IP stack. 00034 * 00035 * Author: Ivan Delamer <delamer@inicotech.com> 00036 * 00037 * Structs and macros for handling IPv6 addresses. 00038 * 00039 * Please coordinate changes and requests with Ivan Delamer 00040 * <delamer@inicotech.com> 00041 */ 00042 #ifndef LWIP_HDR_IP6_ADDR_H 00043 #define LWIP_HDR_IP6_ADDR_H 00044 00045 #include "lwip/opt.h" 00046 #include "def.h" 00047 00048 #if LWIP_IPV6 /* don't build if not configured for use in lwipopts.h */ 00049 00050 00051 #ifdef __cplusplus 00052 extern "C" { 00053 #endif 00054 00055 00056 /** This is the aligned version of ip6_addr_t, 00057 used as local variable, on the stack, etc. */ 00058 struct ip6_addr { 00059 u32_t addr[4]; 00060 }; 00061 00062 /** IPv6 address */ 00063 typedef struct ip6_addr ip6_addr_t; 00064 00065 /** Set an IPv6 partial address given by byte-parts */ 00066 #define IP6_ADDR_PART(ip6addr, index, a,b,c,d) \ 00067 (ip6addr)->addr[index] = PP_HTONL(LWIP_MAKEU32(a,b,c,d)) 00068 00069 /** Set a full IPv6 address by passing the 4 u32_t indices in network byte order 00070 (use PP_HTONL() for constants) */ 00071 #define IP6_ADDR(ip6addr, idx0, idx1, idx2, idx3) do { \ 00072 (ip6addr)->addr[0] = idx0; \ 00073 (ip6addr)->addr[1] = idx1; \ 00074 (ip6addr)->addr[2] = idx2; \ 00075 (ip6addr)->addr[3] = idx3; } while(0) 00076 00077 /** Access address in 16-bit block */ 00078 #define IP6_ADDR_BLOCK1(ip6addr) ((u16_t)((lwip_htonl((ip6addr)->addr[0]) >> 16) & 0xffff)) 00079 /** Access address in 16-bit block */ 00080 #define IP6_ADDR_BLOCK2(ip6addr) ((u16_t)((lwip_htonl((ip6addr)->addr[0])) & 0xffff)) 00081 /** Access address in 16-bit block */ 00082 #define IP6_ADDR_BLOCK3(ip6addr) ((u16_t)((lwip_htonl((ip6addr)->addr[1]) >> 16) & 0xffff)) 00083 /** Access address in 16-bit block */ 00084 #define IP6_ADDR_BLOCK4(ip6addr) ((u16_t)((lwip_htonl((ip6addr)->addr[1])) & 0xffff)) 00085 /** Access address in 16-bit block */ 00086 #define IP6_ADDR_BLOCK5(ip6addr) ((u16_t)((lwip_htonl((ip6addr)->addr[2]) >> 16) & 0xffff)) 00087 /** Access address in 16-bit block */ 00088 #define IP6_ADDR_BLOCK6(ip6addr) ((u16_t)((lwip_htonl((ip6addr)->addr[2])) & 0xffff)) 00089 /** Access address in 16-bit block */ 00090 #define IP6_ADDR_BLOCK7(ip6addr) ((u16_t)((lwip_htonl((ip6addr)->addr[3]) >> 16) & 0xffff)) 00091 /** Access address in 16-bit block */ 00092 #define IP6_ADDR_BLOCK8(ip6addr) ((u16_t)((lwip_htonl((ip6addr)->addr[3])) & 0xffff)) 00093 00094 /** Copy IPv6 address - faster than ip6_addr_set: no NULL check */ 00095 #define ip6_addr_copy(dest, src) do{(dest).addr[0] = (src).addr[0]; \ 00096 (dest).addr[1] = (src).addr[1]; \ 00097 (dest).addr[2] = (src).addr[2]; \ 00098 (dest).addr[3] = (src).addr[3];}while(0) 00099 /** Safely copy one IPv6 address to another (src may be NULL) */ 00100 #define ip6_addr_set(dest, src) do{(dest)->addr[0] = (src) == NULL ? 0 : (src)->addr[0]; \ 00101 (dest)->addr[1] = (src) == NULL ? 0 : (src)->addr[1]; \ 00102 (dest)->addr[2] = (src) == NULL ? 0 : (src)->addr[2]; \ 00103 (dest)->addr[3] = (src) == NULL ? 0 : (src)->addr[3];}while(0) 00104 00105 /** Set complete address to zero */ 00106 #define ip6_addr_set_zero(ip6addr) do{(ip6addr)->addr[0] = 0; \ 00107 (ip6addr)->addr[1] = 0; \ 00108 (ip6addr)->addr[2] = 0; \ 00109 (ip6addr)->addr[3] = 0;}while(0) 00110 00111 /** Set address to ipv6 'any' (no need for lwip_htonl()) */ 00112 #define ip6_addr_set_any(ip6addr) ip6_addr_set_zero(ip6addr) 00113 /** Set address to ipv6 loopback address */ 00114 #define ip6_addr_set_loopback(ip6addr) do{(ip6addr)->addr[0] = 0; \ 00115 (ip6addr)->addr[1] = 0; \ 00116 (ip6addr)->addr[2] = 0; \ 00117 (ip6addr)->addr[3] = PP_HTONL(0x00000001UL);}while(0) 00118 /** Safely copy one IPv6 address to another and change byte order 00119 * from host- to network-order. */ 00120 #define ip6_addr_set_hton(dest, src) do{(dest)->addr[0] = (src) == NULL ? 0 : lwip_htonl((src)->addr[0]); \ 00121 (dest)->addr[1] = (src) == NULL ? 0 : lwip_htonl((src)->addr[1]); \ 00122 (dest)->addr[2] = (src) == NULL ? 0 : lwip_htonl((src)->addr[2]); \ 00123 (dest)->addr[3] = (src) == NULL ? 0 : lwip_htonl((src)->addr[3]);}while(0) 00124 00125 00126 /** 00127 * Determine if two IPv6 address are on the same network. 00128 * 00129 * @arg addr1 IPv6 address 1 00130 * @arg addr2 IPv6 address 2 00131 * @return !0 if the network identifiers of both address match 00132 */ 00133 #define ip6_addr_netcmp(addr1, addr2) (((addr1)->addr[0] == (addr2)->addr[0]) && \ 00134 ((addr1)->addr[1] == (addr2)->addr[1])) 00135 00136 /* Exact-host comparison *after* ip6_addr_netcmp() succeeded, for efficiency. */ 00137 #define ip6_addr_nethostcmp(addr1, addr2) (((addr1)->addr[2] == (addr2)->addr[2]) && \ 00138 ((addr1)->addr[3] == (addr2)->addr[3])) 00139 00140 #define ip6_addr_cmp(addr1, addr2) (((addr1)->addr[0] == (addr2)->addr[0]) && \ 00141 ((addr1)->addr[1] == (addr2)->addr[1]) && \ 00142 ((addr1)->addr[2] == (addr2)->addr[2]) && \ 00143 ((addr1)->addr[3] == (addr2)->addr[3])) 00144 00145 #define ip6_get_subnet_id(ip6addr) (lwip_htonl((ip6addr)->addr[2]) & 0x0000ffffUL) 00146 00147 #define ip6_addr_isany_val(ip6addr) (((ip6addr).addr[0] == 0) && \ 00148 ((ip6addr).addr[1] == 0) && \ 00149 ((ip6addr).addr[2] == 0) && \ 00150 ((ip6addr).addr[3] == 0)) 00151 #define ip6_addr_isany(ip6addr) (((ip6addr) == NULL) || ip6_addr_isany_val(*(ip6addr))) 00152 00153 #define ip6_addr_isloopback(ip6addr) (((ip6addr)->addr[0] == 0UL) && \ 00154 ((ip6addr)->addr[1] == 0UL) && \ 00155 ((ip6addr)->addr[2] == 0UL) && \ 00156 ((ip6addr)->addr[3] == PP_HTONL(0x00000001UL))) 00157 00158 #define ip6_addr_isglobal(ip6addr) (((ip6addr)->addr[0] & PP_HTONL(0xe0000000UL)) == PP_HTONL(0x20000000UL)) 00159 00160 #define ip6_addr_islinklocal(ip6addr) (((ip6addr)->addr[0] & PP_HTONL(0xffc00000UL)) == PP_HTONL(0xfe800000UL)) 00161 00162 #define ip6_addr_issitelocal(ip6addr) (((ip6addr)->addr[0] & PP_HTONL(0xffc00000UL)) == PP_HTONL(0xfec00000UL)) 00163 00164 #define ip6_addr_isuniquelocal(ip6addr) (((ip6addr)->addr[0] & PP_HTONL(0xfe000000UL)) == PP_HTONL(0xfc000000UL)) 00165 00166 #define ip6_addr_isipv4mappedipv6(ip6addr) (((ip6addr)->addr[0] == 0) && ((ip6addr)->addr[1] == 0) && (((ip6addr)->addr[2]) == PP_HTONL(0x0000FFFFUL))) 00167 00168 #define ip6_addr_ismulticast(ip6addr) (((ip6addr)->addr[0] & PP_HTONL(0xff000000UL)) == PP_HTONL(0xff000000UL)) 00169 #define ip6_addr_multicast_transient_flag(ip6addr) ((ip6addr)->addr[0] & PP_HTONL(0x00100000UL)) 00170 #define ip6_addr_multicast_prefix_flag(ip6addr) ((ip6addr)->addr[0] & PP_HTONL(0x00200000UL)) 00171 #define ip6_addr_multicast_rendezvous_flag(ip6addr) ((ip6addr)->addr[0] & PP_HTONL(0x00400000UL)) 00172 #define ip6_addr_multicast_scope(ip6addr) ((lwip_htonl((ip6addr)->addr[0]) >> 16) & 0xf) 00173 #define IP6_MULTICAST_SCOPE_RESERVED 0x0 00174 #define IP6_MULTICAST_SCOPE_RESERVED0 0x0 00175 #define IP6_MULTICAST_SCOPE_INTERFACE_LOCAL 0x1 00176 #define IP6_MULTICAST_SCOPE_LINK_LOCAL 0x2 00177 #define IP6_MULTICAST_SCOPE_RESERVED3 0x3 00178 #define IP6_MULTICAST_SCOPE_ADMIN_LOCAL 0x4 00179 #define IP6_MULTICAST_SCOPE_SITE_LOCAL 0x5 00180 #define IP6_MULTICAST_SCOPE_ORGANIZATION_LOCAL 0x8 00181 #define IP6_MULTICAST_SCOPE_GLOBAL 0xe 00182 #define IP6_MULTICAST_SCOPE_RESERVEDF 0xf 00183 #define ip6_addr_ismulticast_iflocal(ip6addr) (((ip6addr)->addr[0] & PP_HTONL(0xff8f0000UL)) == PP_HTONL(0xff010000UL)) 00184 #define ip6_addr_ismulticast_linklocal(ip6addr) (((ip6addr)->addr[0] & PP_HTONL(0xff8f0000UL)) == PP_HTONL(0xff020000UL)) 00185 #define ip6_addr_ismulticast_adminlocal(ip6addr) (((ip6addr)->addr[0] & PP_HTONL(0xff8f0000UL)) == PP_HTONL(0xff040000UL)) 00186 #define ip6_addr_ismulticast_sitelocal(ip6addr) (((ip6addr)->addr[0] & PP_HTONL(0xff8f0000UL)) == PP_HTONL(0xff050000UL)) 00187 #define ip6_addr_ismulticast_orglocal(ip6addr) (((ip6addr)->addr[0] & PP_HTONL(0xff8f0000UL)) == PP_HTONL(0xff080000UL)) 00188 #define ip6_addr_ismulticast_global(ip6addr) (((ip6addr)->addr[0] & PP_HTONL(0xff8f0000UL)) == PP_HTONL(0xff0e0000UL)) 00189 00190 /* @todo define get/set for well-know multicast addresses, e.g. ff02::1 */ 00191 #define ip6_addr_isallnodes_iflocal(ip6addr) (((ip6addr)->addr[0] == PP_HTONL(0xff010000UL)) && \ 00192 ((ip6addr)->addr[1] == 0UL) && \ 00193 ((ip6addr)->addr[2] == 0UL) && \ 00194 ((ip6addr)->addr[3] == PP_HTONL(0x00000001UL))) 00195 00196 #define ip6_addr_isallnodes_linklocal(ip6addr) (((ip6addr)->addr[0] == PP_HTONL(0xff020000UL)) && \ 00197 ((ip6addr)->addr[1] == 0UL) && \ 00198 ((ip6addr)->addr[2] == 0UL) && \ 00199 ((ip6addr)->addr[3] == PP_HTONL(0x00000001UL))) 00200 #define ip6_addr_set_allnodes_linklocal(ip6addr) do{(ip6addr)->addr[0] = PP_HTONL(0xff020000UL); \ 00201 (ip6addr)->addr[1] = 0; \ 00202 (ip6addr)->addr[2] = 0; \ 00203 (ip6addr)->addr[3] = PP_HTONL(0x00000001UL);}while(0) 00204 00205 #define ip6_addr_isallrouters_linklocal(ip6addr) (((ip6addr)->addr[0] == PP_HTONL(0xff020000UL)) && \ 00206 ((ip6addr)->addr[1] == 0UL) && \ 00207 ((ip6addr)->addr[2] == 0UL) && \ 00208 ((ip6addr)->addr[3] == PP_HTONL(0x00000002UL))) 00209 #define ip6_addr_set_allrouters_linklocal(ip6addr) do{(ip6addr)->addr[0] = PP_HTONL(0xff020000UL); \ 00210 (ip6addr)->addr[1] = 0; \ 00211 (ip6addr)->addr[2] = 0; \ 00212 (ip6addr)->addr[3] = PP_HTONL(0x00000002UL);}while(0) 00213 00214 #define ip6_addr_issolicitednode(ip6addr) ( ((ip6addr)->addr[0] == PP_HTONL(0xff020000UL)) && \ 00215 ((ip6addr)->addr[2] == PP_HTONL(0x00000001UL)) && \ 00216 (((ip6addr)->addr[3] & PP_HTONL(0xff000000UL)) == PP_HTONL(0xff000000UL)) ) 00217 00218 #define ip6_addr_set_solicitednode(ip6addr, if_id) do{(ip6addr)->addr[0] = PP_HTONL(0xff020000UL); \ 00219 (ip6addr)->addr[1] = 0; \ 00220 (ip6addr)->addr[2] = PP_HTONL(0x00000001UL); \ 00221 (ip6addr)->addr[3] = (PP_HTONL(0xff000000UL) | (if_id));}while(0) 00222 00223 #define ip6_addr_cmp_solicitednode(ip6addr, sn_addr) (((ip6addr)->addr[0] == PP_HTONL(0xff020000UL)) && \ 00224 ((ip6addr)->addr[1] == 0) && \ 00225 ((ip6addr)->addr[2] == PP_HTONL(0x00000001UL)) && \ 00226 ((ip6addr)->addr[3] == (PP_HTONL(0xff000000UL) | (sn_addr)->addr[3]))) 00227 00228 /* IPv6 address states. */ 00229 #define IP6_ADDR_INVALID 0x00 00230 #define IP6_ADDR_TENTATIVE 0x08 00231 #define IP6_ADDR_TENTATIVE_1 0x09 /* 1 probe sent */ 00232 #define IP6_ADDR_TENTATIVE_2 0x0a /* 2 probes sent */ 00233 #define IP6_ADDR_TENTATIVE_3 0x0b /* 3 probes sent */ 00234 #define IP6_ADDR_TENTATIVE_4 0x0c /* 4 probes sent */ 00235 #define IP6_ADDR_TENTATIVE_5 0x0d /* 5 probes sent */ 00236 #define IP6_ADDR_TENTATIVE_6 0x0e /* 6 probes sent */ 00237 #define IP6_ADDR_TENTATIVE_7 0x0f /* 7 probes sent */ 00238 #define IP6_ADDR_VALID 0x10 /* This bit marks an address as valid (preferred or deprecated) */ 00239 #define IP6_ADDR_PREFERRED 0x30 00240 #define IP6_ADDR_DEPRECATED 0x10 /* Same as VALID (valid but not preferred) */ 00241 #define IP6_ADDR_DUPLICATED 0x40 /* Failed DAD test, not valid */ 00242 00243 #define IP6_ADDR_TENTATIVE_COUNT_MASK 0x07 /* 1-7 probes sent */ 00244 00245 #define ip6_addr_isinvalid(addr_state) (addr_state == IP6_ADDR_INVALID) 00246 #define ip6_addr_istentative(addr_state) (addr_state & IP6_ADDR_TENTATIVE) 00247 #define ip6_addr_isvalid(addr_state) (addr_state & IP6_ADDR_VALID) /* Include valid, preferred, and deprecated. */ 00248 #define ip6_addr_ispreferred(addr_state) (addr_state == IP6_ADDR_PREFERRED) 00249 #define ip6_addr_isdeprecated(addr_state) (addr_state == IP6_ADDR_DEPRECATED) 00250 #define ip6_addr_isduplicated(addr_state) (addr_state == IP6_ADDR_DUPLICATED) 00251 00252 #if LWIP_IPV6_ADDRESS_LIFETIMES 00253 #define IP6_ADDR_LIFE_STATIC (0) 00254 #define IP6_ADDR_LIFE_INFINITE (0xffffffffUL) 00255 #define ip6_addr_life_isstatic(addr_life) ((addr_life) == IP6_ADDR_LIFE_STATIC) 00256 #define ip6_addr_life_isinfinite(addr_life) ((addr_life) == IP6_ADDR_LIFE_INFINITE) 00257 #endif /* LWIP_IPV6_ADDRESS_LIFETIMES */ 00258 00259 #define ip6_addr_debug_print_parts(debug, a, b, c, d, e, f, g, h) \ 00260 LWIP_DEBUGF(debug, ("%" X16_F ":%" X16_F ":%" X16_F ":%" X16_F ":%" X16_F ":%" X16_F ":%" X16_F ":%" X16_F, \ 00261 a, b, c, d, e, f, g, h)) 00262 #define ip6_addr_debug_print(debug, ipaddr) \ 00263 ip6_addr_debug_print_parts(debug, \ 00264 (u16_t)((ipaddr) != NULL ? IP6_ADDR_BLOCK1(ipaddr) : 0), \ 00265 (u16_t)((ipaddr) != NULL ? IP6_ADDR_BLOCK2(ipaddr) : 0), \ 00266 (u16_t)((ipaddr) != NULL ? IP6_ADDR_BLOCK3(ipaddr) : 0), \ 00267 (u16_t)((ipaddr) != NULL ? IP6_ADDR_BLOCK4(ipaddr) : 0), \ 00268 (u16_t)((ipaddr) != NULL ? IP6_ADDR_BLOCK5(ipaddr) : 0), \ 00269 (u16_t)((ipaddr) != NULL ? IP6_ADDR_BLOCK6(ipaddr) : 0), \ 00270 (u16_t)((ipaddr) != NULL ? IP6_ADDR_BLOCK7(ipaddr) : 0), \ 00271 (u16_t)((ipaddr) != NULL ? IP6_ADDR_BLOCK8(ipaddr) : 0)) 00272 #define ip6_addr_debug_print_val(debug, ipaddr) \ 00273 ip6_addr_debug_print_parts(debug, \ 00274 IP6_ADDR_BLOCK1(&(ipaddr)), \ 00275 IP6_ADDR_BLOCK2(&(ipaddr)), \ 00276 IP6_ADDR_BLOCK3(&(ipaddr)), \ 00277 IP6_ADDR_BLOCK4(&(ipaddr)), \ 00278 IP6_ADDR_BLOCK5(&(ipaddr)), \ 00279 IP6_ADDR_BLOCK6(&(ipaddr)), \ 00280 IP6_ADDR_BLOCK7(&(ipaddr)), \ 00281 IP6_ADDR_BLOCK8(&(ipaddr))) 00282 00283 #define IP6ADDR_STRLEN_MAX 46 00284 00285 int ip6addr_aton(const char *cp, ip6_addr_t *addr); 00286 /** returns ptr to static buffer; not reentrant! */ 00287 char *ip6addr_ntoa(const ip6_addr_t *addr); 00288 char *ip6addr_ntoa_r(const ip6_addr_t *addr, char *buf, int buflen); 00289 00290 00291 00292 #ifdef __cplusplus 00293 } 00294 #endif 00295 00296 #endif /* LWIP_IPV6 */ 00297 00298 #endif /* LWIP_HDR_IP6_ADDR_H */
Generated on Sun Jul 17 2022 08:25:23 by
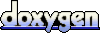