
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ioper_test_target_id.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2015 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 00017 Author: Przemyslaw Wirkus <Przemyslaw.Wirkus@arm.com> 00018 00019 """ 00020 00021 from ioper_base import IOperTestCaseBase 00022 00023 00024 class IOperTest_TargetID (IOperTestCaseBase): 00025 """ tests related to target_id value 00026 """ 00027 00028 def __init__(self, scope=None): 00029 IOperTestCaseBase.__init__(self, scope) 00030 self.TARGET_ID_LEN = 24 00031 00032 def test_target_id_format(self, target_id, target_id_name): 00033 # Expected length == 24, eg. "02400203D94B0E7724B7F3CF" 00034 result = [] 00035 target_id_len = len(target_id) if target_id else 0 00036 if target_id_len == self.TARGET_ID_LEN : 00037 result.append((self.PASS, "TARGET_ID_LEN", self.scope, "%s '%s' is %d chars long " % (target_id_name, target_id, target_id_len))) 00038 result.append((self.INFO, "FW_VER_STR", self.scope, "%s Version String is %s.%s.%s " % (target_id_name, 00039 target_id[0:4], 00040 target_id[4:8], 00041 target_id[8:24], 00042 ))) 00043 else: 00044 result.append((self.ERROR, "TARGET_ID_LEN", self.scope, "%s '%s' is %d chars long. Expected %d chars" % (target_id_name, target_id, target_id_len, self.TARGET_ID_LEN ))) 00045 return result 00046 00047 def test_decode_target_id(self, target_id, target_id_name): 00048 result = [] 00049 target_id_len = len(target_id) if target_id else 0 00050 if target_id_len >= 4: 00051 result.append((self.INFO, "FW_VEN_CODE", self.scope, "%s Vendor Code is '%s'" % (target_id_name, target_id[0:2]))) 00052 result.append((self.INFO, "FW_PLAT_CODE", self.scope, "%s Platform Code is '%s'" % (target_id_name, target_id[2:4]))) 00053 result.append((self.INFO, "FW_VER", self.scope, "%s Firmware Version is '%s'" % (target_id_name, target_id[4:8]))) 00054 result.append((self.INFO, "FW_HASH_SEC", self.scope, "%s Hash of secret is '%s'" % (target_id_name, target_id[8:24]))) 00055 return result 00056 00057 def test(self, param=None): 00058 result = [] 00059 if param: 00060 pass 00061 return result 00062 00063 00064 class IOperTest_TargetID_Basic (IOperTest_TargetID ): 00065 """ Basic interoperability tests checking TargetID compliance 00066 """ 00067 00068 def __init__(self, scope=None): 00069 IOperTest_TargetID.__init__(self, scope) 00070 00071 def test(self, param=None): 00072 result = [] 00073 00074 if param: 00075 result.append((self.PASS, "TARGET_ID", self.scope, "TargetID '%s' found" % param['target_id'])) 00076 00077 # Check if target name can be decoded with mbed-ls 00078 if param['platform_name']: 00079 result.append((self.PASS, "TARGET_ID_DECODE", self.scope, "TargetID '%s' decoded as '%s'" % (param['target_id'][0:4], param['platform_name']))) 00080 else: 00081 result.append((self.ERROR, "TARGET_ID_DECODE", self.scope, "TargetID '%s'... not decoded" % (param['target_id'] if param['target_id'] else ''))) 00082 00083 # Test for USBID and mbed.htm consistency 00084 if param['target_id_mbed_htm'] == param['target_id_usb_id']: 00085 result.append((self.PASS, "TARGET_ID_MATCH", self.scope, "TargetID (USBID) and TargetID (mbed.htm) match")) 00086 else: 00087 text = "TargetID (USBID) and TargetID (mbed.htm) don't match: '%s' != '%s'" % (param['target_id_usb_id'], param['target_id_mbed_htm']) 00088 result.append((self.WARN, "TARGET_ID_MATCH", self.scope, text)) 00089 else: 00090 result.append((self.ERROR, "TARGET_ID", self.scope, "TargetID not found")) 00091 return result 00092 00093 class IOperTest_TargetID_MbedEnabled (IOperTest_TargetID ): 00094 """ Basic interoperability tests checking TargetID compliance 00095 """ 00096 00097 def __init__(self, scope=None): 00098 IOperTest_TargetID.__init__(self, scope) 00099 00100 def test(self, param=None): 00101 result = [] 00102 00103 if param: 00104 # Target ID tests: 00105 result += self.test_target_id_format(param['target_id_usb_id'], "TargetId (USBID)") 00106 result += self.test_target_id_format(param['target_id_mbed_htm'], "TargetId (mbed.htm)") 00107 00108 # Some extra info about TargetID itself 00109 result += self.test_decode_target_id(param['target_id_usb_id'], "TargetId (USBID)") 00110 result += self.test_decode_target_id(param['target_id_mbed_htm'], "TargetId (mbed.htm)") 00111 return result
Generated on Sun Jul 17 2022 08:25:23 by
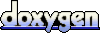