
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ioper_runner.py
00001 #!/usr/bin/env python2 00002 """ 00003 mbed SDK 00004 Copyright (c) 2011-2015 ARM Limited 00005 00006 Licensed under the Apache License, Version 2.0 (the "License"); 00007 you may not use this file except in compliance with the License. 00008 You may obtain a copy of the License at 00009 00010 http://www.apache.org/licenses/LICENSE-2.0 00011 00012 Unless required by applicable law or agreed to in writing, software 00013 distributed under the License is distributed on an "AS IS" BASIS, 00014 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 See the License for the specific language governing permissions and 00016 limitations under the License. 00017 00018 Author: Przemyslaw Wirkus <Przemyslaw.Wirkus@arm.com> 00019 00020 """ 00021 00022 import sys 00023 import mbed_lstools 00024 from prettytable import PrettyTable 00025 00026 try: 00027 from colorama import init 00028 except: 00029 pass 00030 00031 COLORAMA = 'colorama' in sys.modules 00032 00033 from ioper_base import IOperTestCaseBase 00034 from ioper_test_fs import IOperTest_FileStructure_Basic 00035 from ioper_test_fs import IOperTest_FileStructure_MbedEnabled 00036 from ioper_test_target_id import IOperTest_TargetID_Basic 00037 from ioper_test_target_id import IOperTest_TargetID_MbedEnabled 00038 00039 00040 TEST_LIST = [IOperTest_TargetID_Basic('basic'), 00041 IOperTest_TargetID_MbedEnabled('mbed-enabled'), 00042 IOperTest_FileStructure_Basic('basic'), 00043 IOperTest_FileStructure_MbedEnabled('mbed-enabled'), 00044 IOperTestCaseBase('all'), # Dummy used to add 'all' option 00045 ] 00046 00047 00048 class IOperTestRunner (): 00049 """ Calls all i/face interoperability tests 00050 """ 00051 00052 def __init__ (self, scope=None): 00053 """ Test scope: 00054 'pedantic' - all 00055 'mbed-enabled' - let's try to check if this device is mbed-enabled 00056 'basic' - just simple, passive tests (no device flashing) 00057 """ 00058 self.requested_scope = scope # Test scope given by user 00059 self.raw_test_results = {} # Raw test results, can be used by exporters: { Platform: [test results]} 00060 00061 # Test scope definitions 00062 self.SCOPE_BASIC = 'basic' # Basic tests, sanity checks 00063 self.SCOPE_MBED_ENABLED = 'mbed-enabled' # Let's try to check if this device is mbed-enabled 00064 self.SCOPE_PEDANTIC = 'pedantic' # Extensive tests 00065 self.SCOPE_ALL = 'all' # All tests, equal to highest scope level 00066 00067 # This structure will help us sort test scopes so we can include them 00068 # e.g. pedantic also includes basic and mbed-enabled tests 00069 self.scopes = {self.SCOPE_BASIC : 0, 00070 self.SCOPE_MBED_ENABLED : 1, 00071 self.SCOPE_PEDANTIC : 2, 00072 self.SCOPE_ALL : 99, 00073 } 00074 00075 if COLORAMA: 00076 init() # colorama.init() 00077 00078 def run (self): 00079 """ Run tests, calculate overall score and print test results 00080 """ 00081 mbeds = mbed_lstools.create() 00082 muts_list = mbeds.list_mbeds() 00083 test_base = IOperTestCaseBase() 00084 00085 self.raw_test_results = {} 00086 for i, mut in enumerate(muts_list): 00087 result = [] 00088 self.raw_test_results [mut['platform_name']] = [] 00089 00090 print "MBEDLS: Detected %s, port: %s, mounted: %s"% (mut['platform_name'], 00091 mut['serial_port'], 00092 mut['mount_point']) 00093 print "Running interoperability test suite, scope '%s'" % (self.requested_scope ) 00094 for test_case in TEST_LIST: 00095 if self.scopes [self.requested_scope ] >= self.scopes [test_case.scope]: 00096 res = test_case.test(param=mut) 00097 result.extend(res) 00098 self.raw_test_results [mut['platform_name']].extend(res) 00099 00100 columns = ['Platform', 'Test Case', 'Result', 'Scope', 'Description'] 00101 pt = PrettyTable(columns) 00102 for col in columns: 00103 pt.align[col] = 'l' 00104 00105 for tr in result: 00106 severity, tr_name, tr_scope, text = tr 00107 tr = (test_base.COLOR(severity, mut['platform_name']), 00108 test_base.COLOR(severity, tr_name), 00109 test_base.COLOR(severity, severity), 00110 test_base.COLOR(severity, tr_scope), 00111 test_base.COLOR(severity, text)) 00112 pt.add_row(list(tr)) 00113 print pt.get_string(border=True, sortby='Result') 00114 if i + 1 < len(muts_list): 00115 print 00116 return self.raw_test_results 00117 00118 def get_available_oper_test_scopes(): 00119 """ Get list of available test scopes 00120 """ 00121 scopes = set() 00122 for oper_test in TEST_LIST: 00123 if oper_test.scope is not None: 00124 scopes.add(oper_test.scope) 00125 return list(scopes)
Generated on Sun Jul 17 2022 08:25:23 by
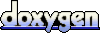