
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
iBeacon.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_BLE_IBEACON_H__ 00017 #define MBED_BLE_IBEACON_H__ 00018 00019 #include "cmsis_compiler.h" 00020 #include "ble/BLE.h" 00021 00022 /** 00023 * iBeacon Service. 00024 * 00025 * @par Purpose 00026 * 00027 * iBeacons are Bluetooth Low Energy (BLE) devices advertising an identification 00028 * number generally used to determine the location of devices or physical objects 00029 * near a mobile phone user. 00030 * 00031 * iOS scans for iBeacon devices in a background task and notifies Apps 00032 * subscribed to a specific region when the area is entered or left. Apps may 00033 * use this information to display context-aware content to users. 00034 * 00035 * As an example, a museum can deploy an app that informs the user when one of 00036 * its exhibitions is entered and then displays specific information about exposed 00037 * pieces of art when the user is sufficiently close to them. 00038 * 00039 * @par Positioning 00040 * 00041 * Location information is hierarchically structured. A UUID specific to the 00042 * application and its deployment is used to identify a region. That region 00043 * usually identifies an organization. The region is divided into subregions 00044 * identified by a major ID. The subregion contains related points of interest 00045 * which a minor ID distinguishes. 00046 * 00047 * As an example, a city willing to improve tourist's experience can deploy a fleet 00048 * of iBeacons in relevant touristic locations it operates. The UUID may 00049 * identify a place managed by the city. The major ID would identify the place; 00050 * it can be a museum, a historic monument, a metro station and so on. The minor ID 00051 * would locate a specific spot within a specific city place. It can be a 00052 * piece of art, a ticket dispenser or a relevant point of interest. 00053 * 00054 * Each iBeacon device is physically attached to the spot it locates and 00055 * advertises the triplet UUID, major ID and minor ID. 00056 * 00057 * @par Proximity 00058 * 00059 * The beacon advertises the signal strength measured by an iOS device at a 00060 * distance of one meter. iOS uses this information to approximate the 00061 * proximity to a given beacon: 00062 * - Immediate: The beacon is less than one meter away from the user. 00063 * - Near: The beacon is one to three meters away from the user. 00064 * - Far: The user is not near the beacon; the distance highly depends on 00065 * the physical environment. 00066 * 00067 * Ideally, beacons should be calibrated at their deployment location because the 00068 * surrounding environment affects the strength of the advertised signal. 00069 * 00070 * @par Usage 00071 * 00072 * Mbed OS applications can use this class to configure a device to broadcast 00073 * advertising packets mimicking an iBeacon. The construction automatically 00074 * creates the payload identifying the beacon and registers it as part of the 00075 * advertising payload of the device. 00076 * 00077 * Beacon configuration and advertising commencement is left to the user. 00078 * 00079 * @important If you are interested in manufacturing iBeacons, you must obtain a 00080 * license from Apple. More information at https://developer.apple.com/ibeacon/. 00081 * The licence also grant access to the iBeacons technical specification. 00082 * 00083 * @note More information at https://developer.apple.com/ibeacon/Getting-Started-with-iBeacon.pdf 00084 */ 00085 class iBeacon 00086 { 00087 public: 00088 /** 00089 * Data buffer of a location UUID. 00090 */ 00091 typedef const uint8_t LocationUUID_t[16]; 00092 00093 /** 00094 * iBeacon payload builder. 00095 * 00096 * This data structure contains the payload of an iBeacon. The payload is 00097 * built at construction time and application code can set up an iBeacon by 00098 * injecting the raw field into the GAP advertising payload as a 00099 * GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA. 00100 */ 00101 union Payload { 00102 /** 00103 * Raw data of the payload. 00104 */ 00105 uint8_t raw[25]; 00106 struct { 00107 /** 00108 * Beacon manufacturer identifier. 00109 */ 00110 uint16_t companyID; 00111 00112 /** 00113 * Packet ID; Equal to 2 for an iBeacon. 00114 */ 00115 uint8_t ID; 00116 00117 /** 00118 * Length of the remaining data presents in the payload. 00119 */ 00120 uint8_t len; 00121 00122 /** 00123 * Beacon UUID. 00124 */ 00125 uint8_t proximityUUID[16]; 00126 00127 /** 00128 * Beacon Major group ID. 00129 */ 00130 uint16_t majorNumber; 00131 00132 /** 00133 * Beacon minor ID. 00134 */ 00135 uint16_t minorNumber; 00136 00137 /** 00138 * Tx power received at 1 meter; in dBm. 00139 */ 00140 uint8_t txPower; 00141 }; 00142 00143 /** 00144 * Assemble an iBeacon payload. 00145 * 00146 * @param[in] uuid Beacon network ID. iBeacon operators use this value 00147 * to group their iBeacons into a single network, a single region and 00148 * identify their organization among others. 00149 * 00150 * @param[in] majNum Beacon major group ID. iBeacon exploitants may use 00151 * this field to divide the region into subregions, their network into 00152 * subnetworks. 00153 * 00154 * @param[in] minNum Identifier of the Beacon in its subregion. 00155 * 00156 * @param[in] transmitPower Measured transmit power of the beacon at 1 00157 * meter. Scanners use this parameter to approximate the distance 00158 * to the beacon. 00159 * 00160 * @param[in] companyIDIn ID of the beacon manufacturer. 00161 */ 00162 Payload( 00163 LocationUUID_t uuid, 00164 uint16_t majNum, 00165 uint16_t minNum, 00166 uint8_t transmitPower, 00167 uint16_t companyIDIn 00168 ) : companyID(companyIDIn), 00169 ID(0x02), 00170 len(0x15), 00171 majorNumber(__REV16(majNum)), 00172 minorNumber(__REV16(minNum)), 00173 txPower(transmitPower) 00174 { 00175 memcpy(proximityUUID, uuid, sizeof(LocationUUID_t)); 00176 } 00177 }; 00178 00179 public: 00180 /** 00181 * Construct an iBeacon::Payload and register it into Gap. 00182 * 00183 * @param[in] _ble The BLE interface to configure with the iBeacon payload. 00184 * 00185 * @param[in] uuid Beacon network ID. iBeacon operators use this value 00186 * to group their iBeacons into a single network, a single region and 00187 * identify their organization among others. 00188 * 00189 * @param[in] majNum Beacon major group ID. iBeacon exploitants may use 00190 * this field to divide the region into subregions, their network into 00191 * subnetworks. 00192 * 00193 * @param[in] minNum Identifier of the Beacon in its subregion. 00194 * 00195 * @param[in] txP Measured transmit power of the beacon at 1 00196 * meter. Scanners use this parameter to approximate the distance 00197 * to the beacon. 00198 * 00199 * @param[in] compID ID of the beacon manufacturer. 00200 */ 00201 iBeacon( 00202 BLE &_ble, 00203 LocationUUID_t uuid, 00204 uint16_t majNum, 00205 uint16_t minNum, 00206 uint8_t txP = 0xC8, 00207 uint16_t compID = 0x004C 00208 ) : ble(_ble), 00209 data(uuid, majNum, minNum, txP, compID) 00210 { 00211 // Generate the 0x020106 part of the iBeacon Prefix. 00212 ble.accumulateAdvertisingPayload( 00213 GapAdvertisingData::BREDR_NOT_SUPPORTED | 00214 GapAdvertisingData::LE_GENERAL_DISCOVERABLE 00215 ); 00216 // Generate the 0x1AFF part of the iBeacon Prefix. 00217 ble.accumulateAdvertisingPayload( 00218 GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA, 00219 data.raw, 00220 sizeof(data.raw) 00221 ); 00222 00223 // Set advertising type. 00224 ble.setAdvertisingType( 00225 GapAdvertisingParams::ADV_NON_CONNECTABLE_UNDIRECTED 00226 ); 00227 } 00228 00229 protected: 00230 BLE &ble; 00231 Payload data; 00232 }; 00233 00234 /** 00235 * iBeacon alias. 00236 * 00237 * @deprecated Please use iBeacon directly. This alias may be dropped from a 00238 * future release. 00239 */ 00240 typedef iBeacon iBeaconService; 00241 00242 #endif //MBED_BLE_IBEACON_H__
Generated on Sun Jul 17 2022 08:25:23 by
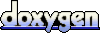