
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
halt_exports.h
00001 /* 00002 * Copyright (c) 2015, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifndef __UVISOR_API_HALT_EXPORTS_H__ 00018 #define __UVISOR_API_HALT_EXPORTS_H__ 00019 00020 #include "uvisor_exports.h" 00021 00022 #define UVISOR_ERROR_INVALID_BOX_ID (-2) 00023 #define UVISOR_ERROR_BUFFER_TOO_SMALL (-3) 00024 #define UVISOR_ERROR_BOX_NAMESPACE_ANONYMOUS (-4) 00025 #define UVISOR_ERROR_BAD_MAGIC (-5) 00026 #define UVISOR_ERROR_BAD_VERSION (-6) 00027 #define UVISOR_ERROR_OUT_OF_STRUCTURES (-7) 00028 #define UVISOR_ERROR_INVALID_PARAMETERS (-8) 00029 #define UVISOR_ERROR_NOT_IMPLEMENTED (-9) 00030 #define UVISOR_ERROR_TIMEOUT (-10) 00031 00032 00033 #define UVISOR_ERROR_CLASS_MASK (0xFFFF0000UL) 00034 #define UVISOR_ERROR_MASK (0x0000FFFFUL) 00035 00036 #define UVISOR_ERROR_CLASS_PAGE (1UL << 16) 00037 00038 typedef enum { 00039 USER_NOT_ALLOWED = 1, 00040 DEBUG_BOX_HALT, 00041 } THaltUserError; 00042 00043 typedef enum { 00044 HALT_NO_ERROR = 0, 00045 PERMISSION_DENIED = 1, 00046 SANITY_CHECK_FAILED, 00047 NOT_IMPLEMENTED, 00048 NOT_ALLOWED, 00049 FAULT_MEMMANAGE, 00050 FAULT_BUS, 00051 FAULT_USAGE, 00052 FAULT_HARD, 00053 FAULT_DEBUG, 00054 FAULT_SECURE, 00055 __THALTERROR_MAX /* always keep as the last element of the enum */ 00056 } THaltError; 00057 00058 /** A basic exception frame 00059 * 00060 * This struct contains the registers always saved during an exception in the 00061 * order they are placed in the memory. 00062 * If FPU state is also saved it's placed after this register block. 00063 * On ARMv8-M in certain cases an additional state context may be placed on 00064 * the stack before this block. 00065 */ 00066 typedef struct { 00067 uint32_t r0; 00068 uint32_t r1; 00069 uint32_t r2; 00070 uint32_t r3; 00071 uint32_t r12; 00072 uint32_t lr; 00073 uint32_t retaddr; 00074 uint32_t retpsr; 00075 } UVISOR_PACKED exception_frame_t; 00076 00077 /* A pointer to this structure will be given to halt_error() handler 00078 * of the debug box driver. */ 00079 typedef struct { 00080 /* A basic exception stack frame that is always present with a valid stack. */ 00081 exception_frame_t stack_frame; 00082 00083 /* A few registers that may be useful for debug. */ 00084 uint32_t lr; 00085 uint32_t control; 00086 uint32_t ipsr; 00087 00088 /* Fault status registers. */ 00089 uint32_t mmfar; 00090 uint32_t bfar; 00091 uint32_t cfsr; 00092 uint32_t hfsr; 00093 uint32_t dfsr; 00094 uint32_t afsr; 00095 00096 /* Bitmask telling which of the above regions are valid. */ 00097 uint32_t valid_data; 00098 } UVISOR_PACKED THaltInfo; 00099 00100 /* Bitmask to specify which HaltInfo regions are valid. */ 00101 typedef enum { 00102 HALT_INFO_STACK_FRAME = 0x1, 00103 HALT_INFO_REGISTERS = 0x2 00104 } THaltInfoValidMask; 00105 00106 #endif /* __UVISOR_API_HALT_EXPORTS_H__ */
Generated on Sun Jul 17 2022 08:25:23 by
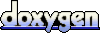