
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
get_interface.cpp
00001 /* 00002 * Copyright (c) 2017, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #include "mbed.h" 00019 00020 // Pick the correct driver based on mbed_app.json 00021 #define INTERNAL 1 00022 #define WIFI_ESP8266 2 00023 #define X_NUCLEO_IDW01M1 3 00024 00025 #if MBED_CONF_APP_WIFI_DRIVER == INTERNAL 00026 00027 #if TARGET_UBLOX_EVK_ODIN_W2 00028 #include "OdinWiFiInterface.h" 00029 #define DRIVER OdinWiFiInterface 00030 00031 #elif TARGET_REALTEK_RTL8195AM 00032 #include "RTWInterface.h" 00033 #define DRIVER RTWInterface 00034 #else 00035 #error [NOT_SUPPORTED] Unsupported Wifi driver 00036 #endif 00037 00038 #elif MBED_CONF_APP_WIFI_DRIVER == WIFI_ESP8266 00039 #include "ESP8266Interface.h" 00040 #define DRIVER ESP8266Interface 00041 00042 #elif MBED_CONF_APP_WIFI_DRIVER == X_NUCLEO_IDW01M1 00043 #include "SpwfSAInterface.h" 00044 #define DRIVER SpwfSAInterface 00045 #else 00046 #error [NOT_SUPPORTED] Unsupported Wifi driver 00047 #endif 00048 00049 WiFiInterface *get_interface() 00050 { 00051 static WiFiInterface *interface = NULL; 00052 00053 if (interface) 00054 delete interface; 00055 00056 #if MBED_CONF_APP_WIFI_DRIVER == INTERNAL 00057 interface = new DRIVER(); 00058 #else 00059 interface = new DRIVER(MBED_CONF_APP_WIFI_TX, MBED_CONF_APP_WIFI_RX); 00060 #endif 00061 return interface; 00062 }
Generated on Sun Jul 17 2022 08:25:23 by
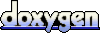