
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
gcm.h
Go to the documentation of this file.
00001 /** 00002 * \file gcm.h 00003 * 00004 * \brief Galois/Counter mode for 128-bit block ciphers 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_GCM_H 00024 #define MBEDTLS_GCM_H 00025 00026 #include "cipher.h" 00027 00028 #include <stdint.h> 00029 00030 #define MBEDTLS_GCM_ENCRYPT 1 00031 #define MBEDTLS_GCM_DECRYPT 0 00032 00033 #define MBEDTLS_ERR_GCM_AUTH_FAILED -0x0012 /**< Authenticated decryption failed. */ 00034 #define MBEDTLS_ERR_GCM_BAD_INPUT -0x0014 /**< Bad input parameters to function. */ 00035 00036 #ifdef __cplusplus 00037 extern "C" { 00038 #endif 00039 00040 /** 00041 * \brief GCM context structure 00042 */ 00043 typedef struct { 00044 mbedtls_cipher_context_t cipher_ctx ;/*!< cipher context used */ 00045 uint64_t HL[16]; /*!< Precalculated HTable */ 00046 uint64_t HH[16]; /*!< Precalculated HTable */ 00047 uint64_t len ; /*!< Total data length */ 00048 uint64_t add_len ; /*!< Total add length */ 00049 unsigned char base_ectr[16];/*!< First ECTR for tag */ 00050 unsigned char y[16]; /*!< Y working value */ 00051 unsigned char buf[16]; /*!< buf working value */ 00052 int mode ; /*!< Encrypt or Decrypt */ 00053 } 00054 mbedtls_gcm_context; 00055 00056 /** 00057 * \brief Initialize GCM context (just makes references valid) 00058 * Makes the context ready for mbedtls_gcm_setkey() or 00059 * mbedtls_gcm_free(). 00060 * 00061 * \param ctx GCM context to initialize 00062 */ 00063 void mbedtls_gcm_init( mbedtls_gcm_context *ctx ); 00064 00065 /** 00066 * \brief GCM initialization (encryption) 00067 * 00068 * \param ctx GCM context to be initialized 00069 * \param cipher cipher to use (a 128-bit block cipher) 00070 * \param key encryption key 00071 * \param keybits must be 128, 192 or 256 00072 * 00073 * \return 0 if successful, or a cipher specific error code 00074 */ 00075 int mbedtls_gcm_setkey( mbedtls_gcm_context *ctx, 00076 mbedtls_cipher_id_t cipher, 00077 const unsigned char *key, 00078 unsigned int keybits ); 00079 00080 /** 00081 * \brief GCM buffer encryption/decryption using a block cipher 00082 * 00083 * \note On encryption, the output buffer can be the same as the input buffer. 00084 * On decryption, the output buffer cannot be the same as input buffer. 00085 * If buffers overlap, the output buffer must trail at least 8 bytes 00086 * behind the input buffer. 00087 * 00088 * \param ctx GCM context 00089 * \param mode MBEDTLS_GCM_ENCRYPT or MBEDTLS_GCM_DECRYPT 00090 * \param length length of the input data 00091 * \param iv initialization vector 00092 * \param iv_len length of IV 00093 * \param add additional data 00094 * \param add_len length of additional data 00095 * \param input buffer holding the input data 00096 * \param output buffer for holding the output data 00097 * \param tag_len length of the tag to generate 00098 * \param tag buffer for holding the tag 00099 * 00100 * \return 0 if successful 00101 */ 00102 int mbedtls_gcm_crypt_and_tag( mbedtls_gcm_context *ctx, 00103 int mode, 00104 size_t length, 00105 const unsigned char *iv, 00106 size_t iv_len, 00107 const unsigned char *add, 00108 size_t add_len, 00109 const unsigned char *input, 00110 unsigned char *output, 00111 size_t tag_len, 00112 unsigned char *tag ); 00113 00114 /** 00115 * \brief GCM buffer authenticated decryption using a block cipher 00116 * 00117 * \note On decryption, the output buffer cannot be the same as input buffer. 00118 * If buffers overlap, the output buffer must trail at least 8 bytes 00119 * behind the input buffer. 00120 * 00121 * \param ctx GCM context 00122 * \param length length of the input data 00123 * \param iv initialization vector 00124 * \param iv_len length of IV 00125 * \param add additional data 00126 * \param add_len length of additional data 00127 * \param tag buffer holding the tag 00128 * \param tag_len length of the tag 00129 * \param input buffer holding the input data 00130 * \param output buffer for holding the output data 00131 * 00132 * \return 0 if successful and authenticated, 00133 * MBEDTLS_ERR_GCM_AUTH_FAILED if tag does not match 00134 */ 00135 int mbedtls_gcm_auth_decrypt( mbedtls_gcm_context *ctx, 00136 size_t length, 00137 const unsigned char *iv, 00138 size_t iv_len, 00139 const unsigned char *add, 00140 size_t add_len, 00141 const unsigned char *tag, 00142 size_t tag_len, 00143 const unsigned char *input, 00144 unsigned char *output ); 00145 00146 /** 00147 * \brief Generic GCM stream start function 00148 * 00149 * \param ctx GCM context 00150 * \param mode MBEDTLS_GCM_ENCRYPT or MBEDTLS_GCM_DECRYPT 00151 * \param iv initialization vector 00152 * \param iv_len length of IV 00153 * \param add additional data (or NULL if length is 0) 00154 * \param add_len length of additional data 00155 * 00156 * \return 0 if successful 00157 */ 00158 int mbedtls_gcm_starts( mbedtls_gcm_context *ctx, 00159 int mode, 00160 const unsigned char *iv, 00161 size_t iv_len, 00162 const unsigned char *add, 00163 size_t add_len ); 00164 00165 /** 00166 * \brief Generic GCM update function. Encrypts/decrypts using the 00167 * given GCM context. Expects input to be a multiple of 16 00168 * bytes! Only the last call before mbedtls_gcm_finish() can be less 00169 * than 16 bytes! 00170 * 00171 * \note On decryption, the output buffer cannot be the same as input buffer. 00172 * If buffers overlap, the output buffer must trail at least 8 bytes 00173 * behind the input buffer. 00174 * 00175 * \param ctx GCM context 00176 * \param length length of the input data 00177 * \param input buffer holding the input data 00178 * \param output buffer for holding the output data 00179 * 00180 * \return 0 if successful or MBEDTLS_ERR_GCM_BAD_INPUT 00181 */ 00182 int mbedtls_gcm_update( mbedtls_gcm_context *ctx, 00183 size_t length, 00184 const unsigned char *input, 00185 unsigned char *output ); 00186 00187 /** 00188 * \brief Generic GCM finalisation function. Wraps up the GCM stream 00189 * and generates the tag. The tag can have a maximum length of 00190 * 16 bytes. 00191 * 00192 * \param ctx GCM context 00193 * \param tag buffer for holding the tag 00194 * \param tag_len length of the tag to generate (must be at least 4) 00195 * 00196 * \return 0 if successful or MBEDTLS_ERR_GCM_BAD_INPUT 00197 */ 00198 int mbedtls_gcm_finish( mbedtls_gcm_context *ctx, 00199 unsigned char *tag, 00200 size_t tag_len ); 00201 00202 /** 00203 * \brief Free a GCM context and underlying cipher sub-context 00204 * 00205 * \param ctx GCM context to free 00206 */ 00207 void mbedtls_gcm_free( mbedtls_gcm_context *ctx ); 00208 00209 /** 00210 * \brief Checkup routine 00211 * 00212 * \return 0 if successful, or 1 if the test failed 00213 */ 00214 int mbedtls_gcm_self_test( int verbose ); 00215 00216 #ifdef __cplusplus 00217 } 00218 #endif 00219 00220 #endif /* gcm.h */
Generated on Sun Jul 17 2022 08:25:23 by
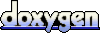