
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
flash_journal_strategy_sequential.h
00001 /* 00002 * Copyright (c) 2006-2016, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #ifndef __FLASH_JOURNAL_STRATEGY_SEQUENTIAL_H__ 00019 #define __FLASH_JOURNAL_STRATEGY_SEQUENTIAL_H__ 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif // __cplusplus 00024 00025 #include "flash-journal/flash_journal.h" 00026 00027 /** 00028 * Create/format a sequential flash journal at a given offset within a storage 00029 * device and with a given slot-cardinality. 00030 * 00031 * This function must be called *once* for each incarnation of a sequential 00032 * journal. 00033 * 00034 * @param[in] mtd 00035 * The underlying Storage driver. 00036 * 00037 * @param[in] numSlots 00038 * Number of slots in the sequential journal. Each slot holds a header, blob-payload, and a tail. 00039 * 00040 * @param[in] callback 00041 * Caller-defined callback to be invoked upon command completion 00042 * in case the storage device executes operations asynchronously. 00043 * Use a NULL pointer when no callback signals are required. 00044 * 00045 * @note: this is an asynchronous operation, but it can finish 00046 * synchronously if the underlying MTD supports that. 00047 * 00048 * @return 00049 * The function executes in the following ways: 00050 * - When the operation is asynchronous, the function only starts the 00051 * initialization and control returns to the caller with an 00052 * JOURNAL_STATUS_OK before the actual completion of the operation (or with 00053 * an appropriate error code in case of failure). When the operation is 00054 * completed the command callback is invoked with 1 passed in as the 00055 * 'status' parameter of the callback. In case of errors, the completion 00056 * callback is invoked with an error status. 00057 * - When the operation is executed by the journal in a blocking (i.e. 00058 * synchronous) manner, control returns to the caller only upon the actual 00059 * completion of the operation or the discovery of a failure condition. In 00060 * this case, the function returns 1 to signal successful synchronous 00061 * completion or an appropriate error code, and no further 00062 * invocation of the completion callback should be expected at a later time. 00063 * 00064 * Here's a code snippet to suggest how this API might be used by callers: 00065 * \code 00066 * ASSERT(JOURNAL_STATUS_OK == 0); // this is a precondition; it doesn't need to be put in code 00067 * int32_t returnValue = flashJournalStrategySequential_format(MTD, numSlots, callbackHandler); 00068 * if (returnValue < JOURNAL_STATUS_OK) { 00069 * // handle error 00070 * } else if (returnValue == JOURNAL_STATUS_OK) { 00071 * ASSERT(MTD->GetCapabilities().asynchronous_ops == 1); 00072 * // handle early return from asynchronous execution 00073 * } else { 00074 * ASSERT(returnValue == 1); 00075 * // handle synchronous completion 00076 * } 00077 * \endcode 00078 * 00079 * +-------------------------------+ ^ 00080 * | | | 00081 * | Journal Header | | 00082 * | starts with generic header | | 00083 * | followed by specific header | | 00084 * | | | multiple of program_unit 00085 * +-------------------------------+ | and erase-boundary 00086 * +-------------------------------+ | 00087 * | | | 00088 * | padding to allow alignment | | 00089 * | | | 00090 * +-------------------------------+ v 00091 * +-------------------------------+ 00092 * | +---------------------------+ | ^ 00093 * | | slot header | | | 00094 * | | aligned with program_unit| | | 00095 * | +---------------------------+ | | slot 0 00096 * | | | aligned with LCM of all erase boundaries 00097 * | | | 00098 * | | | 00099 * | | | 00100 * | BLOB0 | | 00101 * | | | 00102 * | | | 00103 * | +---------------------------+ | | 00104 * | | slot tail | | | 00105 * | | aligned with program_unit| | | 00106 * | +---------------------------+ | | 00107 * +-------------------------------+ v 00108 * +-------------------------------+ 00109 * | +---------------------------+ | ^ 00110 * | | slot header | | | 00111 * | | aligned with program_unit| | | 00112 * | +---------------------------+ | | slot 1 00113 * | | | aligned with LCM of all erase boundaries 00114 * | BLOB1 | | 00115 * | | | 00116 * . . . 00117 * . . . 00118 * 00119 * . . . 00120 * . BLOB(N-1) . . 00121 * | | | 00122 * | +---------------------------+ | | slot 'N - 1' 00123 * | | slot tail | | | aligned with LCM of all erase boundaries 00124 * | | aligned with program_unit| | | 00125 * | +---------------------------+ | | 00126 * +-------------------------------+ v 00127 */ 00128 int32_t flashJournalStrategySequential_format(ARM_DRIVER_STORAGE *mtd, 00129 uint32_t numSlots, 00130 FlashJournal_Callback_t callback); 00131 00132 int32_t flashJournalStrategySequential_initialize(FlashJournal_t *journal, 00133 ARM_DRIVER_STORAGE *mtd, 00134 const FlashJournal_Ops_t *ops, 00135 FlashJournal_Callback_t callback); 00136 FlashJournal_Status_t flashJournalStrategySequential_getInfo(FlashJournal_t *journal, FlashJournal_Info_t *info); 00137 int32_t flashJournalStrategySequential_read(FlashJournal_t *journal, void *blob, size_t n); 00138 int32_t flashJournalStrategySequential_readFrom(FlashJournal_t *journal, size_t offset, void *blob, size_t n); 00139 int32_t flashJournalStrategySequential_log(FlashJournal_t *journal, const void *blob, size_t n); 00140 int32_t flashJournalStrategySequential_commit(FlashJournal_t *journal); 00141 int32_t flashJournalStrategySequential_reset(FlashJournal_t *journal); 00142 00143 static const FlashJournal_Ops_t FLASH_JOURNAL_STRATEGY_SEQUENTIAL = { 00144 flashJournalStrategySequential_initialize, 00145 flashJournalStrategySequential_getInfo, 00146 flashJournalStrategySequential_read, 00147 flashJournalStrategySequential_readFrom, 00148 flashJournalStrategySequential_log, 00149 flashJournalStrategySequential_commit, 00150 flashJournalStrategySequential_reset 00151 }; 00152 00153 #ifdef __cplusplus 00154 } 00155 #endif // __cplusplus 00156 00157 #endif /* __FLASH_JOURNAL_STRATEGY_SEQUENTIAL_H__ */
Generated on Sun Jul 17 2022 08:25:23 by
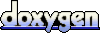