
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
extract.py
00001 #!/usr/bin/env python 00002 """ 00003 mbed 00004 Copyright (c) 2017-2017 ARM Limited 00005 00006 Licensed under the Apache License, Version 2.0 (the "License"); 00007 you may not use this file except in compliance with the License. 00008 You may obtain a copy of the License at 00009 00010 http://www.apache.org/licenses/LICENSE-2.0 00011 00012 Unless required by applicable law or agreed to in writing, software 00013 distributed under the License is distributed on an "AS IS" BASIS, 00014 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 See the License for the specific language governing permissions and 00016 limitations under the License. 00017 """ 00018 00019 from __future__ import print_function 00020 import sys 00021 import os 00022 import argparse 00023 from os.path import join, abspath, dirname 00024 from flash_algo import PackFlashAlgo 00025 00026 # Be sure that the tools directory is in the search path 00027 ROOT = abspath(join(dirname(__file__), "..", "..")) 00028 sys.path.insert(0, ROOT) 00029 00030 from tools.targets import TARGETS 00031 from tools.arm_pack_manager import Cache 00032 00033 TEMPLATE_PATH = "c_blob_mbed.tmpl" 00034 00035 00036 def main(): 00037 """Generate flash algorithms""" 00038 parser = argparse.ArgumentParser(description='Flash generator') 00039 parser.add_argument("--rebuild_all", action="store_true", 00040 help="Rebuild entire cache") 00041 parser.add_argument("--rebuild_descriptors", action="store_true", 00042 help="Rebuild descriptors") 00043 parser.add_argument("--target", default=None, 00044 help="Name of target to generate algo for") 00045 parser.add_argument("--all", action="store_true", 00046 help="Build all flash algos for devcies") 00047 args = parser.parse_args() 00048 00049 cache = Cache(True, True) 00050 if args.rebuild_all: 00051 cache.cache_everything() 00052 print("Cache rebuilt") 00053 return 00054 00055 if args.rebuild_descriptors: 00056 cache.cache_descriptors() 00057 print("Descriptors rebuilt") 00058 return 00059 00060 if args.target is None: 00061 device_and_filenames = [(target.device_name, target.name) for target 00062 in TARGETS if hasattr(target, "device_name")] 00063 else: 00064 device_and_filenames = [(args.target, args.target.replace("/", "-"))] 00065 00066 try: 00067 os.mkdir("output") 00068 except OSError: 00069 # Directory already exists 00070 pass 00071 00072 for device, filename in device_and_filenames: 00073 dev = cache.index[device] 00074 binaries = cache.get_flash_algorthim_binary(device, all=True) 00075 algos = [PackFlashAlgo(binary.read()) for binary in binaries] 00076 filtered_algos = algos if args.all else filter_algos(dev, algos) 00077 for idx, algo in enumerate(filtered_algos): 00078 file_name = ("%s_%i.c" % (filename, idx) 00079 if args.all or len(filtered_algos) != 1 00080 else "%s.c" % filename) 00081 output_path = join("output", file_name) 00082 algo.process_template(TEMPLATE_PATH, output_path) 00083 print("%s: %s \r" % (device, filename)) 00084 00085 00086 def filter_algos(dev, algos): 00087 if "memory" not in dev: 00088 return algos 00089 if "IROM1" not in dev["memory"]: 00090 return algos 00091 if "IROM2" in dev["memory"]: 00092 return algos 00093 00094 rom_rgn = dev["memory"]["IROM1"] 00095 try: 00096 start = int(rom_rgn["start"], 0) 00097 size = int(rom_rgn["size"], 0) 00098 except ValueError: 00099 return algos 00100 00101 matching_algos = [algo for algo in algos if 00102 algo.flash_start == start and algo.flash_size == size] 00103 return matching_algos if len(matching_algos) == 1 else algos 00104 00105 00106 if __name__ == '__main__': 00107 main()
Generated on Sun Jul 17 2022 08:25:22 by
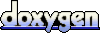