
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
__init__.py
00001 # mbed SDK 00002 # Copyright (c) 2011-2016 ARM Limited 00003 # 00004 # Licensed under the Apache License, Version 2.0 (the "License"); 00005 # you may not use this file except in compliance with the License. 00006 # You may obtain a copy of the License at 00007 # 00008 # http://www.apache.org/licenses/LICENSE-2.0 00009 # 00010 # Unless required by applicable law or agreed to in writing, software 00011 # distributed under the License is distributed on an "AS IS" BASIS, 00012 # WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 # See the License for the specific language governing permissions and 00014 # limitations under the License. 00015 00016 from os.path import join, exists, realpath, relpath, basename, isfile, splitext 00017 from os import makedirs, listdir 00018 import json 00019 00020 from tools.export.makefile import Makefile, GccArm, Armc5, IAR 00021 00022 class VSCode (Makefile ): 00023 """Generic VSCode project. Intended to be subclassed by classes that 00024 specify a type of Makefile. 00025 """ 00026 def generate (self): 00027 """Generate Makefile and VSCode launch and task files 00028 """ 00029 super(VSCode, self).generate() 00030 ctx = { 00031 'name': self.project_name, 00032 'elf_location': join('BUILD', self.project_name)+'.elf', 00033 'c_symbols': self.toolchain.get_symbols(), 00034 'asm_symbols': self.toolchain.get_symbols(True), 00035 'target': self.target, 00036 'include_paths': self.resources.inc_dirs, 00037 'load_exe': str(self.LOAD_EXE).lower() 00038 } 00039 00040 if not exists(join(self.export_dir, '.vscode')): 00041 makedirs(join(self.export_dir, '.vscode')) 00042 00043 self.gen_file('vscode/tasks.tmpl', ctx, 00044 join('.vscode', 'tasks.json')) 00045 self.gen_file('vscode/launch.tmpl', ctx, 00046 join('.vscode', 'launch.json')) 00047 self.gen_file('vscode/settings.tmpl', ctx, 00048 join('.vscode', 'settings.json')) 00049 00050 # So.... I want all .h and .hpp files in self.resources.inc_dirs 00051 all_directories = [] 00052 00053 for directory in self.resources.inc_dirs: 00054 if not directory: 00055 continue 00056 00057 if directory == ".": 00058 all_directories.append("${workspaceRoot}/*") 00059 else: 00060 all_directories.append(directory.replace("./", "${workspaceRoot}/") + "/*") 00061 00062 cpp_props = { 00063 "configurations": [ 00064 { 00065 "name": "Windows", 00066 "includePath": [x.replace("/", "\\") for x in all_directories], 00067 "defines": [symbol for symbol in self.toolchain.get_symbols()] 00068 }, 00069 { 00070 "name": "Mac", 00071 "includePath": all_directories, 00072 "defines": [symbol for symbol in self.toolchain.get_symbols()] 00073 }, 00074 { 00075 "name": "Linux", 00076 "includePath": all_directories, 00077 "defines": [symbol for symbol in self.toolchain.get_symbols()] 00078 } 00079 ] 00080 } 00081 00082 with open(join(self.export_dir, '.vscode', 'c_cpp_properties.json'), 'w') as outfile: 00083 json.dump(cpp_props, outfile, indent=4, separators=(',', ': ')) 00084 00085 00086 class VSCodeGcc(VSCode , GccArm): 00087 LOAD_EXE = True 00088 NAME = "VSCode-GCC-ARM" 00089 00090 class VSCodeArmc5(VSCode , Armc5): 00091 LOAD_EXE = True 00092 NAME = "VSCode-Armc5" 00093 00094 class VSCodeIAR(VSCode , IAR): 00095 LOAD_EXE = True 00096 NAME = "VSCode-IAR" 00097 00098
Generated on Sun Jul 17 2022 08:25:18 by
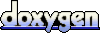