
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
example4.cpp
Go to the documentation of this file.
00001 /* 00002 * mbed Microcontroller Library 00003 * Copyright (c) 2006-2016 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 * 00017 */ 00018 00019 /** @file example4.cpp Test case to demonstrate a subset of the API functions each work correctly. 00020 * 00021 * Test case created from Issue 10 code supplied by Motti Gondabi. The code: 00022 * - creates a KV 00023 * - writes the KV 00024 * - closes the KV 00025 * - flushes the KV 00026 * - opens the KV. 00027 * - deletes the KV. 00028 * - flushes empty configuration store. 00029 * 00030 * The test case makes sure that the implementation can flush an empty configuration store 00031 * without causing errors. This has only been possible since flash-journal-strategy-sequential 00032 * v0.4.0. 00033 */ 00034 00035 #include "mbed.h" 00036 #include "cfstore_config.h" 00037 #include "cfstore_test.h" 00038 #include "cfstore_debug.h" 00039 #include "Driver_Common.h" 00040 #include "configuration_store.h" 00041 #include "utest/utest.h" 00042 #include "unity/unity.h" 00043 #include "greentea-client/test_env.h" 00044 #ifdef YOTTA_CFG_CONFIG_UVISOR 00045 #include "uvisor-lib/uvisor-lib.h" 00046 #endif /* YOTTA_CFG_CONFIG_UVISOR */ 00047 00048 #include <stdio.h> 00049 #include <stdlib.h> 00050 #include <string.h> 00051 #include <assert.h> 00052 #include <inttypes.h> 00053 00054 using namespace utest::v1; 00055 00056 static char cfstore_example4_utest_msg_g[CFSTORE_UTEST_MSG_BUF_SIZE]; 00057 00058 /* defines */ 00059 /// @cond CFSTORE_DOXYGEN_DISABLE 00060 #define PvMemSet memset 00061 #define PvStrLen strlen 00062 #define PvKeyValue_t cfstore_kv_data_t 00063 00064 ARM_CFSTORE_DRIVER *gCfStoreDriver = &cfstore_driver; 00065 /// @endcond 00066 00067 static control_t cfstore_example4_test_00(const size_t call_count) 00068 { 00069 int32_t ret = ARM_DRIVER_ERROR; 00070 ARM_CFSTORE_CAPABILITIES caps;; 00071 00072 (void) call_count; 00073 00074 /* initialise the context */ 00075 caps = gCfStoreDriver->GetCapabilities(); 00076 CFSTORE_LOG("%s:INITIALIZING: caps.asynchronous_ops=%lu\n", __func__, caps.asynchronous_ops); 00077 if(caps.asynchronous_ops == 1){ 00078 /* This is a sync mode only test. If this test is not built for sync mode, then skip testing return true 00079 * This means the test will conveniently pass when run in CI as part of async mode testing */ 00080 CFSTORE_LOG("*** Skipping test as binary built for flash journal async mode, and this test is sync-only%s", "\n"); 00081 return CaseNext; 00082 } 00083 ret = cfstore_test_startup(); 00084 CFSTORE_TEST_UTEST_MESSAGE(cfstore_example4_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to perform test startup (ret=%d).\n", __func__, (int) ret); 00085 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_example4_utest_msg_g); 00086 return CaseNext; 00087 } 00088 00089 00090 /* used for sync mode build only */ 00091 #if defined STORAGE_DRIVER_CONFIG_HARDWARE_MTD_ASYNC_OPS && STORAGE_DRIVER_CONFIG_HARDWARE_MTD_ASYNC_OPS==0 00092 00093 static const PvKeyValue_t testDataKeyValue[] = { 00094 { "com.arm.mbed.spv.assets.dtls", "This Is my DTLS Secret" }, 00095 { "com.arm.mbed.spv.assets.asset1.payload", "The Rolling Stone" }, 00096 { "com.arm.mbed.spv.assets.asset2.payload", "Grumpy old man" }, 00097 { "com.arm.mbed.spv.assets.asset3.payload", "Delete this asset payload" }, 00098 }; 00099 00100 00101 static int32_t CreateKeyValueStore( 00102 const char *keyName, 00103 const char *data, 00104 ARM_CFSTORE_SIZE *dataLength, 00105 ARM_CFSTORE_KEYDESC *keyDesc) 00106 { 00107 int32_t cfsStatus = ARM_DRIVER_ERROR; 00108 ARM_CFSTORE_SIZE valueLength = 0; 00109 ARM_CFSTORE_HANDLE_INIT(hkey); 00110 00111 valueLength = *dataLength; 00112 cfsStatus = gCfStoreDriver->Create(keyName, valueLength, keyDesc, hkey); 00113 CFSTORE_TEST_UTEST_MESSAGE(cfstore_example4_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Create() failed (cfsStatus=%d)\n", __func__, (int) cfsStatus); 00114 TEST_ASSERT_MESSAGE(cfsStatus >= ARM_DRIVER_OK, cfstore_example4_utest_msg_g); 00115 00116 valueLength = *dataLength; 00117 cfsStatus = gCfStoreDriver->Write(hkey, data, &valueLength); 00118 CFSTORE_TEST_UTEST_MESSAGE(cfstore_example4_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Write() failed (cfsStatus=%d)\n", __func__, (int) cfsStatus); 00119 TEST_ASSERT_MESSAGE(cfsStatus >= ARM_DRIVER_OK, cfstore_example4_utest_msg_g); 00120 CFSTORE_TEST_UTEST_MESSAGE(cfstore_example4_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: valueLength != *dataLength\n", __func__); 00121 TEST_ASSERT_MESSAGE(valueLength == *dataLength, cfstore_example4_utest_msg_g); 00122 00123 cfsStatus = gCfStoreDriver->Close(hkey); 00124 CFSTORE_TEST_UTEST_MESSAGE(cfstore_example4_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Close() failed (cfsStatus=%d)\n", __func__, (int) cfsStatus); 00125 TEST_ASSERT_MESSAGE(cfsStatus >= ARM_DRIVER_OK, cfstore_example4_utest_msg_g); 00126 00127 cfsStatus = gCfStoreDriver->Flush(); 00128 CFSTORE_TEST_UTEST_MESSAGE(cfstore_example4_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Flush() failed (cfsStatus=%d)\n", __func__, (int) cfsStatus); 00129 TEST_ASSERT_MESSAGE(cfsStatus >= ARM_DRIVER_OK, cfstore_example4_utest_msg_g); 00130 00131 return ARM_DRIVER_OK; 00132 } 00133 00134 00135 static control_t cfstore_example4_test_01(const size_t call_count) 00136 { 00137 int32_t cfsStatus = ARM_DRIVER_ERROR; 00138 ARM_CFSTORE_KEYDESC kdesc; 00139 ARM_CFSTORE_SIZE valueLen; 00140 ARM_CFSTORE_FMODE flags; 00141 ARM_CFSTORE_HANDLE_INIT(hkey); 00142 00143 (void) call_count; 00144 PvMemSet(&kdesc, 0, sizeof(kdesc)); 00145 00146 kdesc.drl = ARM_RETENTION_NVM; 00147 valueLen = PvStrLen(testDataKeyValue[0].value); 00148 00149 cfsStatus = gCfStoreDriver->Initialize(NULL, NULL); 00150 CFSTORE_TEST_UTEST_MESSAGE(cfstore_example4_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Initialize() failed (cfsStatus=%d)\n", __func__, (int) cfsStatus); 00151 TEST_ASSERT_MESSAGE(cfsStatus >= ARM_DRIVER_OK, cfstore_example4_utest_msg_g); 00152 00153 cfsStatus = CreateKeyValueStore(testDataKeyValue[0].key_name, testDataKeyValue[0].value, &valueLen, &kdesc); 00154 CFSTORE_TEST_UTEST_MESSAGE(cfstore_example4_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: CreateKeyValueStore() failed (cfsStatus=%d)\n", __func__, (int) cfsStatus); 00155 TEST_ASSERT_MESSAGE(cfsStatus >= ARM_DRIVER_OK, cfstore_example4_utest_msg_g); 00156 00157 PvMemSet(&flags, 0, sizeof(flags)); 00158 00159 cfsStatus = gCfStoreDriver->Open(testDataKeyValue[0].key_name, flags, hkey); 00160 CFSTORE_TEST_UTEST_MESSAGE(cfstore_example4_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Open() failed (cfsStatus=%d)\n", __func__, (int) cfsStatus); 00161 TEST_ASSERT_MESSAGE(cfsStatus >= ARM_DRIVER_OK, cfstore_example4_utest_msg_g); 00162 00163 cfsStatus = gCfStoreDriver->Delete(hkey); 00164 CFSTORE_TEST_UTEST_MESSAGE(cfstore_example4_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Delete() failed (cfsStatus=%d)\n", __func__, (int) cfsStatus); 00165 TEST_ASSERT_MESSAGE(cfsStatus >= ARM_DRIVER_OK, cfstore_example4_utest_msg_g); 00166 00167 cfsStatus = gCfStoreDriver->Close(hkey); 00168 CFSTORE_TEST_UTEST_MESSAGE(cfstore_example4_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Close() failed (cfsStatus=%d)\n", __func__, (int) cfsStatus); 00169 TEST_ASSERT_MESSAGE(cfsStatus >= ARM_DRIVER_OK, cfstore_example4_utest_msg_g); 00170 00171 cfsStatus = gCfStoreDriver->Flush(); 00172 CFSTORE_TEST_UTEST_MESSAGE(cfstore_example4_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Flush() failed (cfsStatus=%d)\n", __func__, (int) cfsStatus); 00173 TEST_ASSERT_MESSAGE(cfsStatus >= ARM_DRIVER_OK, cfstore_example4_utest_msg_g); 00174 00175 cfsStatus = gCfStoreDriver->Uninitialize(); 00176 CFSTORE_TEST_UTEST_MESSAGE(cfstore_example4_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Uninitialize() failed (cfsStatus=%d)\n", __func__, (int) cfsStatus); 00177 TEST_ASSERT_MESSAGE(cfsStatus >= ARM_DRIVER_OK, cfstore_example4_utest_msg_g); 00178 00179 return CaseNext; 00180 } 00181 #endif // STORAGE_DRIVER_CONFIG_HARDWARE_MTD_ASYNC_OPS 00182 00183 00184 /// @cond CFSTORE_DOXYGEN_DISABLE 00185 utest::v1::status_t greentea_setup(const size_t number_of_cases) 00186 { 00187 GREENTEA_SETUP(400, "default_auto"); 00188 return greentea_test_setup_handler(number_of_cases); 00189 } 00190 00191 Case cases[] = { 00192 /* 1 2 3 4 5 6 7 */ 00193 /* 1234567890123456789012345678901234567890123456789012345678901234567890 */ 00194 Case("EXAMPLE4_test_00", cfstore_example4_test_00), 00195 #if defined STORAGE_DRIVER_CONFIG_HARDWARE_MTD_ASYNC_OPS && STORAGE_DRIVER_CONFIG_HARDWARE_MTD_ASYNC_OPS==0 00196 Case("EXAMPLE4_test_01", cfstore_example4_test_01), 00197 #endif // STORAGE_DRIVER_CONFIG_HARDWARE_MTD_ASYNC_OPS 00198 }; 00199 00200 00201 /* Declare your test specification with a custom setup handler */ 00202 Specification specification(greentea_setup, cases); 00203 00204 int main() 00205 { 00206 return !Harness::run(specification); 00207 } 00208 /// @endcond
Generated on Sun Jul 17 2022 08:25:22 by
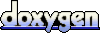