
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ethernet_mac_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** \file ethernet_mac_api.h 00016 * \brief Ethernet MAC API 00017 */ 00018 00019 #ifndef ETHERNET_MAC_API_H 00020 #define ETHERNET_MAC_API_H 00021 00022 #include <inttypes.h> 00023 #include "platform/arm_hal_phy.h" 00024 00025 #ifdef __cplusplus 00026 extern "C" { 00027 #endif 00028 00029 #define ETHERTYPE_IPV4 0x0800 /**< ethernet type for IPv4 */ 00030 #define ETHERTYPE_ARP 0x0806 /**< ethernet type for ARP */ 00031 #define ETHERTYPE_802_1Q_TAG 0x8100 /**< ethernet type for 802_1Q */ 00032 #define ETHERTYPE_IPV6 0x86dd /**< ethernet type for IPv6 */ 00033 00034 typedef struct eth_mac_api_s eth_mac_api_t; 00035 00036 /** 00037 * \brief Struct eth_data_conf_s defines arguments for data confirm message 00038 */ 00039 typedef struct eth_data_conf_s { 00040 uint8_t msduHandle; /**< Handle associated with MSDU */ 00041 uint8_t status; /**< Status of the last transaction */ 00042 }eth_data_conf_t; 00043 00044 /** 00045 * \brief Struct eth_data_req_s defines arguments for data request message 00046 */ 00047 typedef struct eth_data_req_s { 00048 uint16_t msduLength; /**< Service data unit length */ 00049 uint8_t *msdu; /**< Service data unit */ 00050 uint8_t *srcAddress; /**< Source address */ 00051 uint8_t *dstAddress; /**< Destination address */ 00052 uint16_t etehernet_type; /**< Ethernet type */ 00053 uint8_t msduHandle; /**< Handle associated with MSDU */ 00054 } eth_data_req_t; 00055 00056 /** 00057 * \brief Struct eth_data_ind_s defines arguments for data indication message 00058 */ 00059 typedef struct eth_data_ind_s { 00060 uint16_t msduLength; /**< Service data unit length */ 00061 uint8_t *msdu; /**< Service data unit */ 00062 uint8_t srcAddress[6]; /**< Source address */ 00063 uint8_t dstAddress[6]; /**< Destination address */ 00064 uint16_t etehernet_type; /**< Ethernet type */ 00065 uint8_t link_quality; /**< Link quality */ 00066 int8_t dbm; /**< measured dBm */ 00067 }eth_data_ind_t; 00068 00069 /** 00070 * @brief Creates ethernet MAC API instance which will use driver given 00071 * @param driver_id Ethernet driver id. Must be valid 00072 * @return New MAC instance if successful, NULL otherwise 00073 */ 00074 extern eth_mac_api_t *ethernet_mac_create(int8_t driver_id); 00075 00076 /** 00077 * @brief Destroy ethernet MAC API instance 00078 * Call this only for freeing all allocated memory and when mac is total unused 00079 * 00080 * @param mac_api Removed mac class pointer 00081 * @return -1 Unknow MAC 00082 * @return 0 Mac class is removed 00083 */ 00084 extern int8_t ethernet_mac_destroy(eth_mac_api_t *mac_api); 00085 00086 /** 00087 * @brief data_request data request call 00088 * @param api API to handle the request 00089 * @param data Data containing request parameters 00090 */ 00091 typedef void eth_mac_data_request(const eth_mac_api_t* api, const eth_data_req_t *data); 00092 00093 /** 00094 * @brief data_confirm confirm is called as a response to data_request 00095 * @param api The API which handled the request 00096 * @param data Data containing confirm parameters 00097 */ 00098 typedef void eth_mac_data_confirm(const eth_mac_api_t* api, const eth_data_conf_t *data ); 00099 00100 /** 00101 * @brief data_indication Data indication is called when MAC layer has received data 00102 * @param api The API which handled the response 00103 * @param data Data containing indication parameters 00104 */ 00105 typedef void eth_mac_data_indication(const eth_mac_api_t* api, const eth_data_ind_t *data ); 00106 00107 /** 00108 * @brief Set 48 bit address from MAC 00109 * @param api API to handle the request 00110 * @param mac48 Pointer having mac address to be set 00111 * @return 0 if successful, -1 otherwise 00112 */ 00113 typedef int8_t eth_mac_mac48_address_set(const eth_mac_api_t* api, const uint8_t *mac48); 00114 00115 /** 00116 * @brief Read 48 bit address from MAC 00117 * @param api API to handle the request 00118 * @param mac48_buf Pointer where mac address can be written 00119 * @return 0 if successful, -1 otherwise 00120 */ 00121 typedef int8_t eth_mac_mac48_address_get(const eth_mac_api_t* api, uint8_t *mac48_buf); 00122 00123 /** 00124 * @brief Upper layer will call this function, when MAC is taken into use 00125 * @param api API to initialize 00126 * @param conf_cb Callback for confirm type of messages 00127 * @param ind_cb Callback for indication type of messages 00128 * @param parent_id Upper layer identifier 00129 * @return 0 if success; -1 if api is NULL or not found 00130 */ 00131 typedef int8_t eth_mac_api_initialize(eth_mac_api_t *api, eth_mac_data_confirm *conf_cb, 00132 eth_mac_data_indication *ind_cb, uint8_t parent_id); 00133 00134 /** 00135 * \brief Struct eth_mac_api_s defines functions for two-way communications between ethernet MAC and Upper layer. 00136 */ 00137 struct eth_mac_api_s { 00138 eth_mac_api_initialize *mac_initialize; /**< Callback function for MAC initialization */ 00139 00140 eth_mac_data_request *data_req; /**< Callback function for data request */ 00141 eth_mac_data_confirm *data_conf_cb; /**< Callback function for data confirmation */ 00142 eth_mac_data_indication *data_ind_cb; /**< Callback function for data indication */ 00143 00144 eth_mac_mac48_address_set *mac48_set; /**< Setter for MAC address */ 00145 eth_mac_mac48_address_get *mac48_get; /**< Getter for MAC address */ 00146 00147 uint8_t parent_id; /**< Upper layer ID */ 00148 bool address_resolution_needed; /**< Normal ethernet should set this true for tunnel or false for slip */ 00149 }; 00150 00151 #ifdef __cplusplus 00152 } 00153 #endif 00154 00155 #endif // ETHERNET_MAC_API_H 00156
Generated on Sun Jul 17 2022 08:25:22 by
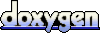