
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
emac_stack_mem.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2016 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_EMAC_STACK_MEM_H 00017 #define MBED_EMAC_STACK_MEM_H 00018 00019 #if DEVICE_EMAC 00020 00021 #include <stdint.h> 00022 00023 /** 00024 * Stack memory module 00025 * 00026 * This interface provides abstraction for memory modules used in different IP stacks (often to accommodate zero copy). 00027 * Emac interface may be required to accept output packets and provide received data using this stack specific API. 00028 * This header should be implemented for each IP stack, so that we keep emacs module independent. 00029 */ 00030 typedef void emac_stack_mem_t; 00031 typedef void emac_stack_mem_chain_t; 00032 typedef void emac_stack_t; 00033 00034 /** 00035 * Allocates stack memory 00036 * 00037 * @param stack Emac stack context 00038 * @param size Size of memory to allocate 00039 * @param align Memory alignment requirements 00040 * @return Allocated memory struct, or NULL in case of error 00041 */ 00042 emac_stack_mem_t *emac_stack_mem_alloc(emac_stack_t* stack, uint32_t size, uint32_t align); 00043 00044 /** 00045 * Free memory allocated using @a stack_mem_alloc 00046 * 00047 * @param stack Emac stack context 00048 * @param mem Memory to be freed 00049 */ 00050 void emac_stack_mem_free(emac_stack_t* stack, emac_stack_mem_t *mem); 00051 00052 /** 00053 * Copy memory 00054 * 00055 * @param stack Emac stack context 00056 * @param to Memory to copy to 00057 * @param from Memory to copy from 00058 */ 00059 void emac_stack_mem_copy(emac_stack_t* stack, emac_stack_mem_t *to, emac_stack_mem_t *from); 00060 00061 /** 00062 * Return pointer to the payload 00063 * 00064 * @param stack Emac stack context 00065 * @param mem Memory structure 00066 * @return Pointer to the payload 00067 */ 00068 void *emac_stack_mem_ptr(emac_stack_t* stack, emac_stack_mem_t *mem); 00069 00070 /** 00071 * Return actual payload size 00072 * 00073 * @param stack Emac stack context 00074 * @param mem Memory structure 00075 * @return Size in bytes 00076 */ 00077 uint32_t emac_stack_mem_len(emac_stack_t* stack, emac_stack_mem_t *mem); 00078 00079 /** 00080 * Sets the actual payload size (the allocated payload size will not change) 00081 * 00082 * @param stack Emac stack context 00083 * @param mem Memory structure 00084 * @param len Actual payload size 00085 */ 00086 void emac_stack_mem_set_len(emac_stack_t* stack, emac_stack_mem_t *mem, uint32_t len); 00087 00088 /** 00089 * Returns first memory structure from the list and move the head to point to the next node 00090 * 00091 * @param stack Emac stack context 00092 * @param chain Pointer to the list 00093 * @return First memory structure from the list 00094 */ 00095 emac_stack_mem_t *emac_stack_mem_chain_dequeue(emac_stack_t* stack, emac_stack_mem_chain_t **chain); 00096 00097 /** 00098 * Return total length of the memory chain 00099 * 00100 * @param stack Emac stack context 00101 * @param chain Memory chain 00102 * @return Chain length 00103 */ 00104 uint32_t emac_stack_mem_chain_len(emac_stack_t* stack, emac_stack_mem_chain_t *chain); 00105 00106 /** 00107 * Increases the reference counter for the memory 00108 * 00109 * @param stack Emac stack context 00110 * @param mem Memory structure 00111 */ 00112 void emac_stack_mem_ref(emac_stack_t* stack, emac_stack_mem_t *mem); 00113 00114 #endif /* DEVICE_EMAC */ 00115 00116 #endif /* EMAC_MBED_STACK_MEM_h */
Generated on Sun Jul 17 2022 08:25:22 by
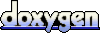