
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
dev_null_auto.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 class DevNullTest(): 00019 00020 def check_readline(self, selftest, text): 00021 """ Reads line from serial port and checks if text was part of read string 00022 """ 00023 result = False 00024 c = selftest.mbed.serial_readline() 00025 if c and text in c: 00026 result = True 00027 return result 00028 00029 def test(self, selftest): 00030 result = True 00031 # Test should print some text and later stop printing 00032 # 'MBED: re-routing stdout to /null' 00033 res = self.check_readline(selftest, "re-routing stdout to /null") 00034 if not res: 00035 # We haven't read preamble line 00036 result = False 00037 else: 00038 # Check if there are printed characters 00039 str = '' 00040 for i in range(3): 00041 c = selftest.mbed.serial_read(32) 00042 if c is None: 00043 return selftest.RESULT_IO_SERIAL 00044 else: 00045 str += c 00046 if len(str) > 0: 00047 result = False 00048 break 00049 selftest.notify("Received %d bytes: %s"% (len(str), str)) 00050 return selftest.RESULT_SUCCESS if result else selftest.RESULT_FAILURE
Generated on Sun Jul 17 2022 08:25:21 by
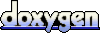