
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
coap_message_handler_stub.c
00001 /* 00002 * Copyright (c) 2015-2017 ARM Limited. All Rights Reserved. 00003 */ 00004 00005 #include "coap_message_handler_stub.h" 00006 00007 coap_message_handler_stub_def coap_message_handler_stub; 00008 00009 coap_msg_handler_t *coap_message_handler_init(void *(*used_malloc_func_ptr)(uint16_t), void (*used_free_func_ptr)(void *), 00010 uint8_t (*used_tx_callback_ptr)(uint8_t *, uint16_t, sn_nsdl_addr_s *, void *)) 00011 { 00012 if(coap_message_handler_stub.coap_ptr){ 00013 coap_message_handler_stub.coap_ptr->sn_coap_service_malloc = used_malloc_func_ptr; 00014 coap_message_handler_stub.coap_ptr->sn_coap_service_free = used_free_func_ptr; 00015 coap_message_handler_stub.coap_ptr->sn_coap_tx_callback = used_tx_callback_ptr; 00016 } 00017 return coap_message_handler_stub.coap_ptr; 00018 } 00019 00020 void transaction_delete(coap_transaction_t *this) 00021 { 00022 00023 } 00024 00025 void transactions_delete_all(uint8_t *address_ptr, uint16_t port) 00026 { 00027 00028 } 00029 int8_t coap_message_handler_destroy(coap_msg_handler_t *handle) 00030 { 00031 return coap_message_handler_stub.int8_value; 00032 } 00033 00034 coap_transaction_t *coap_message_handler_transaction_valid(coap_transaction_t *tr_ptr) 00035 { 00036 return coap_message_handler_stub.coap_ptr; 00037 } 00038 00039 coap_transaction_t *coap_message_handler_find_transaction(uint8_t *address_ptr, uint16_t port) 00040 { 00041 return coap_message_handler_stub.coap_tx_ptr; 00042 } 00043 00044 int16_t coap_message_handler_coap_msg_process(coap_msg_handler_t *handle, int8_t socket_id, const uint8_t source_addr_ptr[static 16], uint16_t port, const uint8_t dst_addr_ptr[static 16], 00045 uint8_t *data_ptr, uint16_t data_len, int16_t (cb)(int8_t, sn_coap_hdr_s *, coap_transaction_t *)) 00046 { 00047 coap_message_handler_stub.cb = cb; 00048 return coap_message_handler_stub.int16_value; 00049 } 00050 00051 uint16_t coap_message_handler_request_send(coap_msg_handler_t *handle, int8_t service_id, uint8_t options, 00052 const uint8_t destination_addr[static 16], uint16_t destination_port, sn_coap_msg_type_e msg_type, sn_coap_msg_code_e msg_code, 00053 const char *uri, sn_coap_content_format_e cont_type, const uint8_t *payload_ptr, uint16_t payload_len, coap_message_handler_response_recv *request_response_cb) 00054 { 00055 return coap_message_handler_stub.uint16_value; 00056 } 00057 00058 int8_t coap_message_handler_response_send(coap_msg_handler_t *handle, int8_t service_id, uint8_t options, sn_coap_hdr_s *request_ptr, sn_coap_msg_code_e message_code,sn_coap_content_format_e content_type, const uint8_t *payload_ptr, uint16_t payload_len) 00059 { 00060 return coap_message_handler_stub.int8_value; 00061 } 00062 00063 int8_t coap_message_handler_request_delete(coap_msg_handler_t *handle, int8_t service_id, uint16_t msg_id) 00064 { 00065 return 0; 00066 } 00067 00068 int8_t coap_message_handler_exec(coap_msg_handler_t *handle, uint32_t current_time) 00069 { 00070 return coap_message_handler_stub.int8_value; 00071 } 00072
Generated on Sun Jul 17 2022 08:25:21 by
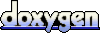