
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
cipher.h
Go to the documentation of this file.
00001 /** 00002 * \file cipher.h 00003 * 00004 * \brief Generic cipher wrapper. 00005 * 00006 * \author Adriaan de Jong <dejong@fox-it.com> 00007 * 00008 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00009 * SPDX-License-Identifier: Apache-2.0 00010 * 00011 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00012 * not use this file except in compliance with the License. 00013 * You may obtain a copy of the License at 00014 * 00015 * http://www.apache.org/licenses/LICENSE-2.0 00016 * 00017 * Unless required by applicable law or agreed to in writing, software 00018 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00019 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00020 * See the License for the specific language governing permissions and 00021 * limitations under the License. 00022 * 00023 * This file is part of mbed TLS (https://tls.mbed.org) 00024 */ 00025 00026 #ifndef MBEDTLS_CIPHER_H 00027 #define MBEDTLS_CIPHER_H 00028 00029 #if !defined(MBEDTLS_CONFIG_FILE) 00030 #include "config.h" 00031 #else 00032 #include MBEDTLS_CONFIG_FILE 00033 #endif 00034 00035 #include <stddef.h> 00036 00037 #if defined(MBEDTLS_GCM_C) || defined(MBEDTLS_CCM_C) 00038 #define MBEDTLS_CIPHER_MODE_AEAD 00039 #endif 00040 00041 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00042 #define MBEDTLS_CIPHER_MODE_WITH_PADDING 00043 #endif 00044 00045 #if defined(MBEDTLS_ARC4_C) 00046 #define MBEDTLS_CIPHER_MODE_STREAM 00047 #endif 00048 00049 #if ( defined(__ARMCC_VERSION) || defined(_MSC_VER) ) && \ 00050 !defined(inline) && !defined(__cplusplus) 00051 #define inline __inline 00052 #endif 00053 00054 #define MBEDTLS_ERR_CIPHER_FEATURE_UNAVAILABLE -0x6080 /**< The selected feature is not available. */ 00055 #define MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA -0x6100 /**< Bad input parameters to function. */ 00056 #define MBEDTLS_ERR_CIPHER_ALLOC_FAILED -0x6180 /**< Failed to allocate memory. */ 00057 #define MBEDTLS_ERR_CIPHER_INVALID_PADDING -0x6200 /**< Input data contains invalid padding and is rejected. */ 00058 #define MBEDTLS_ERR_CIPHER_FULL_BLOCK_EXPECTED -0x6280 /**< Decryption of block requires a full block. */ 00059 #define MBEDTLS_ERR_CIPHER_AUTH_FAILED -0x6300 /**< Authentication failed (for AEAD modes). */ 00060 #define MBEDTLS_ERR_CIPHER_INVALID_CONTEXT -0x6380 /**< The context is invalid, eg because it was free()ed. */ 00061 00062 #define MBEDTLS_CIPHER_VARIABLE_IV_LEN 0x01 /**< Cipher accepts IVs of variable length */ 00063 #define MBEDTLS_CIPHER_VARIABLE_KEY_LEN 0x02 /**< Cipher accepts keys of variable length */ 00064 00065 #ifdef __cplusplus 00066 extern "C" { 00067 #endif 00068 00069 typedef enum { 00070 MBEDTLS_CIPHER_ID_NONE = 0, 00071 MBEDTLS_CIPHER_ID_NULL, 00072 MBEDTLS_CIPHER_ID_AES, 00073 MBEDTLS_CIPHER_ID_DES, 00074 MBEDTLS_CIPHER_ID_3DES, 00075 MBEDTLS_CIPHER_ID_CAMELLIA, 00076 MBEDTLS_CIPHER_ID_BLOWFISH, 00077 MBEDTLS_CIPHER_ID_ARC4, 00078 } mbedtls_cipher_id_t; 00079 00080 typedef enum { 00081 MBEDTLS_CIPHER_NONE = 0, 00082 MBEDTLS_CIPHER_NULL, 00083 MBEDTLS_CIPHER_AES_128_ECB, 00084 MBEDTLS_CIPHER_AES_192_ECB, 00085 MBEDTLS_CIPHER_AES_256_ECB, 00086 MBEDTLS_CIPHER_AES_128_CBC, 00087 MBEDTLS_CIPHER_AES_192_CBC, 00088 MBEDTLS_CIPHER_AES_256_CBC, 00089 MBEDTLS_CIPHER_AES_128_CFB128, 00090 MBEDTLS_CIPHER_AES_192_CFB128, 00091 MBEDTLS_CIPHER_AES_256_CFB128, 00092 MBEDTLS_CIPHER_AES_128_CTR, 00093 MBEDTLS_CIPHER_AES_192_CTR, 00094 MBEDTLS_CIPHER_AES_256_CTR, 00095 MBEDTLS_CIPHER_AES_128_GCM, 00096 MBEDTLS_CIPHER_AES_192_GCM, 00097 MBEDTLS_CIPHER_AES_256_GCM, 00098 MBEDTLS_CIPHER_CAMELLIA_128_ECB, 00099 MBEDTLS_CIPHER_CAMELLIA_192_ECB, 00100 MBEDTLS_CIPHER_CAMELLIA_256_ECB, 00101 MBEDTLS_CIPHER_CAMELLIA_128_CBC, 00102 MBEDTLS_CIPHER_CAMELLIA_192_CBC, 00103 MBEDTLS_CIPHER_CAMELLIA_256_CBC, 00104 MBEDTLS_CIPHER_CAMELLIA_128_CFB128, 00105 MBEDTLS_CIPHER_CAMELLIA_192_CFB128, 00106 MBEDTLS_CIPHER_CAMELLIA_256_CFB128, 00107 MBEDTLS_CIPHER_CAMELLIA_128_CTR, 00108 MBEDTLS_CIPHER_CAMELLIA_192_CTR, 00109 MBEDTLS_CIPHER_CAMELLIA_256_CTR, 00110 MBEDTLS_CIPHER_CAMELLIA_128_GCM, 00111 MBEDTLS_CIPHER_CAMELLIA_192_GCM, 00112 MBEDTLS_CIPHER_CAMELLIA_256_GCM, 00113 MBEDTLS_CIPHER_DES_ECB, 00114 MBEDTLS_CIPHER_DES_CBC, 00115 MBEDTLS_CIPHER_DES_EDE_ECB, 00116 MBEDTLS_CIPHER_DES_EDE_CBC, 00117 MBEDTLS_CIPHER_DES_EDE3_ECB, 00118 MBEDTLS_CIPHER_DES_EDE3_CBC, 00119 MBEDTLS_CIPHER_BLOWFISH_ECB, 00120 MBEDTLS_CIPHER_BLOWFISH_CBC, 00121 MBEDTLS_CIPHER_BLOWFISH_CFB64, 00122 MBEDTLS_CIPHER_BLOWFISH_CTR, 00123 MBEDTLS_CIPHER_ARC4_128, 00124 MBEDTLS_CIPHER_AES_128_CCM, 00125 MBEDTLS_CIPHER_AES_192_CCM, 00126 MBEDTLS_CIPHER_AES_256_CCM, 00127 MBEDTLS_CIPHER_CAMELLIA_128_CCM, 00128 MBEDTLS_CIPHER_CAMELLIA_192_CCM, 00129 MBEDTLS_CIPHER_CAMELLIA_256_CCM, 00130 } mbedtls_cipher_type_t; 00131 00132 typedef enum { 00133 MBEDTLS_MODE_NONE = 0, 00134 MBEDTLS_MODE_ECB, 00135 MBEDTLS_MODE_CBC, 00136 MBEDTLS_MODE_CFB, 00137 MBEDTLS_MODE_OFB, /* Unused! */ 00138 MBEDTLS_MODE_CTR, 00139 MBEDTLS_MODE_GCM, 00140 MBEDTLS_MODE_STREAM, 00141 MBEDTLS_MODE_CCM, 00142 } mbedtls_cipher_mode_t; 00143 00144 typedef enum { 00145 MBEDTLS_PADDING_PKCS7 = 0, /**< PKCS7 padding (default) */ 00146 MBEDTLS_PADDING_ONE_AND_ZEROS, /**< ISO/IEC 7816-4 padding */ 00147 MBEDTLS_PADDING_ZEROS_AND_LEN, /**< ANSI X.923 padding */ 00148 MBEDTLS_PADDING_ZEROS, /**< zero padding (not reversible!) */ 00149 MBEDTLS_PADDING_NONE, /**< never pad (full blocks only) */ 00150 } mbedtls_cipher_padding_t ; 00151 00152 typedef enum { 00153 MBEDTLS_OPERATION_NONE = -1, 00154 MBEDTLS_DECRYPT = 0, 00155 MBEDTLS_ENCRYPT, 00156 } mbedtls_operation_t; 00157 00158 enum { 00159 /** Undefined key length */ 00160 MBEDTLS_KEY_LENGTH_NONE = 0, 00161 /** Key length, in bits (including parity), for DES keys */ 00162 MBEDTLS_KEY_LENGTH_DES = 64, 00163 /** Key length, in bits (including parity), for DES in two key EDE */ 00164 MBEDTLS_KEY_LENGTH_DES_EDE = 128, 00165 /** Key length, in bits (including parity), for DES in three-key EDE */ 00166 MBEDTLS_KEY_LENGTH_DES_EDE3 = 192, 00167 }; 00168 00169 /** Maximum length of any IV, in bytes */ 00170 #define MBEDTLS_MAX_IV_LENGTH 16 00171 /** Maximum block size of any cipher, in bytes */ 00172 #define MBEDTLS_MAX_BLOCK_LENGTH 16 00173 00174 /** 00175 * Base cipher information (opaque struct). 00176 */ 00177 typedef struct mbedtls_cipher_base_t mbedtls_cipher_base_t; 00178 00179 /** 00180 * CMAC context (opaque struct). 00181 */ 00182 typedef struct mbedtls_cmac_context_t mbedtls_cmac_context_t; 00183 00184 /** 00185 * Cipher information. Allows cipher functions to be called in a generic way. 00186 */ 00187 typedef struct { 00188 /** Full cipher identifier (e.g. MBEDTLS_CIPHER_AES_256_CBC) */ 00189 mbedtls_cipher_type_t type; 00190 00191 /** Cipher mode (e.g. MBEDTLS_MODE_CBC) */ 00192 mbedtls_cipher_mode_t mode; 00193 00194 /** Cipher key length, in bits (default length for variable sized ciphers) 00195 * (Includes parity bits for ciphers like DES) */ 00196 unsigned int key_bitlen; 00197 00198 /** Name of the cipher */ 00199 const char * name; 00200 00201 /** IV/NONCE size, in bytes. 00202 * For cipher that accept many sizes: recommended size */ 00203 unsigned int iv_size; 00204 00205 /** Flags for variable IV size, variable key size, etc. */ 00206 int flags; 00207 00208 /** block size, in bytes */ 00209 unsigned int block_size; 00210 00211 /** Base cipher information and functions */ 00212 const mbedtls_cipher_base_t *base; 00213 00214 } mbedtls_cipher_info_t; 00215 00216 /** 00217 * Generic cipher context. 00218 */ 00219 typedef struct { 00220 /** Information about the associated cipher */ 00221 const mbedtls_cipher_info_t *cipher_info; 00222 00223 /** Key length to use */ 00224 int key_bitlen; 00225 00226 /** Operation that the context's key has been initialised for */ 00227 mbedtls_operation_t operation; 00228 00229 #if defined(MBEDTLS_CIPHER_MODE_WITH_PADDING) 00230 /** Padding functions to use, if relevant for cipher mode */ 00231 void (*add_padding)( unsigned char *output, size_t olen, size_t data_len ); 00232 int (*get_padding)( unsigned char *input, size_t ilen, size_t *data_len ); 00233 #endif 00234 00235 /** Buffer for data that hasn't been encrypted yet */ 00236 unsigned char unprocessed_data[MBEDTLS_MAX_BLOCK_LENGTH]; 00237 00238 /** Number of bytes that still need processing */ 00239 size_t unprocessed_len; 00240 00241 /** Current IV or NONCE_COUNTER for CTR-mode */ 00242 unsigned char iv[MBEDTLS_MAX_IV_LENGTH]; 00243 00244 /** IV size in bytes (for ciphers with variable-length IVs) */ 00245 size_t iv_size; 00246 00247 /** Cipher-specific context */ 00248 void *cipher_ctx; 00249 00250 #if defined(MBEDTLS_CMAC_C) 00251 /** CMAC Specific context */ 00252 mbedtls_cmac_context_t *cmac_ctx; 00253 #endif 00254 } mbedtls_cipher_context_t; 00255 00256 /** 00257 * \brief Returns the list of ciphers supported by the generic cipher module. 00258 * 00259 * \return a statically allocated array of ciphers, the last entry 00260 * is 0. 00261 */ 00262 const int *mbedtls_cipher_list( void ); 00263 00264 /** 00265 * \brief Returns the cipher information structure associated 00266 * with the given cipher name. 00267 * 00268 * \param cipher_name Name of the cipher to search for. 00269 * 00270 * \return the cipher information structure associated with the 00271 * given cipher_name, or NULL if not found. 00272 */ 00273 const mbedtls_cipher_info_t *mbedtls_cipher_info_from_string( const char *cipher_name ); 00274 00275 /** 00276 * \brief Returns the cipher information structure associated 00277 * with the given cipher type. 00278 * 00279 * \param cipher_type Type of the cipher to search for. 00280 * 00281 * \return the cipher information structure associated with the 00282 * given cipher_type, or NULL if not found. 00283 */ 00284 const mbedtls_cipher_info_t *mbedtls_cipher_info_from_type( const mbedtls_cipher_type_t cipher_type ); 00285 00286 /** 00287 * \brief Returns the cipher information structure associated 00288 * with the given cipher id, key size and mode. 00289 * 00290 * \param cipher_id Id of the cipher to search for 00291 * (e.g. MBEDTLS_CIPHER_ID_AES) 00292 * \param key_bitlen Length of the key in bits 00293 * \param mode Cipher mode (e.g. MBEDTLS_MODE_CBC) 00294 * 00295 * \return the cipher information structure associated with the 00296 * given cipher_type, or NULL if not found. 00297 */ 00298 const mbedtls_cipher_info_t *mbedtls_cipher_info_from_values( const mbedtls_cipher_id_t cipher_id, 00299 int key_bitlen, 00300 const mbedtls_cipher_mode_t mode ); 00301 00302 /** 00303 * \brief Initialize a cipher_context (as NONE) 00304 */ 00305 void mbedtls_cipher_init( mbedtls_cipher_context_t *ctx ); 00306 00307 /** 00308 * \brief Free and clear the cipher-specific context of ctx. 00309 * Freeing ctx itself remains the responsibility of the 00310 * caller. 00311 */ 00312 void mbedtls_cipher_free( mbedtls_cipher_context_t *ctx ); 00313 00314 /** 00315 * \brief Initialises and fills the cipher context structure with 00316 * the appropriate values. 00317 * 00318 * \note Currently also clears structure. In future versions you 00319 * will be required to call mbedtls_cipher_init() on the structure 00320 * first. 00321 * 00322 * \param ctx context to initialise. May not be NULL. 00323 * \param cipher_info cipher to use. 00324 * 00325 * \return 0 on success, 00326 * MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA on parameter failure, 00327 * MBEDTLS_ERR_CIPHER_ALLOC_FAILED if allocation of the 00328 * cipher-specific context failed. 00329 */ 00330 int mbedtls_cipher_setup( mbedtls_cipher_context_t *ctx, const mbedtls_cipher_info_t *cipher_info ); 00331 00332 /** 00333 * \brief Returns the block size of the given cipher. 00334 * 00335 * \param ctx cipher's context. Must have been initialised. 00336 * 00337 * \return size of the cipher's blocks, or 0 if ctx has not been 00338 * initialised. 00339 */ 00340 static inline unsigned int mbedtls_cipher_get_block_size( const mbedtls_cipher_context_t *ctx ) 00341 { 00342 if( NULL == ctx || NULL == ctx->cipher_info ) 00343 return 0; 00344 00345 return ctx->cipher_info->block_size; 00346 } 00347 00348 /** 00349 * \brief Returns the mode of operation for the cipher. 00350 * (e.g. MBEDTLS_MODE_CBC) 00351 * 00352 * \param ctx cipher's context. Must have been initialised. 00353 * 00354 * \return mode of operation, or MBEDTLS_MODE_NONE if ctx 00355 * has not been initialised. 00356 */ 00357 static inline mbedtls_cipher_mode_t mbedtls_cipher_get_cipher_mode( const mbedtls_cipher_context_t *ctx ) 00358 { 00359 if( NULL == ctx || NULL == ctx->cipher_info ) 00360 return MBEDTLS_MODE_NONE; 00361 00362 return ctx->cipher_info->mode; 00363 } 00364 00365 /** 00366 * \brief Returns the size of the cipher's IV/NONCE in bytes. 00367 * 00368 * \param ctx cipher's context. Must have been initialised. 00369 * 00370 * \return If IV has not been set yet: (recommended) IV size 00371 * (0 for ciphers not using IV/NONCE). 00372 * If IV has already been set: actual size. 00373 */ 00374 static inline int mbedtls_cipher_get_iv_size( const mbedtls_cipher_context_t *ctx ) 00375 { 00376 if( NULL == ctx || NULL == ctx->cipher_info ) 00377 return 0; 00378 00379 if( ctx->iv_size != 0 ) 00380 return (int) ctx->iv_size; 00381 00382 return (int) ctx->cipher_info->iv_size; 00383 } 00384 00385 /** 00386 * \brief Returns the type of the given cipher. 00387 * 00388 * \param ctx cipher's context. Must have been initialised. 00389 * 00390 * \return type of the cipher, or MBEDTLS_CIPHER_NONE if ctx has 00391 * not been initialised. 00392 */ 00393 static inline mbedtls_cipher_type_t mbedtls_cipher_get_type( const mbedtls_cipher_context_t *ctx ) 00394 { 00395 if( NULL == ctx || NULL == ctx->cipher_info ) 00396 return MBEDTLS_CIPHER_NONE; 00397 00398 return ctx->cipher_info->type; 00399 } 00400 00401 /** 00402 * \brief Returns the name of the given cipher, as a string. 00403 * 00404 * \param ctx cipher's context. Must have been initialised. 00405 * 00406 * \return name of the cipher, or NULL if ctx was not initialised. 00407 */ 00408 static inline const char *mbedtls_cipher_get_name( const mbedtls_cipher_context_t *ctx ) 00409 { 00410 if( NULL == ctx || NULL == ctx->cipher_info ) 00411 return 0; 00412 00413 return ctx->cipher_info->name; 00414 } 00415 00416 /** 00417 * \brief Returns the key length of the cipher. 00418 * 00419 * \param ctx cipher's context. Must have been initialised. 00420 * 00421 * \return cipher's key length, in bits, or 00422 * MBEDTLS_KEY_LENGTH_NONE if ctx has not been 00423 * initialised. 00424 */ 00425 static inline int mbedtls_cipher_get_key_bitlen( const mbedtls_cipher_context_t *ctx ) 00426 { 00427 if( NULL == ctx || NULL == ctx->cipher_info ) 00428 return MBEDTLS_KEY_LENGTH_NONE; 00429 00430 return (int) ctx->cipher_info->key_bitlen; 00431 } 00432 00433 /** 00434 * \brief Returns the operation of the given cipher. 00435 * 00436 * \param ctx cipher's context. Must have been initialised. 00437 * 00438 * \return operation (MBEDTLS_ENCRYPT or MBEDTLS_DECRYPT), 00439 * or MBEDTLS_OPERATION_NONE if ctx has not been 00440 * initialised. 00441 */ 00442 static inline mbedtls_operation_t mbedtls_cipher_get_operation( const mbedtls_cipher_context_t *ctx ) 00443 { 00444 if( NULL == ctx || NULL == ctx->cipher_info ) 00445 return MBEDTLS_OPERATION_NONE; 00446 00447 return ctx->operation; 00448 } 00449 00450 /** 00451 * \brief Set the key to use with the given context. 00452 * 00453 * \param ctx generic cipher context. May not be NULL. Must have been 00454 * initialised using cipher_context_from_type or 00455 * cipher_context_from_string. 00456 * \param key The key to use. 00457 * \param key_bitlen key length to use, in bits. 00458 * \param operation Operation that the key will be used for, either 00459 * MBEDTLS_ENCRYPT or MBEDTLS_DECRYPT. 00460 * 00461 * \returns 0 on success, MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA if 00462 * parameter verification fails or a cipher specific 00463 * error code. 00464 */ 00465 int mbedtls_cipher_setkey( mbedtls_cipher_context_t *ctx, const unsigned char *key, 00466 int key_bitlen, const mbedtls_operation_t operation ); 00467 00468 #if defined(MBEDTLS_CIPHER_MODE_WITH_PADDING) 00469 /** 00470 * \brief Set padding mode, for cipher modes that use padding. 00471 * (Default: PKCS7 padding.) 00472 * 00473 * \param ctx generic cipher context 00474 * \param mode padding mode 00475 * 00476 * \returns 0 on success, MBEDTLS_ERR_CIPHER_FEATURE_UNAVAILABLE 00477 * if selected padding mode is not supported, or 00478 * MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA if the cipher mode 00479 * does not support padding. 00480 */ 00481 int mbedtls_cipher_set_padding_mode( mbedtls_cipher_context_t *ctx, mbedtls_cipher_padding_t mode ); 00482 #endif /* MBEDTLS_CIPHER_MODE_WITH_PADDING */ 00483 00484 /** 00485 * \brief Set the initialization vector (IV) or nonce 00486 * 00487 * \param ctx generic cipher context 00488 * \param iv IV to use (or NONCE_COUNTER for CTR-mode ciphers) 00489 * \param iv_len IV length for ciphers with variable-size IV; 00490 * discarded by ciphers with fixed-size IV. 00491 * 00492 * \returns 0 on success, or MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA 00493 * 00494 * \note Some ciphers don't use IVs nor NONCE. For these 00495 * ciphers, this function has no effect. 00496 */ 00497 int mbedtls_cipher_set_iv( mbedtls_cipher_context_t *ctx, 00498 const unsigned char *iv, size_t iv_len ); 00499 00500 /** 00501 * \brief Finish preparation of the given context 00502 * 00503 * \param ctx generic cipher context 00504 * 00505 * \returns 0 on success, MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA 00506 * if parameter verification fails. 00507 */ 00508 int mbedtls_cipher_reset( mbedtls_cipher_context_t *ctx ); 00509 00510 #if defined(MBEDTLS_GCM_C) 00511 /** 00512 * \brief Add additional data (for AEAD ciphers). 00513 * Currently only supported with GCM. 00514 * Must be called exactly once, after mbedtls_cipher_reset(). 00515 * 00516 * \param ctx generic cipher context 00517 * \param ad Additional data to use. 00518 * \param ad_len Length of ad. 00519 * 00520 * \return 0 on success, or a specific error code. 00521 */ 00522 int mbedtls_cipher_update_ad( mbedtls_cipher_context_t *ctx, 00523 const unsigned char *ad, size_t ad_len ); 00524 #endif /* MBEDTLS_GCM_C */ 00525 00526 /** 00527 * \brief Generic cipher update function. Encrypts/decrypts 00528 * using the given cipher context. Writes as many block 00529 * size'd blocks of data as possible to output. Any data 00530 * that cannot be written immediately will either be added 00531 * to the next block, or flushed when cipher_final is 00532 * called. 00533 * Exception: for MBEDTLS_MODE_ECB, expects single block 00534 * in size (e.g. 16 bytes for AES) 00535 * 00536 * \param ctx generic cipher context 00537 * \param input buffer holding the input data 00538 * \param ilen length of the input data 00539 * \param output buffer for the output data. Should be able to hold at 00540 * least ilen + block_size. Cannot be the same buffer as 00541 * input! 00542 * \param olen length of the output data, will be filled with the 00543 * actual number of bytes written. 00544 * 00545 * \returns 0 on success, MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA if 00546 * parameter verification fails, 00547 * MBEDTLS_ERR_CIPHER_FEATURE_UNAVAILABLE on an 00548 * unsupported mode for a cipher or a cipher specific 00549 * error code. 00550 * 00551 * \note If the underlying cipher is GCM, all calls to this 00552 * function, except the last one before mbedtls_cipher_finish(), 00553 * must have ilen a multiple of the block size. 00554 */ 00555 int mbedtls_cipher_update( mbedtls_cipher_context_t *ctx, const unsigned char *input, 00556 size_t ilen, unsigned char *output, size_t *olen ); 00557 00558 /** 00559 * \brief Generic cipher finalisation function. If data still 00560 * needs to be flushed from an incomplete block, data 00561 * contained within it will be padded with the size of 00562 * the last block, and written to the output buffer. 00563 * 00564 * \param ctx Generic cipher context 00565 * \param output buffer to write data to. Needs block_size available. 00566 * \param olen length of the data written to the output buffer. 00567 * 00568 * \returns 0 on success, MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA if 00569 * parameter verification fails, 00570 * MBEDTLS_ERR_CIPHER_FULL_BLOCK_EXPECTED if decryption 00571 * expected a full block but was not provided one, 00572 * MBEDTLS_ERR_CIPHER_INVALID_PADDING on invalid padding 00573 * while decrypting or a cipher specific error code. 00574 */ 00575 int mbedtls_cipher_finish( mbedtls_cipher_context_t *ctx, 00576 unsigned char *output, size_t *olen ); 00577 00578 #if defined(MBEDTLS_GCM_C) 00579 /** 00580 * \brief Write tag for AEAD ciphers. 00581 * Currently only supported with GCM. 00582 * Must be called after mbedtls_cipher_finish(). 00583 * 00584 * \param ctx Generic cipher context 00585 * \param tag buffer to write the tag 00586 * \param tag_len Length of the tag to write 00587 * 00588 * \return 0 on success, or a specific error code. 00589 */ 00590 int mbedtls_cipher_write_tag( mbedtls_cipher_context_t *ctx, 00591 unsigned char *tag, size_t tag_len ); 00592 00593 /** 00594 * \brief Check tag for AEAD ciphers. 00595 * Currently only supported with GCM. 00596 * Must be called after mbedtls_cipher_finish(). 00597 * 00598 * \param ctx Generic cipher context 00599 * \param tag Buffer holding the tag 00600 * \param tag_len Length of the tag to check 00601 * 00602 * \return 0 on success, or a specific error code. 00603 */ 00604 int mbedtls_cipher_check_tag( mbedtls_cipher_context_t *ctx, 00605 const unsigned char *tag, size_t tag_len ); 00606 #endif /* MBEDTLS_GCM_C */ 00607 00608 /** 00609 * \brief Generic all-in-one encryption/decryption 00610 * (for all ciphers except AEAD constructs). 00611 * 00612 * \param ctx generic cipher context 00613 * \param iv IV to use (or NONCE_COUNTER for CTR-mode ciphers) 00614 * \param iv_len IV length for ciphers with variable-size IV; 00615 * discarded by ciphers with fixed-size IV. 00616 * \param input buffer holding the input data 00617 * \param ilen length of the input data 00618 * \param output buffer for the output data. Should be able to hold at 00619 * least ilen + block_size. Cannot be the same buffer as 00620 * input! 00621 * \param olen length of the output data, will be filled with the 00622 * actual number of bytes written. 00623 * 00624 * \note Some ciphers don't use IVs nor NONCE. For these 00625 * ciphers, use iv = NULL and iv_len = 0. 00626 * 00627 * \returns 0 on success, or 00628 * MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA, or 00629 * MBEDTLS_ERR_CIPHER_FULL_BLOCK_EXPECTED if decryption 00630 * expected a full block but was not provided one, or 00631 * MBEDTLS_ERR_CIPHER_INVALID_PADDING on invalid padding 00632 * while decrypting, or 00633 * a cipher specific error code. 00634 */ 00635 int mbedtls_cipher_crypt( mbedtls_cipher_context_t *ctx, 00636 const unsigned char *iv, size_t iv_len, 00637 const unsigned char *input, size_t ilen, 00638 unsigned char *output, size_t *olen ); 00639 00640 #if defined(MBEDTLS_CIPHER_MODE_AEAD) 00641 /** 00642 * \brief Generic autenticated encryption (AEAD ciphers). 00643 * 00644 * \param ctx generic cipher context 00645 * \param iv IV to use (or NONCE_COUNTER for CTR-mode ciphers) 00646 * \param iv_len IV length for ciphers with variable-size IV; 00647 * discarded by ciphers with fixed-size IV. 00648 * \param ad Additional data to authenticate. 00649 * \param ad_len Length of ad. 00650 * \param input buffer holding the input data 00651 * \param ilen length of the input data 00652 * \param output buffer for the output data. 00653 * Should be able to hold at least ilen. 00654 * \param olen length of the output data, will be filled with the 00655 * actual number of bytes written. 00656 * \param tag buffer for the authentication tag 00657 * \param tag_len desired tag length 00658 * 00659 * \returns 0 on success, or 00660 * MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA, or 00661 * a cipher specific error code. 00662 */ 00663 int mbedtls_cipher_auth_encrypt( mbedtls_cipher_context_t *ctx, 00664 const unsigned char *iv, size_t iv_len, 00665 const unsigned char *ad, size_t ad_len, 00666 const unsigned char *input, size_t ilen, 00667 unsigned char *output, size_t *olen, 00668 unsigned char *tag, size_t tag_len ); 00669 00670 /** 00671 * \brief Generic autenticated decryption (AEAD ciphers). 00672 * 00673 * \param ctx generic cipher context 00674 * \param iv IV to use (or NONCE_COUNTER for CTR-mode ciphers) 00675 * \param iv_len IV length for ciphers with variable-size IV; 00676 * discarded by ciphers with fixed-size IV. 00677 * \param ad Additional data to be authenticated. 00678 * \param ad_len Length of ad. 00679 * \param input buffer holding the input data 00680 * \param ilen length of the input data 00681 * \param output buffer for the output data. 00682 * Should be able to hold at least ilen. 00683 * \param olen length of the output data, will be filled with the 00684 * actual number of bytes written. 00685 * \param tag buffer holding the authentication tag 00686 * \param tag_len length of the authentication tag 00687 * 00688 * \returns 0 on success, or 00689 * MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA, or 00690 * MBEDTLS_ERR_CIPHER_AUTH_FAILED if data isn't authentic, 00691 * or a cipher specific error code. 00692 * 00693 * \note If the data is not authentic, then the output buffer 00694 * is zeroed out to prevent the unauthentic plaintext to 00695 * be used by mistake, making this interface safer. 00696 */ 00697 int mbedtls_cipher_auth_decrypt( mbedtls_cipher_context_t *ctx, 00698 const unsigned char *iv, size_t iv_len, 00699 const unsigned char *ad, size_t ad_len, 00700 const unsigned char *input, size_t ilen, 00701 unsigned char *output, size_t *olen, 00702 const unsigned char *tag, size_t tag_len ); 00703 #endif /* MBEDTLS_CIPHER_MODE_AEAD */ 00704 00705 #ifdef __cplusplus 00706 } 00707 #endif 00708 00709 #endif /* MBEDTLS_CIPHER_H */
Generated on Sun Jul 17 2022 08:25:21 by
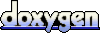