
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
cfstore_list.h
Go to the documentation of this file.
00001 /** @file cfstore_list.h 00002 * 00003 * mbed Microcontroller Library 00004 * Copyright (c) 2006-2016 ARM Limited 00005 * 00006 * Licensed under the Apache License, Version 2.0 (the "License"); 00007 * you may not use this file except in compliance with the License. 00008 * You may obtain a copy of the License at 00009 * 00010 * http://www.apache.org/licenses/LICENSE-2.0 00011 * 00012 * Unless required by applicable law or agreed to in writing, software 00013 * distributed under the License is distributed on an "AS IS" BASIS, 00014 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 * See the License for the specific language governing permissions and 00016 * limitations under the License. 00017 */ 00018 #ifndef _CFSTORE_LIST_H_ 00019 #define _CFSTORE_LIST_H_ 00020 00021 #include <stddef.h> 00022 00023 /* 00024 * Doubly linked list implementation based on the following design 00025 * and psuedo-code: 00026 * Introduction to Algorithms, TH Cormen, CE Leiserson, Rl Rivest, 00027 * ISBN 0-262-03141-8 (1989), Pages 206-207. 00028 */ 00029 typedef struct cfstore_list_node_t 00030 { 00031 struct cfstore_list_node_t *next; 00032 struct cfstore_list_node_t *prev; 00033 } cfstore_list_node_t; 00034 00035 00036 #define CFSTORE_ZERO_NODE(_node_cFStOrE) \ 00037 do{ \ 00038 (_node_cFStOrE)->next=NULL; \ 00039 (_node_cFStOrE)->prev=NULL; \ 00040 }while(0) 00041 00042 #define CFSTORE_INIT_LIST_HEAD(_node_cFStOrE) \ 00043 do { \ 00044 (_node_cFStOrE)->next = (_node_cFStOrE); \ 00045 (_node_cFStOrE)->prev = (_node_cFStOrE); \ 00046 } while (0) 00047 00048 /* brief insert the new_node between 2 other nodes, the one before being node_before, the one after being node_after */ 00049 static inline void cfstore_listAdd(cfstore_list_node_t* node_before, cfstore_list_node_t * new_node, cfstore_list_node_t* node_after) 00050 { 00051 /* init new node before insertion */ 00052 new_node->next = node_after; 00053 new_node->prev = node_before; 00054 node_before->next = new_node; 00055 node_after->prev = new_node; 00056 } 00057 00058 /* brief remove the node D from the list by making the nodes before and after D point to each other */ 00059 static inline void cfstore_listDel(cfstore_list_node_t *node) 00060 { 00061 node->prev->next = node->next; 00062 node->next->prev = node->prev; 00063 CFSTORE_ZERO_NODE(node); 00064 } 00065 #endif /* _CFSTORE_LIST_H_ */
Generated on Sun Jul 17 2022 08:25:21 by
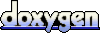