
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ccm.h
Go to the documentation of this file.
00001 /** 00002 * \file ccm.h 00003 * 00004 * \brief Counter with CBC-MAC (CCM) for 128-bit block ciphers 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_CCM_H 00024 #define MBEDTLS_CCM_H 00025 00026 #include "cipher.h" 00027 00028 #define MBEDTLS_ERR_CCM_BAD_INPUT -0x000D /**< Bad input parameters to function. */ 00029 #define MBEDTLS_ERR_CCM_AUTH_FAILED -0x000F /**< Authenticated decryption failed. */ 00030 00031 #ifdef __cplusplus 00032 extern "C" { 00033 #endif 00034 00035 /** 00036 * \brief CCM context structure 00037 */ 00038 typedef struct { 00039 mbedtls_cipher_context_t cipher_ctx ; /*!< cipher context used */ 00040 } 00041 mbedtls_ccm_context; 00042 00043 /** 00044 * \brief Initialize CCM context (just makes references valid) 00045 * Makes the context ready for mbedtls_ccm_setkey() or 00046 * mbedtls_ccm_free(). 00047 * 00048 * \param ctx CCM context to initialize 00049 */ 00050 void mbedtls_ccm_init( mbedtls_ccm_context *ctx ); 00051 00052 /** 00053 * \brief CCM initialization (encryption and decryption) 00054 * 00055 * \param ctx CCM context to be initialized 00056 * \param cipher cipher to use (a 128-bit block cipher) 00057 * \param key encryption key 00058 * \param keybits key size in bits (must be acceptable by the cipher) 00059 * 00060 * \return 0 if successful, or a cipher specific error code 00061 */ 00062 int mbedtls_ccm_setkey( mbedtls_ccm_context *ctx, 00063 mbedtls_cipher_id_t cipher, 00064 const unsigned char *key, 00065 unsigned int keybits ); 00066 00067 /** 00068 * \brief Free a CCM context and underlying cipher sub-context 00069 * 00070 * \param ctx CCM context to free 00071 */ 00072 void mbedtls_ccm_free( mbedtls_ccm_context *ctx ); 00073 00074 /** 00075 * \brief CCM buffer encryption 00076 * 00077 * \param ctx CCM context 00078 * \param length length of the input data in bytes 00079 * \param iv nonce (initialization vector) 00080 * \param iv_len length of IV in bytes 00081 * must be 2, 3, 4, 5, 6, 7 or 8 00082 * \param add additional data 00083 * \param add_len length of additional data in bytes 00084 * must be less than 2^16 - 2^8 00085 * \param input buffer holding the input data 00086 * \param output buffer for holding the output data 00087 * must be at least 'length' bytes wide 00088 * \param tag buffer for holding the tag 00089 * \param tag_len length of the tag to generate in bytes 00090 * must be 4, 6, 8, 10, 14 or 16 00091 * 00092 * \note The tag is written to a separate buffer. To get the tag 00093 * concatenated with the output as in the CCM spec, use 00094 * tag = output + length and make sure the output buffer is 00095 * at least length + tag_len wide. 00096 * 00097 * \return 0 if successful 00098 */ 00099 int mbedtls_ccm_encrypt_and_tag( mbedtls_ccm_context *ctx, size_t length, 00100 const unsigned char *iv, size_t iv_len, 00101 const unsigned char *add, size_t add_len, 00102 const unsigned char *input, unsigned char *output, 00103 unsigned char *tag, size_t tag_len ); 00104 00105 /** 00106 * \brief CCM buffer authenticated decryption 00107 * 00108 * \param ctx CCM context 00109 * \param length length of the input data 00110 * \param iv initialization vector 00111 * \param iv_len length of IV 00112 * \param add additional data 00113 * \param add_len length of additional data 00114 * \param input buffer holding the input data 00115 * \param output buffer for holding the output data 00116 * \param tag buffer holding the tag 00117 * \param tag_len length of the tag 00118 * 00119 * \return 0 if successful and authenticated, 00120 * MBEDTLS_ERR_CCM_AUTH_FAILED if tag does not match 00121 */ 00122 int mbedtls_ccm_auth_decrypt( mbedtls_ccm_context *ctx, size_t length, 00123 const unsigned char *iv, size_t iv_len, 00124 const unsigned char *add, size_t add_len, 00125 const unsigned char *input, unsigned char *output, 00126 const unsigned char *tag, size_t tag_len ); 00127 00128 #if defined(MBEDTLS_SELF_TEST) && defined(MBEDTLS_AES_C) 00129 /** 00130 * \brief Checkup routine 00131 * 00132 * \return 0 if successful, or 1 if the test failed 00133 */ 00134 int mbedtls_ccm_self_test( int verbose ); 00135 #endif /* MBEDTLS_SELF_TEST && MBEDTLS_AES_C */ 00136 00137 #ifdef __cplusplus 00138 } 00139 #endif 00140 00141 #endif /* MBEDTLS_CCM_H */
Generated on Sun Jul 17 2022 08:25:20 by
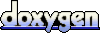