
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
attributes.c
00001 #include "mbed_toolchain.h" 00002 00003 #include <stdio.h> 00004 #include <stdint.h> 00005 00006 00007 MBED_PACKED(struct) TestAttrPackedStruct1 { 00008 char a; 00009 int x; 00010 }; 00011 00012 typedef MBED_PACKED(struct) { 00013 char a; 00014 int x; 00015 } TestAttrPackedStruct2; 00016 00017 int testPacked() { 00018 int failed = 0; 00019 00020 if (sizeof(struct TestAttrPackedStruct1) != sizeof(int) + sizeof(char)) { 00021 failed++; 00022 } 00023 00024 if (sizeof(TestAttrPackedStruct2) != sizeof(int) + sizeof(char)) { 00025 failed++; 00026 } 00027 00028 return failed; 00029 } 00030 00031 00032 MBED_ALIGN(8) char a; 00033 MBED_ALIGN(8) char b; 00034 MBED_ALIGN(16) char c; 00035 MBED_ALIGN(8) char d; 00036 MBED_ALIGN(16) char e; 00037 00038 int testAlign() { 00039 int failed = 0; 00040 00041 if(((uintptr_t)&a) & 0x7){ 00042 failed++; 00043 } 00044 if(((uintptr_t)&b) & 0x7){ 00045 failed++; 00046 } 00047 if(((uintptr_t)&c) & 0xf){ 00048 failed++; 00049 } 00050 if(((uintptr_t)&d) & 0x7){ 00051 failed++; 00052 } 00053 if(((uintptr_t)&e) & 0xf){ 00054 failed++; 00055 } 00056 00057 return failed; 00058 } 00059 00060 00061 int testUnused1(MBED_UNUSED int arg) { 00062 return 0; 00063 } 00064 00065 int testUnused() { 00066 return testUnused1(0); 00067 } 00068 00069 00070 int testWeak1(); 00071 int testWeak2(); 00072 00073 MBED_WEAK int testWeak1() { 00074 return 1; 00075 } 00076 00077 int testWeak2() { 00078 return 0; 00079 } 00080 00081 int testWeak() { 00082 return testWeak1() | testWeak2(); 00083 } 00084 00085 00086 MBED_PURE int testPure1() { 00087 return 0; 00088 } 00089 00090 int testPure() { 00091 return testPure1(); 00092 } 00093 00094 00095 MBED_FORCEINLINE int testForceInline1() { 00096 return 0; 00097 } 00098 00099 int testForceInline() { 00100 return testForceInline1(); 00101 } 00102 00103 00104 MBED_NORETURN int testNoReturn1() { 00105 while (1) {} 00106 } 00107 00108 int testNoReturn() { 00109 if (0) { 00110 testNoReturn1(); 00111 } 00112 return 0; 00113 } 00114 00115 00116 int testUnreachable1(int i) { 00117 switch (i) { 00118 case 0: 00119 return 0; 00120 } 00121 00122 MBED_UNREACHABLE; 00123 } 00124 00125 int testUnreachable() { 00126 return testUnreachable1(0); 00127 } 00128 00129 00130 MBED_DEPRECATED("this message should not be displayed") 00131 void testDeprecatedUnused(); 00132 void testDeprecatedUnused() { } 00133 00134 MBED_DEPRECATED("this message should be displayed") 00135 int testDeprecatedUsed(); 00136 int testDeprecatedUsed() { 00137 return 0; 00138 } 00139 00140 MBED_DEPRECATED_SINCE("mbed-os-3.14", "this message should not be displayed") 00141 void testDeprecatedSinceUnused(); 00142 void testDeprecatedSinceUnused() { } 00143 00144 MBED_DEPRECATED_SINCE("mbed-os-3.14", "this message should be displayed") 00145 int testDeprecatedSinceUsed(); 00146 int testDeprecatedSinceUsed() { 00147 return 0; 00148 } 00149 00150 int testDeprecated() { 00151 return testDeprecatedUsed() + testDeprecatedSinceUsed(); 00152 } 00153
Generated on Sun Jul 17 2022 08:25:20 by
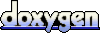