
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
aes.c
00001 /* 00002 * FIPS-197 compliant AES implementation 00003 * 00004 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00008 * not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00015 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 * 00019 * This file is part of mbed TLS (https://tls.mbed.org) 00020 */ 00021 /* 00022 * The AES block cipher was designed by Vincent Rijmen and Joan Daemen. 00023 * 00024 * http://csrc.nist.gov/encryption/aes/rijndael/Rijndael.pdf 00025 * http://csrc.nist.gov/publications/fips/fips197/fips-197.pdf 00026 */ 00027 00028 #if !defined(MBEDTLS_CONFIG_FILE) 00029 #include "mbedtls/config.h" 00030 #else 00031 #include MBEDTLS_CONFIG_FILE 00032 #endif 00033 00034 #if defined(MBEDTLS_AES_C) 00035 00036 #include <string.h> 00037 00038 #include "mbedtls/aes.h" 00039 #if defined(MBEDTLS_PADLOCK_C) 00040 #include "mbedtls/padlock.h" 00041 #endif 00042 #if defined(MBEDTLS_AESNI_C) 00043 #include "mbedtls/aesni.h" 00044 #endif 00045 00046 #if defined(MBEDTLS_SELF_TEST) 00047 #if defined(MBEDTLS_PLATFORM_C) 00048 #include "mbedtls/platform.h" 00049 #else 00050 #include <stdio.h> 00051 #define mbedtls_printf printf 00052 #endif /* MBEDTLS_PLATFORM_C */ 00053 #endif /* MBEDTLS_SELF_TEST */ 00054 00055 #if !defined(MBEDTLS_AES_ALT) 00056 00057 /* Implementation that should never be optimized out by the compiler */ 00058 static void mbedtls_zeroize( void *v, size_t n ) { 00059 volatile unsigned char *p = (unsigned char*)v; while( n-- ) *p++ = 0; 00060 } 00061 00062 /* 00063 * 32-bit integer manipulation macros (little endian) 00064 */ 00065 #ifndef GET_UINT32_LE 00066 #define GET_UINT32_LE(n,b,i) \ 00067 { \ 00068 (n) = ( (uint32_t) (b)[(i) ] ) \ 00069 | ( (uint32_t) (b)[(i) + 1] << 8 ) \ 00070 | ( (uint32_t) (b)[(i) + 2] << 16 ) \ 00071 | ( (uint32_t) (b)[(i) + 3] << 24 ); \ 00072 } 00073 #endif 00074 00075 #ifndef PUT_UINT32_LE 00076 #define PUT_UINT32_LE(n,b,i) \ 00077 { \ 00078 (b)[(i) ] = (unsigned char) ( ( (n) ) & 0xFF ); \ 00079 (b)[(i) + 1] = (unsigned char) ( ( (n) >> 8 ) & 0xFF ); \ 00080 (b)[(i) + 2] = (unsigned char) ( ( (n) >> 16 ) & 0xFF ); \ 00081 (b)[(i) + 3] = (unsigned char) ( ( (n) >> 24 ) & 0xFF ); \ 00082 } 00083 #endif 00084 00085 #if defined(MBEDTLS_PADLOCK_C) && \ 00086 ( defined(MBEDTLS_HAVE_X86) || defined(MBEDTLS_PADLOCK_ALIGN16) ) 00087 static int aes_padlock_ace = -1; 00088 #endif 00089 00090 #if defined(MBEDTLS_AES_ROM_TABLES) 00091 /* 00092 * Forward S-box 00093 */ 00094 static const unsigned char FSb[256] = 00095 { 00096 0x63, 0x7C, 0x77, 0x7B, 0xF2, 0x6B, 0x6F, 0xC5, 00097 0x30, 0x01, 0x67, 0x2B, 0xFE, 0xD7, 0xAB, 0x76, 00098 0xCA, 0x82, 0xC9, 0x7D, 0xFA, 0x59, 0x47, 0xF0, 00099 0xAD, 0xD4, 0xA2, 0xAF, 0x9C, 0xA4, 0x72, 0xC0, 00100 0xB7, 0xFD, 0x93, 0x26, 0x36, 0x3F, 0xF7, 0xCC, 00101 0x34, 0xA5, 0xE5, 0xF1, 0x71, 0xD8, 0x31, 0x15, 00102 0x04, 0xC7, 0x23, 0xC3, 0x18, 0x96, 0x05, 0x9A, 00103 0x07, 0x12, 0x80, 0xE2, 0xEB, 0x27, 0xB2, 0x75, 00104 0x09, 0x83, 0x2C, 0x1A, 0x1B, 0x6E, 0x5A, 0xA0, 00105 0x52, 0x3B, 0xD6, 0xB3, 0x29, 0xE3, 0x2F, 0x84, 00106 0x53, 0xD1, 0x00, 0xED, 0x20, 0xFC, 0xB1, 0x5B, 00107 0x6A, 0xCB, 0xBE, 0x39, 0x4A, 0x4C, 0x58, 0xCF, 00108 0xD0, 0xEF, 0xAA, 0xFB, 0x43, 0x4D, 0x33, 0x85, 00109 0x45, 0xF9, 0x02, 0x7F, 0x50, 0x3C, 0x9F, 0xA8, 00110 0x51, 0xA3, 0x40, 0x8F, 0x92, 0x9D, 0x38, 0xF5, 00111 0xBC, 0xB6, 0xDA, 0x21, 0x10, 0xFF, 0xF3, 0xD2, 00112 0xCD, 0x0C, 0x13, 0xEC, 0x5F, 0x97, 0x44, 0x17, 00113 0xC4, 0xA7, 0x7E, 0x3D, 0x64, 0x5D, 0x19, 0x73, 00114 0x60, 0x81, 0x4F, 0xDC, 0x22, 0x2A, 0x90, 0x88, 00115 0x46, 0xEE, 0xB8, 0x14, 0xDE, 0x5E, 0x0B, 0xDB, 00116 0xE0, 0x32, 0x3A, 0x0A, 0x49, 0x06, 0x24, 0x5C, 00117 0xC2, 0xD3, 0xAC, 0x62, 0x91, 0x95, 0xE4, 0x79, 00118 0xE7, 0xC8, 0x37, 0x6D, 0x8D, 0xD5, 0x4E, 0xA9, 00119 0x6C, 0x56, 0xF4, 0xEA, 0x65, 0x7A, 0xAE, 0x08, 00120 0xBA, 0x78, 0x25, 0x2E, 0x1C, 0xA6, 0xB4, 0xC6, 00121 0xE8, 0xDD, 0x74, 0x1F, 0x4B, 0xBD, 0x8B, 0x8A, 00122 0x70, 0x3E, 0xB5, 0x66, 0x48, 0x03, 0xF6, 0x0E, 00123 0x61, 0x35, 0x57, 0xB9, 0x86, 0xC1, 0x1D, 0x9E, 00124 0xE1, 0xF8, 0x98, 0x11, 0x69, 0xD9, 0x8E, 0x94, 00125 0x9B, 0x1E, 0x87, 0xE9, 0xCE, 0x55, 0x28, 0xDF, 00126 0x8C, 0xA1, 0x89, 0x0D, 0xBF, 0xE6, 0x42, 0x68, 00127 0x41, 0x99, 0x2D, 0x0F, 0xB0, 0x54, 0xBB, 0x16 00128 }; 00129 00130 /* 00131 * Forward tables 00132 */ 00133 #define FT \ 00134 \ 00135 V(A5,63,63,C6), V(84,7C,7C,F8), V(99,77,77,EE), V(8D,7B,7B,F6), \ 00136 V(0D,F2,F2,FF), V(BD,6B,6B,D6), V(B1,6F,6F,DE), V(54,C5,C5,91), \ 00137 V(50,30,30,60), V(03,01,01,02), V(A9,67,67,CE), V(7D,2B,2B,56), \ 00138 V(19,FE,FE,E7), V(62,D7,D7,B5), V(E6,AB,AB,4D), V(9A,76,76,EC), \ 00139 V(45,CA,CA,8F), V(9D,82,82,1F), V(40,C9,C9,89), V(87,7D,7D,FA), \ 00140 V(15,FA,FA,EF), V(EB,59,59,B2), V(C9,47,47,8E), V(0B,F0,F0,FB), \ 00141 V(EC,AD,AD,41), V(67,D4,D4,B3), V(FD,A2,A2,5F), V(EA,AF,AF,45), \ 00142 V(BF,9C,9C,23), V(F7,A4,A4,53), V(96,72,72,E4), V(5B,C0,C0,9B), \ 00143 V(C2,B7,B7,75), V(1C,FD,FD,E1), V(AE,93,93,3D), V(6A,26,26,4C), \ 00144 V(5A,36,36,6C), V(41,3F,3F,7E), V(02,F7,F7,F5), V(4F,CC,CC,83), \ 00145 V(5C,34,34,68), V(F4,A5,A5,51), V(34,E5,E5,D1), V(08,F1,F1,F9), \ 00146 V(93,71,71,E2), V(73,D8,D8,AB), V(53,31,31,62), V(3F,15,15,2A), \ 00147 V(0C,04,04,08), V(52,C7,C7,95), V(65,23,23,46), V(5E,C3,C3,9D), \ 00148 V(28,18,18,30), V(A1,96,96,37), V(0F,05,05,0A), V(B5,9A,9A,2F), \ 00149 V(09,07,07,0E), V(36,12,12,24), V(9B,80,80,1B), V(3D,E2,E2,DF), \ 00150 V(26,EB,EB,CD), V(69,27,27,4E), V(CD,B2,B2,7F), V(9F,75,75,EA), \ 00151 V(1B,09,09,12), V(9E,83,83,1D), V(74,2C,2C,58), V(2E,1A,1A,34), \ 00152 V(2D,1B,1B,36), V(B2,6E,6E,DC), V(EE,5A,5A,B4), V(FB,A0,A0,5B), \ 00153 V(F6,52,52,A4), V(4D,3B,3B,76), V(61,D6,D6,B7), V(CE,B3,B3,7D), \ 00154 V(7B,29,29,52), V(3E,E3,E3,DD), V(71,2F,2F,5E), V(97,84,84,13), \ 00155 V(F5,53,53,A6), V(68,D1,D1,B9), V(00,00,00,00), V(2C,ED,ED,C1), \ 00156 V(60,20,20,40), V(1F,FC,FC,E3), V(C8,B1,B1,79), V(ED,5B,5B,B6), \ 00157 V(BE,6A,6A,D4), V(46,CB,CB,8D), V(D9,BE,BE,67), V(4B,39,39,72), \ 00158 V(DE,4A,4A,94), V(D4,4C,4C,98), V(E8,58,58,B0), V(4A,CF,CF,85), \ 00159 V(6B,D0,D0,BB), V(2A,EF,EF,C5), V(E5,AA,AA,4F), V(16,FB,FB,ED), \ 00160 V(C5,43,43,86), V(D7,4D,4D,9A), V(55,33,33,66), V(94,85,85,11), \ 00161 V(CF,45,45,8A), V(10,F9,F9,E9), V(06,02,02,04), V(81,7F,7F,FE), \ 00162 V(F0,50,50,A0), V(44,3C,3C,78), V(BA,9F,9F,25), V(E3,A8,A8,4B), \ 00163 V(F3,51,51,A2), V(FE,A3,A3,5D), V(C0,40,40,80), V(8A,8F,8F,05), \ 00164 V(AD,92,92,3F), V(BC,9D,9D,21), V(48,38,38,70), V(04,F5,F5,F1), \ 00165 V(DF,BC,BC,63), V(C1,B6,B6,77), V(75,DA,DA,AF), V(63,21,21,42), \ 00166 V(30,10,10,20), V(1A,FF,FF,E5), V(0E,F3,F3,FD), V(6D,D2,D2,BF), \ 00167 V(4C,CD,CD,81), V(14,0C,0C,18), V(35,13,13,26), V(2F,EC,EC,C3), \ 00168 V(E1,5F,5F,BE), V(A2,97,97,35), V(CC,44,44,88), V(39,17,17,2E), \ 00169 V(57,C4,C4,93), V(F2,A7,A7,55), V(82,7E,7E,FC), V(47,3D,3D,7A), \ 00170 V(AC,64,64,C8), V(E7,5D,5D,BA), V(2B,19,19,32), V(95,73,73,E6), \ 00171 V(A0,60,60,C0), V(98,81,81,19), V(D1,4F,4F,9E), V(7F,DC,DC,A3), \ 00172 V(66,22,22,44), V(7E,2A,2A,54), V(AB,90,90,3B), V(83,88,88,0B), \ 00173 V(CA,46,46,8C), V(29,EE,EE,C7), V(D3,B8,B8,6B), V(3C,14,14,28), \ 00174 V(79,DE,DE,A7), V(E2,5E,5E,BC), V(1D,0B,0B,16), V(76,DB,DB,AD), \ 00175 V(3B,E0,E0,DB), V(56,32,32,64), V(4E,3A,3A,74), V(1E,0A,0A,14), \ 00176 V(DB,49,49,92), V(0A,06,06,0C), V(6C,24,24,48), V(E4,5C,5C,B8), \ 00177 V(5D,C2,C2,9F), V(6E,D3,D3,BD), V(EF,AC,AC,43), V(A6,62,62,C4), \ 00178 V(A8,91,91,39), V(A4,95,95,31), V(37,E4,E4,D3), V(8B,79,79,F2), \ 00179 V(32,E7,E7,D5), V(43,C8,C8,8B), V(59,37,37,6E), V(B7,6D,6D,DA), \ 00180 V(8C,8D,8D,01), V(64,D5,D5,B1), V(D2,4E,4E,9C), V(E0,A9,A9,49), \ 00181 V(B4,6C,6C,D8), V(FA,56,56,AC), V(07,F4,F4,F3), V(25,EA,EA,CF), \ 00182 V(AF,65,65,CA), V(8E,7A,7A,F4), V(E9,AE,AE,47), V(18,08,08,10), \ 00183 V(D5,BA,BA,6F), V(88,78,78,F0), V(6F,25,25,4A), V(72,2E,2E,5C), \ 00184 V(24,1C,1C,38), V(F1,A6,A6,57), V(C7,B4,B4,73), V(51,C6,C6,97), \ 00185 V(23,E8,E8,CB), V(7C,DD,DD,A1), V(9C,74,74,E8), V(21,1F,1F,3E), \ 00186 V(DD,4B,4B,96), V(DC,BD,BD,61), V(86,8B,8B,0D), V(85,8A,8A,0F), \ 00187 V(90,70,70,E0), V(42,3E,3E,7C), V(C4,B5,B5,71), V(AA,66,66,CC), \ 00188 V(D8,48,48,90), V(05,03,03,06), V(01,F6,F6,F7), V(12,0E,0E,1C), \ 00189 V(A3,61,61,C2), V(5F,35,35,6A), V(F9,57,57,AE), V(D0,B9,B9,69), \ 00190 V(91,86,86,17), V(58,C1,C1,99), V(27,1D,1D,3A), V(B9,9E,9E,27), \ 00191 V(38,E1,E1,D9), V(13,F8,F8,EB), V(B3,98,98,2B), V(33,11,11,22), \ 00192 V(BB,69,69,D2), V(70,D9,D9,A9), V(89,8E,8E,07), V(A7,94,94,33), \ 00193 V(B6,9B,9B,2D), V(22,1E,1E,3C), V(92,87,87,15), V(20,E9,E9,C9), \ 00194 V(49,CE,CE,87), V(FF,55,55,AA), V(78,28,28,50), V(7A,DF,DF,A5), \ 00195 V(8F,8C,8C,03), V(F8,A1,A1,59), V(80,89,89,09), V(17,0D,0D,1A), \ 00196 V(DA,BF,BF,65), V(31,E6,E6,D7), V(C6,42,42,84), V(B8,68,68,D0), \ 00197 V(C3,41,41,82), V(B0,99,99,29), V(77,2D,2D,5A), V(11,0F,0F,1E), \ 00198 V(CB,B0,B0,7B), V(FC,54,54,A8), V(D6,BB,BB,6D), V(3A,16,16,2C) 00199 00200 #define V(a,b,c,d) 0x##a##b##c##d 00201 static const uint32_t FT0[256] = { FT }; 00202 #undef V 00203 00204 #define V(a,b,c,d) 0x##b##c##d##a 00205 static const uint32_t FT1[256] = { FT }; 00206 #undef V 00207 00208 #define V(a,b,c,d) 0x##c##d##a##b 00209 static const uint32_t FT2[256] = { FT }; 00210 #undef V 00211 00212 #define V(a,b,c,d) 0x##d##a##b##c 00213 static const uint32_t FT3[256] = { FT }; 00214 #undef V 00215 00216 #undef FT 00217 00218 /* 00219 * Reverse S-box 00220 */ 00221 static const unsigned char RSb[256] = 00222 { 00223 0x52, 0x09, 0x6A, 0xD5, 0x30, 0x36, 0xA5, 0x38, 00224 0xBF, 0x40, 0xA3, 0x9E, 0x81, 0xF3, 0xD7, 0xFB, 00225 0x7C, 0xE3, 0x39, 0x82, 0x9B, 0x2F, 0xFF, 0x87, 00226 0x34, 0x8E, 0x43, 0x44, 0xC4, 0xDE, 0xE9, 0xCB, 00227 0x54, 0x7B, 0x94, 0x32, 0xA6, 0xC2, 0x23, 0x3D, 00228 0xEE, 0x4C, 0x95, 0x0B, 0x42, 0xFA, 0xC3, 0x4E, 00229 0x08, 0x2E, 0xA1, 0x66, 0x28, 0xD9, 0x24, 0xB2, 00230 0x76, 0x5B, 0xA2, 0x49, 0x6D, 0x8B, 0xD1, 0x25, 00231 0x72, 0xF8, 0xF6, 0x64, 0x86, 0x68, 0x98, 0x16, 00232 0xD4, 0xA4, 0x5C, 0xCC, 0x5D, 0x65, 0xB6, 0x92, 00233 0x6C, 0x70, 0x48, 0x50, 0xFD, 0xED, 0xB9, 0xDA, 00234 0x5E, 0x15, 0x46, 0x57, 0xA7, 0x8D, 0x9D, 0x84, 00235 0x90, 0xD8, 0xAB, 0x00, 0x8C, 0xBC, 0xD3, 0x0A, 00236 0xF7, 0xE4, 0x58, 0x05, 0xB8, 0xB3, 0x45, 0x06, 00237 0xD0, 0x2C, 0x1E, 0x8F, 0xCA, 0x3F, 0x0F, 0x02, 00238 0xC1, 0xAF, 0xBD, 0x03, 0x01, 0x13, 0x8A, 0x6B, 00239 0x3A, 0x91, 0x11, 0x41, 0x4F, 0x67, 0xDC, 0xEA, 00240 0x97, 0xF2, 0xCF, 0xCE, 0xF0, 0xB4, 0xE6, 0x73, 00241 0x96, 0xAC, 0x74, 0x22, 0xE7, 0xAD, 0x35, 0x85, 00242 0xE2, 0xF9, 0x37, 0xE8, 0x1C, 0x75, 0xDF, 0x6E, 00243 0x47, 0xF1, 0x1A, 0x71, 0x1D, 0x29, 0xC5, 0x89, 00244 0x6F, 0xB7, 0x62, 0x0E, 0xAA, 0x18, 0xBE, 0x1B, 00245 0xFC, 0x56, 0x3E, 0x4B, 0xC6, 0xD2, 0x79, 0x20, 00246 0x9A, 0xDB, 0xC0, 0xFE, 0x78, 0xCD, 0x5A, 0xF4, 00247 0x1F, 0xDD, 0xA8, 0x33, 0x88, 0x07, 0xC7, 0x31, 00248 0xB1, 0x12, 0x10, 0x59, 0x27, 0x80, 0xEC, 0x5F, 00249 0x60, 0x51, 0x7F, 0xA9, 0x19, 0xB5, 0x4A, 0x0D, 00250 0x2D, 0xE5, 0x7A, 0x9F, 0x93, 0xC9, 0x9C, 0xEF, 00251 0xA0, 0xE0, 0x3B, 0x4D, 0xAE, 0x2A, 0xF5, 0xB0, 00252 0xC8, 0xEB, 0xBB, 0x3C, 0x83, 0x53, 0x99, 0x61, 00253 0x17, 0x2B, 0x04, 0x7E, 0xBA, 0x77, 0xD6, 0x26, 00254 0xE1, 0x69, 0x14, 0x63, 0x55, 0x21, 0x0C, 0x7D 00255 }; 00256 00257 /* 00258 * Reverse tables 00259 */ 00260 #define RT \ 00261 \ 00262 V(50,A7,F4,51), V(53,65,41,7E), V(C3,A4,17,1A), V(96,5E,27,3A), \ 00263 V(CB,6B,AB,3B), V(F1,45,9D,1F), V(AB,58,FA,AC), V(93,03,E3,4B), \ 00264 V(55,FA,30,20), V(F6,6D,76,AD), V(91,76,CC,88), V(25,4C,02,F5), \ 00265 V(FC,D7,E5,4F), V(D7,CB,2A,C5), V(80,44,35,26), V(8F,A3,62,B5), \ 00266 V(49,5A,B1,DE), V(67,1B,BA,25), V(98,0E,EA,45), V(E1,C0,FE,5D), \ 00267 V(02,75,2F,C3), V(12,F0,4C,81), V(A3,97,46,8D), V(C6,F9,D3,6B), \ 00268 V(E7,5F,8F,03), V(95,9C,92,15), V(EB,7A,6D,BF), V(DA,59,52,95), \ 00269 V(2D,83,BE,D4), V(D3,21,74,58), V(29,69,E0,49), V(44,C8,C9,8E), \ 00270 V(6A,89,C2,75), V(78,79,8E,F4), V(6B,3E,58,99), V(DD,71,B9,27), \ 00271 V(B6,4F,E1,BE), V(17,AD,88,F0), V(66,AC,20,C9), V(B4,3A,CE,7D), \ 00272 V(18,4A,DF,63), V(82,31,1A,E5), V(60,33,51,97), V(45,7F,53,62), \ 00273 V(E0,77,64,B1), V(84,AE,6B,BB), V(1C,A0,81,FE), V(94,2B,08,F9), \ 00274 V(58,68,48,70), V(19,FD,45,8F), V(87,6C,DE,94), V(B7,F8,7B,52), \ 00275 V(23,D3,73,AB), V(E2,02,4B,72), V(57,8F,1F,E3), V(2A,AB,55,66), \ 00276 V(07,28,EB,B2), V(03,C2,B5,2F), V(9A,7B,C5,86), V(A5,08,37,D3), \ 00277 V(F2,87,28,30), V(B2,A5,BF,23), V(BA,6A,03,02), V(5C,82,16,ED), \ 00278 V(2B,1C,CF,8A), V(92,B4,79,A7), V(F0,F2,07,F3), V(A1,E2,69,4E), \ 00279 V(CD,F4,DA,65), V(D5,BE,05,06), V(1F,62,34,D1), V(8A,FE,A6,C4), \ 00280 V(9D,53,2E,34), V(A0,55,F3,A2), V(32,E1,8A,05), V(75,EB,F6,A4), \ 00281 V(39,EC,83,0B), V(AA,EF,60,40), V(06,9F,71,5E), V(51,10,6E,BD), \ 00282 V(F9,8A,21,3E), V(3D,06,DD,96), V(AE,05,3E,DD), V(46,BD,E6,4D), \ 00283 V(B5,8D,54,91), V(05,5D,C4,71), V(6F,D4,06,04), V(FF,15,50,60), \ 00284 V(24,FB,98,19), V(97,E9,BD,D6), V(CC,43,40,89), V(77,9E,D9,67), \ 00285 V(BD,42,E8,B0), V(88,8B,89,07), V(38,5B,19,E7), V(DB,EE,C8,79), \ 00286 V(47,0A,7C,A1), V(E9,0F,42,7C), V(C9,1E,84,F8), V(00,00,00,00), \ 00287 V(83,86,80,09), V(48,ED,2B,32), V(AC,70,11,1E), V(4E,72,5A,6C), \ 00288 V(FB,FF,0E,FD), V(56,38,85,0F), V(1E,D5,AE,3D), V(27,39,2D,36), \ 00289 V(64,D9,0F,0A), V(21,A6,5C,68), V(D1,54,5B,9B), V(3A,2E,36,24), \ 00290 V(B1,67,0A,0C), V(0F,E7,57,93), V(D2,96,EE,B4), V(9E,91,9B,1B), \ 00291 V(4F,C5,C0,80), V(A2,20,DC,61), V(69,4B,77,5A), V(16,1A,12,1C), \ 00292 V(0A,BA,93,E2), V(E5,2A,A0,C0), V(43,E0,22,3C), V(1D,17,1B,12), \ 00293 V(0B,0D,09,0E), V(AD,C7,8B,F2), V(B9,A8,B6,2D), V(C8,A9,1E,14), \ 00294 V(85,19,F1,57), V(4C,07,75,AF), V(BB,DD,99,EE), V(FD,60,7F,A3), \ 00295 V(9F,26,01,F7), V(BC,F5,72,5C), V(C5,3B,66,44), V(34,7E,FB,5B), \ 00296 V(76,29,43,8B), V(DC,C6,23,CB), V(68,FC,ED,B6), V(63,F1,E4,B8), \ 00297 V(CA,DC,31,D7), V(10,85,63,42), V(40,22,97,13), V(20,11,C6,84), \ 00298 V(7D,24,4A,85), V(F8,3D,BB,D2), V(11,32,F9,AE), V(6D,A1,29,C7), \ 00299 V(4B,2F,9E,1D), V(F3,30,B2,DC), V(EC,52,86,0D), V(D0,E3,C1,77), \ 00300 V(6C,16,B3,2B), V(99,B9,70,A9), V(FA,48,94,11), V(22,64,E9,47), \ 00301 V(C4,8C,FC,A8), V(1A,3F,F0,A0), V(D8,2C,7D,56), V(EF,90,33,22), \ 00302 V(C7,4E,49,87), V(C1,D1,38,D9), V(FE,A2,CA,8C), V(36,0B,D4,98), \ 00303 V(CF,81,F5,A6), V(28,DE,7A,A5), V(26,8E,B7,DA), V(A4,BF,AD,3F), \ 00304 V(E4,9D,3A,2C), V(0D,92,78,50), V(9B,CC,5F,6A), V(62,46,7E,54), \ 00305 V(C2,13,8D,F6), V(E8,B8,D8,90), V(5E,F7,39,2E), V(F5,AF,C3,82), \ 00306 V(BE,80,5D,9F), V(7C,93,D0,69), V(A9,2D,D5,6F), V(B3,12,25,CF), \ 00307 V(3B,99,AC,C8), V(A7,7D,18,10), V(6E,63,9C,E8), V(7B,BB,3B,DB), \ 00308 V(09,78,26,CD), V(F4,18,59,6E), V(01,B7,9A,EC), V(A8,9A,4F,83), \ 00309 V(65,6E,95,E6), V(7E,E6,FF,AA), V(08,CF,BC,21), V(E6,E8,15,EF), \ 00310 V(D9,9B,E7,BA), V(CE,36,6F,4A), V(D4,09,9F,EA), V(D6,7C,B0,29), \ 00311 V(AF,B2,A4,31), V(31,23,3F,2A), V(30,94,A5,C6), V(C0,66,A2,35), \ 00312 V(37,BC,4E,74), V(A6,CA,82,FC), V(B0,D0,90,E0), V(15,D8,A7,33), \ 00313 V(4A,98,04,F1), V(F7,DA,EC,41), V(0E,50,CD,7F), V(2F,F6,91,17), \ 00314 V(8D,D6,4D,76), V(4D,B0,EF,43), V(54,4D,AA,CC), V(DF,04,96,E4), \ 00315 V(E3,B5,D1,9E), V(1B,88,6A,4C), V(B8,1F,2C,C1), V(7F,51,65,46), \ 00316 V(04,EA,5E,9D), V(5D,35,8C,01), V(73,74,87,FA), V(2E,41,0B,FB), \ 00317 V(5A,1D,67,B3), V(52,D2,DB,92), V(33,56,10,E9), V(13,47,D6,6D), \ 00318 V(8C,61,D7,9A), V(7A,0C,A1,37), V(8E,14,F8,59), V(89,3C,13,EB), \ 00319 V(EE,27,A9,CE), V(35,C9,61,B7), V(ED,E5,1C,E1), V(3C,B1,47,7A), \ 00320 V(59,DF,D2,9C), V(3F,73,F2,55), V(79,CE,14,18), V(BF,37,C7,73), \ 00321 V(EA,CD,F7,53), V(5B,AA,FD,5F), V(14,6F,3D,DF), V(86,DB,44,78), \ 00322 V(81,F3,AF,CA), V(3E,C4,68,B9), V(2C,34,24,38), V(5F,40,A3,C2), \ 00323 V(72,C3,1D,16), V(0C,25,E2,BC), V(8B,49,3C,28), V(41,95,0D,FF), \ 00324 V(71,01,A8,39), V(DE,B3,0C,08), V(9C,E4,B4,D8), V(90,C1,56,64), \ 00325 V(61,84,CB,7B), V(70,B6,32,D5), V(74,5C,6C,48), V(42,57,B8,D0) 00326 00327 #define V(a,b,c,d) 0x##a##b##c##d 00328 static const uint32_t RT0[256] = { RT }; 00329 #undef V 00330 00331 #define V(a,b,c,d) 0x##b##c##d##a 00332 static const uint32_t RT1[256] = { RT }; 00333 #undef V 00334 00335 #define V(a,b,c,d) 0x##c##d##a##b 00336 static const uint32_t RT2[256] = { RT }; 00337 #undef V 00338 00339 #define V(a,b,c,d) 0x##d##a##b##c 00340 static const uint32_t RT3[256] = { RT }; 00341 #undef V 00342 00343 #undef RT 00344 00345 /* 00346 * Round constants 00347 */ 00348 static const uint32_t RCON[10] = 00349 { 00350 0x00000001, 0x00000002, 0x00000004, 0x00000008, 00351 0x00000010, 0x00000020, 0x00000040, 0x00000080, 00352 0x0000001B, 0x00000036 00353 }; 00354 00355 #else /* MBEDTLS_AES_ROM_TABLES */ 00356 00357 /* 00358 * Forward S-box & tables 00359 */ 00360 static unsigned char FSb[256]; 00361 static uint32_t FT0[256]; 00362 static uint32_t FT1[256]; 00363 static uint32_t FT2[256]; 00364 static uint32_t FT3[256]; 00365 00366 /* 00367 * Reverse S-box & tables 00368 */ 00369 static unsigned char RSb[256]; 00370 static uint32_t RT0[256]; 00371 static uint32_t RT1[256]; 00372 static uint32_t RT2[256]; 00373 static uint32_t RT3[256]; 00374 00375 /* 00376 * Round constants 00377 */ 00378 static uint32_t RCON[10]; 00379 00380 /* 00381 * Tables generation code 00382 */ 00383 #define ROTL8(x) ( ( x << 8 ) & 0xFFFFFFFF ) | ( x >> 24 ) 00384 #define XTIME(x) ( ( x << 1 ) ^ ( ( x & 0x80 ) ? 0x1B : 0x00 ) ) 00385 #define MUL(x,y) ( ( x && y ) ? pow[(log[x]+log[y]) % 255] : 0 ) 00386 00387 static int aes_init_done = 0; 00388 00389 static void aes_gen_tables( void ) 00390 { 00391 int i, x, y, z; 00392 int pow[256]; 00393 int log[256]; 00394 00395 /* 00396 * compute pow and log tables over GF(2^8) 00397 */ 00398 for( i = 0, x = 1; i < 256; i++ ) 00399 { 00400 pow[i] = x; 00401 log[x] = i; 00402 x = ( x ^ XTIME( x ) ) & 0xFF; 00403 } 00404 00405 /* 00406 * calculate the round constants 00407 */ 00408 for( i = 0, x = 1; i < 10; i++ ) 00409 { 00410 RCON[i] = (uint32_t) x; 00411 x = XTIME( x ) & 0xFF; 00412 } 00413 00414 /* 00415 * generate the forward and reverse S-boxes 00416 */ 00417 FSb[0x00] = 0x63; 00418 RSb[0x63] = 0x00; 00419 00420 for( i = 1; i < 256; i++ ) 00421 { 00422 x = pow[255 - log[i]]; 00423 00424 y = x; y = ( ( y << 1 ) | ( y >> 7 ) ) & 0xFF; 00425 x ^= y; y = ( ( y << 1 ) | ( y >> 7 ) ) & 0xFF; 00426 x ^= y; y = ( ( y << 1 ) | ( y >> 7 ) ) & 0xFF; 00427 x ^= y; y = ( ( y << 1 ) | ( y >> 7 ) ) & 0xFF; 00428 x ^= y ^ 0x63; 00429 00430 FSb[i] = (unsigned char) x; 00431 RSb[x] = (unsigned char) i; 00432 } 00433 00434 /* 00435 * generate the forward and reverse tables 00436 */ 00437 for( i = 0; i < 256; i++ ) 00438 { 00439 x = FSb[i]; 00440 y = XTIME( x ) & 0xFF; 00441 z = ( y ^ x ) & 0xFF; 00442 00443 FT0[i] = ( (uint32_t) y ) ^ 00444 ( (uint32_t) x << 8 ) ^ 00445 ( (uint32_t) x << 16 ) ^ 00446 ( (uint32_t) z << 24 ); 00447 00448 FT1[i] = ROTL8( FT0[i] ); 00449 FT2[i] = ROTL8( FT1[i] ); 00450 FT3[i] = ROTL8( FT2[i] ); 00451 00452 x = RSb[i]; 00453 00454 RT0[i] = ( (uint32_t) MUL( 0x0E, x ) ) ^ 00455 ( (uint32_t) MUL( 0x09, x ) << 8 ) ^ 00456 ( (uint32_t) MUL( 0x0D, x ) << 16 ) ^ 00457 ( (uint32_t) MUL( 0x0B, x ) << 24 ); 00458 00459 RT1[i] = ROTL8( RT0[i] ); 00460 RT2[i] = ROTL8( RT1[i] ); 00461 RT3[i] = ROTL8( RT2[i] ); 00462 } 00463 } 00464 00465 #endif /* MBEDTLS_AES_ROM_TABLES */ 00466 00467 void mbedtls_aes_init( mbedtls_aes_context *ctx ) 00468 { 00469 memset( ctx, 0, sizeof( mbedtls_aes_context ) ); 00470 } 00471 00472 void mbedtls_aes_free( mbedtls_aes_context *ctx ) 00473 { 00474 if( ctx == NULL ) 00475 return; 00476 00477 mbedtls_zeroize( ctx, sizeof( mbedtls_aes_context ) ); 00478 } 00479 00480 /* 00481 * AES key schedule (encryption) 00482 */ 00483 #if !defined(MBEDTLS_AES_SETKEY_ENC_ALT) 00484 int mbedtls_aes_setkey_enc( mbedtls_aes_context *ctx, const unsigned char *key, 00485 unsigned int keybits ) 00486 { 00487 unsigned int i; 00488 uint32_t *RK; 00489 00490 #if !defined(MBEDTLS_AES_ROM_TABLES) 00491 if( aes_init_done == 0 ) 00492 { 00493 aes_gen_tables(); 00494 aes_init_done = 1; 00495 00496 } 00497 #endif 00498 00499 switch( keybits ) 00500 { 00501 case 128: ctx->nr = 10; break; 00502 case 192: ctx->nr = 12; break; 00503 case 256: ctx->nr = 14; break; 00504 default : return( MBEDTLS_ERR_AES_INVALID_KEY_LENGTH ); 00505 } 00506 00507 #if defined(MBEDTLS_PADLOCK_C) && defined(MBEDTLS_PADLOCK_ALIGN16) 00508 if( aes_padlock_ace == -1 ) 00509 aes_padlock_ace = mbedtls_padlock_has_support( MBEDTLS_PADLOCK_ACE ); 00510 00511 if( aes_padlock_ace ) 00512 ctx->rk = RK = MBEDTLS_PADLOCK_ALIGN16( ctx->buf ); 00513 else 00514 #endif 00515 ctx->rk = RK = ctx->buf ; 00516 00517 #if defined(MBEDTLS_AESNI_C) && defined(MBEDTLS_HAVE_X86_64) 00518 if( mbedtls_aesni_has_support( MBEDTLS_AESNI_AES ) ) 00519 return( mbedtls_aesni_setkey_enc( (unsigned char *) ctx->rk , key, keybits ) ); 00520 #endif 00521 00522 for( i = 0; i < ( keybits >> 5 ); i++ ) 00523 { 00524 GET_UINT32_LE( RK[i], key, i << 2 ); 00525 } 00526 00527 switch( ctx->nr ) 00528 { 00529 case 10: 00530 00531 for( i = 0; i < 10; i++, RK += 4 ) 00532 { 00533 RK[4] = RK[0] ^ RCON[i] ^ 00534 ( (uint32_t) FSb[ ( RK[3] >> 8 ) & 0xFF ] ) ^ 00535 ( (uint32_t) FSb[ ( RK[3] >> 16 ) & 0xFF ] << 8 ) ^ 00536 ( (uint32_t) FSb[ ( RK[3] >> 24 ) & 0xFF ] << 16 ) ^ 00537 ( (uint32_t) FSb[ ( RK[3] ) & 0xFF ] << 24 ); 00538 00539 RK[5] = RK[1] ^ RK[4]; 00540 RK[6] = RK[2] ^ RK[5]; 00541 RK[7] = RK[3] ^ RK[6]; 00542 } 00543 break; 00544 00545 case 12: 00546 00547 for( i = 0; i < 8; i++, RK += 6 ) 00548 { 00549 RK[6] = RK[0] ^ RCON[i] ^ 00550 ( (uint32_t) FSb[ ( RK[5] >> 8 ) & 0xFF ] ) ^ 00551 ( (uint32_t) FSb[ ( RK[5] >> 16 ) & 0xFF ] << 8 ) ^ 00552 ( (uint32_t) FSb[ ( RK[5] >> 24 ) & 0xFF ] << 16 ) ^ 00553 ( (uint32_t) FSb[ ( RK[5] ) & 0xFF ] << 24 ); 00554 00555 RK[7] = RK[1] ^ RK[6]; 00556 RK[8] = RK[2] ^ RK[7]; 00557 RK[9] = RK[3] ^ RK[8]; 00558 RK[10] = RK[4] ^ RK[9]; 00559 RK[11] = RK[5] ^ RK[10]; 00560 } 00561 break; 00562 00563 case 14: 00564 00565 for( i = 0; i < 7; i++, RK += 8 ) 00566 { 00567 RK[8] = RK[0] ^ RCON[i] ^ 00568 ( (uint32_t) FSb[ ( RK[7] >> 8 ) & 0xFF ] ) ^ 00569 ( (uint32_t) FSb[ ( RK[7] >> 16 ) & 0xFF ] << 8 ) ^ 00570 ( (uint32_t) FSb[ ( RK[7] >> 24 ) & 0xFF ] << 16 ) ^ 00571 ( (uint32_t) FSb[ ( RK[7] ) & 0xFF ] << 24 ); 00572 00573 RK[9] = RK[1] ^ RK[8]; 00574 RK[10] = RK[2] ^ RK[9]; 00575 RK[11] = RK[3] ^ RK[10]; 00576 00577 RK[12] = RK[4] ^ 00578 ( (uint32_t) FSb[ ( RK[11] ) & 0xFF ] ) ^ 00579 ( (uint32_t) FSb[ ( RK[11] >> 8 ) & 0xFF ] << 8 ) ^ 00580 ( (uint32_t) FSb[ ( RK[11] >> 16 ) & 0xFF ] << 16 ) ^ 00581 ( (uint32_t) FSb[ ( RK[11] >> 24 ) & 0xFF ] << 24 ); 00582 00583 RK[13] = RK[5] ^ RK[12]; 00584 RK[14] = RK[6] ^ RK[13]; 00585 RK[15] = RK[7] ^ RK[14]; 00586 } 00587 break; 00588 } 00589 00590 return( 0 ); 00591 } 00592 #endif /* !MBEDTLS_AES_SETKEY_ENC_ALT */ 00593 00594 /* 00595 * AES key schedule (decryption) 00596 */ 00597 #if !defined(MBEDTLS_AES_SETKEY_DEC_ALT) 00598 int mbedtls_aes_setkey_dec( mbedtls_aes_context *ctx, const unsigned char *key, 00599 unsigned int keybits ) 00600 { 00601 int i, j, ret; 00602 mbedtls_aes_context cty; 00603 uint32_t *RK; 00604 uint32_t *SK; 00605 00606 mbedtls_aes_init( &cty ); 00607 00608 #if defined(MBEDTLS_PADLOCK_C) && defined(MBEDTLS_PADLOCK_ALIGN16) 00609 if( aes_padlock_ace == -1 ) 00610 aes_padlock_ace = mbedtls_padlock_has_support( MBEDTLS_PADLOCK_ACE ); 00611 00612 if( aes_padlock_ace ) 00613 ctx->rk = RK = MBEDTLS_PADLOCK_ALIGN16( ctx->buf ); 00614 else 00615 #endif 00616 ctx->rk = RK = ctx->buf ; 00617 00618 /* Also checks keybits */ 00619 if( ( ret = mbedtls_aes_setkey_enc( &cty, key, keybits ) ) != 0 ) 00620 goto exit; 00621 00622 ctx->nr = cty.nr ; 00623 00624 #if defined(MBEDTLS_AESNI_C) && defined(MBEDTLS_HAVE_X86_64) 00625 if( mbedtls_aesni_has_support( MBEDTLS_AESNI_AES ) ) 00626 { 00627 mbedtls_aesni_inverse_key( (unsigned char *) ctx->rk , 00628 (const unsigned char *) cty.rk , ctx->nr ); 00629 goto exit; 00630 } 00631 #endif 00632 00633 SK = cty.rk + cty.nr * 4; 00634 00635 *RK++ = *SK++; 00636 *RK++ = *SK++; 00637 *RK++ = *SK++; 00638 *RK++ = *SK++; 00639 00640 for( i = ctx->nr - 1, SK -= 8; i > 0; i--, SK -= 8 ) 00641 { 00642 for( j = 0; j < 4; j++, SK++ ) 00643 { 00644 *RK++ = RT0[ FSb[ ( *SK ) & 0xFF ] ] ^ 00645 RT1[ FSb[ ( *SK >> 8 ) & 0xFF ] ] ^ 00646 RT2[ FSb[ ( *SK >> 16 ) & 0xFF ] ] ^ 00647 RT3[ FSb[ ( *SK >> 24 ) & 0xFF ] ]; 00648 } 00649 } 00650 00651 *RK++ = *SK++; 00652 *RK++ = *SK++; 00653 *RK++ = *SK++; 00654 *RK++ = *SK++; 00655 00656 exit: 00657 mbedtls_aes_free( &cty ); 00658 00659 return( ret ); 00660 } 00661 #endif /* !MBEDTLS_AES_SETKEY_DEC_ALT */ 00662 00663 #define AES_FROUND(X0,X1,X2,X3,Y0,Y1,Y2,Y3) \ 00664 { \ 00665 X0 = *RK++ ^ FT0[ ( Y0 ) & 0xFF ] ^ \ 00666 FT1[ ( Y1 >> 8 ) & 0xFF ] ^ \ 00667 FT2[ ( Y2 >> 16 ) & 0xFF ] ^ \ 00668 FT3[ ( Y3 >> 24 ) & 0xFF ]; \ 00669 \ 00670 X1 = *RK++ ^ FT0[ ( Y1 ) & 0xFF ] ^ \ 00671 FT1[ ( Y2 >> 8 ) & 0xFF ] ^ \ 00672 FT2[ ( Y3 >> 16 ) & 0xFF ] ^ \ 00673 FT3[ ( Y0 >> 24 ) & 0xFF ]; \ 00674 \ 00675 X2 = *RK++ ^ FT0[ ( Y2 ) & 0xFF ] ^ \ 00676 FT1[ ( Y3 >> 8 ) & 0xFF ] ^ \ 00677 FT2[ ( Y0 >> 16 ) & 0xFF ] ^ \ 00678 FT3[ ( Y1 >> 24 ) & 0xFF ]; \ 00679 \ 00680 X3 = *RK++ ^ FT0[ ( Y3 ) & 0xFF ] ^ \ 00681 FT1[ ( Y0 >> 8 ) & 0xFF ] ^ \ 00682 FT2[ ( Y1 >> 16 ) & 0xFF ] ^ \ 00683 FT3[ ( Y2 >> 24 ) & 0xFF ]; \ 00684 } 00685 00686 #define AES_RROUND(X0,X1,X2,X3,Y0,Y1,Y2,Y3) \ 00687 { \ 00688 X0 = *RK++ ^ RT0[ ( Y0 ) & 0xFF ] ^ \ 00689 RT1[ ( Y3 >> 8 ) & 0xFF ] ^ \ 00690 RT2[ ( Y2 >> 16 ) & 0xFF ] ^ \ 00691 RT3[ ( Y1 >> 24 ) & 0xFF ]; \ 00692 \ 00693 X1 = *RK++ ^ RT0[ ( Y1 ) & 0xFF ] ^ \ 00694 RT1[ ( Y0 >> 8 ) & 0xFF ] ^ \ 00695 RT2[ ( Y3 >> 16 ) & 0xFF ] ^ \ 00696 RT3[ ( Y2 >> 24 ) & 0xFF ]; \ 00697 \ 00698 X2 = *RK++ ^ RT0[ ( Y2 ) & 0xFF ] ^ \ 00699 RT1[ ( Y1 >> 8 ) & 0xFF ] ^ \ 00700 RT2[ ( Y0 >> 16 ) & 0xFF ] ^ \ 00701 RT3[ ( Y3 >> 24 ) & 0xFF ]; \ 00702 \ 00703 X3 = *RK++ ^ RT0[ ( Y3 ) & 0xFF ] ^ \ 00704 RT1[ ( Y2 >> 8 ) & 0xFF ] ^ \ 00705 RT2[ ( Y1 >> 16 ) & 0xFF ] ^ \ 00706 RT3[ ( Y0 >> 24 ) & 0xFF ]; \ 00707 } 00708 00709 /* 00710 * AES-ECB block encryption 00711 */ 00712 #if !defined(MBEDTLS_AES_ENCRYPT_ALT) 00713 int mbedtls_internal_aes_encrypt( mbedtls_aes_context *ctx, 00714 const unsigned char input[16], 00715 unsigned char output[16] ) 00716 { 00717 int i; 00718 uint32_t *RK, X0, X1, X2, X3, Y0, Y1, Y2, Y3; 00719 00720 RK = ctx->rk ; 00721 00722 GET_UINT32_LE( X0, input, 0 ); X0 ^= *RK++; 00723 GET_UINT32_LE( X1, input, 4 ); X1 ^= *RK++; 00724 GET_UINT32_LE( X2, input, 8 ); X2 ^= *RK++; 00725 GET_UINT32_LE( X3, input, 12 ); X3 ^= *RK++; 00726 00727 for( i = ( ctx->nr >> 1 ) - 1; i > 0; i-- ) 00728 { 00729 AES_FROUND( Y0, Y1, Y2, Y3, X0, X1, X2, X3 ); 00730 AES_FROUND( X0, X1, X2, X3, Y0, Y1, Y2, Y3 ); 00731 } 00732 00733 AES_FROUND( Y0, Y1, Y2, Y3, X0, X1, X2, X3 ); 00734 00735 X0 = *RK++ ^ \ 00736 ( (uint32_t) FSb[ ( Y0 ) & 0xFF ] ) ^ 00737 ( (uint32_t) FSb[ ( Y1 >> 8 ) & 0xFF ] << 8 ) ^ 00738 ( (uint32_t) FSb[ ( Y2 >> 16 ) & 0xFF ] << 16 ) ^ 00739 ( (uint32_t) FSb[ ( Y3 >> 24 ) & 0xFF ] << 24 ); 00740 00741 X1 = *RK++ ^ \ 00742 ( (uint32_t) FSb[ ( Y1 ) & 0xFF ] ) ^ 00743 ( (uint32_t) FSb[ ( Y2 >> 8 ) & 0xFF ] << 8 ) ^ 00744 ( (uint32_t) FSb[ ( Y3 >> 16 ) & 0xFF ] << 16 ) ^ 00745 ( (uint32_t) FSb[ ( Y0 >> 24 ) & 0xFF ] << 24 ); 00746 00747 X2 = *RK++ ^ \ 00748 ( (uint32_t) FSb[ ( Y2 ) & 0xFF ] ) ^ 00749 ( (uint32_t) FSb[ ( Y3 >> 8 ) & 0xFF ] << 8 ) ^ 00750 ( (uint32_t) FSb[ ( Y0 >> 16 ) & 0xFF ] << 16 ) ^ 00751 ( (uint32_t) FSb[ ( Y1 >> 24 ) & 0xFF ] << 24 ); 00752 00753 X3 = *RK++ ^ \ 00754 ( (uint32_t) FSb[ ( Y3 ) & 0xFF ] ) ^ 00755 ( (uint32_t) FSb[ ( Y0 >> 8 ) & 0xFF ] << 8 ) ^ 00756 ( (uint32_t) FSb[ ( Y1 >> 16 ) & 0xFF ] << 16 ) ^ 00757 ( (uint32_t) FSb[ ( Y2 >> 24 ) & 0xFF ] << 24 ); 00758 00759 PUT_UINT32_LE( X0, output, 0 ); 00760 PUT_UINT32_LE( X1, output, 4 ); 00761 PUT_UINT32_LE( X2, output, 8 ); 00762 PUT_UINT32_LE( X3, output, 12 ); 00763 00764 return( 0 ); 00765 } 00766 #endif /* !MBEDTLS_AES_ENCRYPT_ALT */ 00767 00768 void mbedtls_aes_encrypt( mbedtls_aes_context *ctx, 00769 const unsigned char input[16], 00770 unsigned char output[16] ) 00771 { 00772 mbedtls_internal_aes_encrypt( ctx, input, output ); 00773 } 00774 00775 /* 00776 * AES-ECB block decryption 00777 */ 00778 #if !defined(MBEDTLS_AES_DECRYPT_ALT) 00779 int mbedtls_internal_aes_decrypt( mbedtls_aes_context *ctx, 00780 const unsigned char input[16], 00781 unsigned char output[16] ) 00782 { 00783 int i; 00784 uint32_t *RK, X0, X1, X2, X3, Y0, Y1, Y2, Y3; 00785 00786 RK = ctx->rk ; 00787 00788 GET_UINT32_LE( X0, input, 0 ); X0 ^= *RK++; 00789 GET_UINT32_LE( X1, input, 4 ); X1 ^= *RK++; 00790 GET_UINT32_LE( X2, input, 8 ); X2 ^= *RK++; 00791 GET_UINT32_LE( X3, input, 12 ); X3 ^= *RK++; 00792 00793 for( i = ( ctx->nr >> 1 ) - 1; i > 0; i-- ) 00794 { 00795 AES_RROUND( Y0, Y1, Y2, Y3, X0, X1, X2, X3 ); 00796 AES_RROUND( X0, X1, X2, X3, Y0, Y1, Y2, Y3 ); 00797 } 00798 00799 AES_RROUND( Y0, Y1, Y2, Y3, X0, X1, X2, X3 ); 00800 00801 X0 = *RK++ ^ \ 00802 ( (uint32_t) RSb[ ( Y0 ) & 0xFF ] ) ^ 00803 ( (uint32_t) RSb[ ( Y3 >> 8 ) & 0xFF ] << 8 ) ^ 00804 ( (uint32_t) RSb[ ( Y2 >> 16 ) & 0xFF ] << 16 ) ^ 00805 ( (uint32_t) RSb[ ( Y1 >> 24 ) & 0xFF ] << 24 ); 00806 00807 X1 = *RK++ ^ \ 00808 ( (uint32_t) RSb[ ( Y1 ) & 0xFF ] ) ^ 00809 ( (uint32_t) RSb[ ( Y0 >> 8 ) & 0xFF ] << 8 ) ^ 00810 ( (uint32_t) RSb[ ( Y3 >> 16 ) & 0xFF ] << 16 ) ^ 00811 ( (uint32_t) RSb[ ( Y2 >> 24 ) & 0xFF ] << 24 ); 00812 00813 X2 = *RK++ ^ \ 00814 ( (uint32_t) RSb[ ( Y2 ) & 0xFF ] ) ^ 00815 ( (uint32_t) RSb[ ( Y1 >> 8 ) & 0xFF ] << 8 ) ^ 00816 ( (uint32_t) RSb[ ( Y0 >> 16 ) & 0xFF ] << 16 ) ^ 00817 ( (uint32_t) RSb[ ( Y3 >> 24 ) & 0xFF ] << 24 ); 00818 00819 X3 = *RK++ ^ \ 00820 ( (uint32_t) RSb[ ( Y3 ) & 0xFF ] ) ^ 00821 ( (uint32_t) RSb[ ( Y2 >> 8 ) & 0xFF ] << 8 ) ^ 00822 ( (uint32_t) RSb[ ( Y1 >> 16 ) & 0xFF ] << 16 ) ^ 00823 ( (uint32_t) RSb[ ( Y0 >> 24 ) & 0xFF ] << 24 ); 00824 00825 PUT_UINT32_LE( X0, output, 0 ); 00826 PUT_UINT32_LE( X1, output, 4 ); 00827 PUT_UINT32_LE( X2, output, 8 ); 00828 PUT_UINT32_LE( X3, output, 12 ); 00829 00830 return( 0 ); 00831 } 00832 #endif /* !MBEDTLS_AES_DECRYPT_ALT */ 00833 00834 void mbedtls_aes_decrypt( mbedtls_aes_context *ctx, 00835 const unsigned char input[16], 00836 unsigned char output[16] ) 00837 { 00838 mbedtls_internal_aes_decrypt( ctx, input, output ); 00839 } 00840 00841 /* 00842 * AES-ECB block encryption/decryption 00843 */ 00844 int mbedtls_aes_crypt_ecb( mbedtls_aes_context *ctx, 00845 int mode, 00846 const unsigned char input[16], 00847 unsigned char output[16] ) 00848 { 00849 #if defined(MBEDTLS_AESNI_C) && defined(MBEDTLS_HAVE_X86_64) 00850 if( mbedtls_aesni_has_support( MBEDTLS_AESNI_AES ) ) 00851 return( mbedtls_aesni_crypt_ecb( ctx, mode, input, output ) ); 00852 #endif 00853 00854 #if defined(MBEDTLS_PADLOCK_C) && defined(MBEDTLS_HAVE_X86) 00855 if( aes_padlock_ace ) 00856 { 00857 if( mbedtls_padlock_xcryptecb( ctx, mode, input, output ) == 0 ) 00858 return( 0 ); 00859 00860 // If padlock data misaligned, we just fall back to 00861 // unaccelerated mode 00862 // 00863 } 00864 #endif 00865 00866 if( mode == MBEDTLS_AES_ENCRYPT ) 00867 return( mbedtls_internal_aes_encrypt( ctx, input, output ) ); 00868 else 00869 return( mbedtls_internal_aes_decrypt( ctx, input, output ) ); 00870 } 00871 00872 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00873 /* 00874 * AES-CBC buffer encryption/decryption 00875 */ 00876 int mbedtls_aes_crypt_cbc( mbedtls_aes_context *ctx, 00877 int mode, 00878 size_t length, 00879 unsigned char iv[16], 00880 const unsigned char *input, 00881 unsigned char *output ) 00882 { 00883 int i; 00884 unsigned char temp[16]; 00885 00886 if( length % 16 ) 00887 return( MBEDTLS_ERR_AES_INVALID_INPUT_LENGTH ); 00888 00889 #if defined(MBEDTLS_PADLOCK_C) && defined(MBEDTLS_HAVE_X86) 00890 if( aes_padlock_ace ) 00891 { 00892 if( mbedtls_padlock_xcryptcbc( ctx, mode, length, iv, input, output ) == 0 ) 00893 return( 0 ); 00894 00895 // If padlock data misaligned, we just fall back to 00896 // unaccelerated mode 00897 // 00898 } 00899 #endif 00900 00901 if( mode == MBEDTLS_AES_DECRYPT ) 00902 { 00903 while( length > 0 ) 00904 { 00905 memcpy( temp, input, 16 ); 00906 mbedtls_aes_crypt_ecb( ctx, mode, input, output ); 00907 00908 for( i = 0; i < 16; i++ ) 00909 output[i] = (unsigned char)( output[i] ^ iv[i] ); 00910 00911 memcpy( iv, temp, 16 ); 00912 00913 input += 16; 00914 output += 16; 00915 length -= 16; 00916 } 00917 } 00918 else 00919 { 00920 while( length > 0 ) 00921 { 00922 for( i = 0; i < 16; i++ ) 00923 output[i] = (unsigned char)( input[i] ^ iv[i] ); 00924 00925 mbedtls_aes_crypt_ecb( ctx, mode, output, output ); 00926 memcpy( iv, output, 16 ); 00927 00928 input += 16; 00929 output += 16; 00930 length -= 16; 00931 } 00932 } 00933 00934 return( 0 ); 00935 } 00936 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00937 00938 #if defined(MBEDTLS_CIPHER_MODE_CFB) 00939 /* 00940 * AES-CFB128 buffer encryption/decryption 00941 */ 00942 int mbedtls_aes_crypt_cfb128( mbedtls_aes_context *ctx, 00943 int mode, 00944 size_t length, 00945 size_t *iv_off, 00946 unsigned char iv[16], 00947 const unsigned char *input, 00948 unsigned char *output ) 00949 { 00950 int c; 00951 size_t n = *iv_off; 00952 00953 if( mode == MBEDTLS_AES_DECRYPT ) 00954 { 00955 while( length-- ) 00956 { 00957 if( n == 0 ) 00958 mbedtls_aes_crypt_ecb( ctx, MBEDTLS_AES_ENCRYPT, iv, iv ); 00959 00960 c = *input++; 00961 *output++ = (unsigned char)( c ^ iv[n] ); 00962 iv[n] = (unsigned char) c; 00963 00964 n = ( n + 1 ) & 0x0F; 00965 } 00966 } 00967 else 00968 { 00969 while( length-- ) 00970 { 00971 if( n == 0 ) 00972 mbedtls_aes_crypt_ecb( ctx, MBEDTLS_AES_ENCRYPT, iv, iv ); 00973 00974 iv[n] = *output++ = (unsigned char)( iv[n] ^ *input++ ); 00975 00976 n = ( n + 1 ) & 0x0F; 00977 } 00978 } 00979 00980 *iv_off = n; 00981 00982 return( 0 ); 00983 } 00984 00985 /* 00986 * AES-CFB8 buffer encryption/decryption 00987 */ 00988 int mbedtls_aes_crypt_cfb8( mbedtls_aes_context *ctx, 00989 int mode, 00990 size_t length, 00991 unsigned char iv[16], 00992 const unsigned char *input, 00993 unsigned char *output ) 00994 { 00995 unsigned char c; 00996 unsigned char ov[17]; 00997 00998 while( length-- ) 00999 { 01000 memcpy( ov, iv, 16 ); 01001 mbedtls_aes_crypt_ecb( ctx, MBEDTLS_AES_ENCRYPT, iv, iv ); 01002 01003 if( mode == MBEDTLS_AES_DECRYPT ) 01004 ov[16] = *input; 01005 01006 c = *output++ = (unsigned char)( iv[0] ^ *input++ ); 01007 01008 if( mode == MBEDTLS_AES_ENCRYPT ) 01009 ov[16] = c; 01010 01011 memcpy( iv, ov + 1, 16 ); 01012 } 01013 01014 return( 0 ); 01015 } 01016 #endif /*MBEDTLS_CIPHER_MODE_CFB */ 01017 01018 #if defined(MBEDTLS_CIPHER_MODE_CTR) 01019 /* 01020 * AES-CTR buffer encryption/decryption 01021 */ 01022 int mbedtls_aes_crypt_ctr( mbedtls_aes_context *ctx, 01023 size_t length, 01024 size_t *nc_off, 01025 unsigned char nonce_counter[16], 01026 unsigned char stream_block[16], 01027 const unsigned char *input, 01028 unsigned char *output ) 01029 { 01030 int c, i; 01031 size_t n = *nc_off; 01032 01033 while( length-- ) 01034 { 01035 if( n == 0 ) { 01036 mbedtls_aes_crypt_ecb( ctx, MBEDTLS_AES_ENCRYPT, nonce_counter, stream_block ); 01037 01038 for( i = 16; i > 0; i-- ) 01039 if( ++nonce_counter[i - 1] != 0 ) 01040 break; 01041 } 01042 c = *input++; 01043 *output++ = (unsigned char)( c ^ stream_block[n] ); 01044 01045 n = ( n + 1 ) & 0x0F; 01046 } 01047 01048 *nc_off = n; 01049 01050 return( 0 ); 01051 } 01052 #endif /* MBEDTLS_CIPHER_MODE_CTR */ 01053 01054 #endif /* !MBEDTLS_AES_ALT */ 01055 01056 #if defined(MBEDTLS_SELF_TEST) 01057 /* 01058 * AES test vectors from: 01059 * 01060 * http://csrc.nist.gov/archive/aes/rijndael/rijndael-vals.zip 01061 */ 01062 static const unsigned char aes_test_ecb_dec[3][16] = 01063 { 01064 { 0x44, 0x41, 0x6A, 0xC2, 0xD1, 0xF5, 0x3C, 0x58, 01065 0x33, 0x03, 0x91, 0x7E, 0x6B, 0xE9, 0xEB, 0xE0 }, 01066 { 0x48, 0xE3, 0x1E, 0x9E, 0x25, 0x67, 0x18, 0xF2, 01067 0x92, 0x29, 0x31, 0x9C, 0x19, 0xF1, 0x5B, 0xA4 }, 01068 { 0x05, 0x8C, 0xCF, 0xFD, 0xBB, 0xCB, 0x38, 0x2D, 01069 0x1F, 0x6F, 0x56, 0x58, 0x5D, 0x8A, 0x4A, 0xDE } 01070 }; 01071 01072 static const unsigned char aes_test_ecb_enc[3][16] = 01073 { 01074 { 0xC3, 0x4C, 0x05, 0x2C, 0xC0, 0xDA, 0x8D, 0x73, 01075 0x45, 0x1A, 0xFE, 0x5F, 0x03, 0xBE, 0x29, 0x7F }, 01076 { 0xF3, 0xF6, 0x75, 0x2A, 0xE8, 0xD7, 0x83, 0x11, 01077 0x38, 0xF0, 0x41, 0x56, 0x06, 0x31, 0xB1, 0x14 }, 01078 { 0x8B, 0x79, 0xEE, 0xCC, 0x93, 0xA0, 0xEE, 0x5D, 01079 0xFF, 0x30, 0xB4, 0xEA, 0x21, 0x63, 0x6D, 0xA4 } 01080 }; 01081 01082 #if defined(MBEDTLS_CIPHER_MODE_CBC) 01083 static const unsigned char aes_test_cbc_dec[3][16] = 01084 { 01085 { 0xFA, 0xCA, 0x37, 0xE0, 0xB0, 0xC8, 0x53, 0x73, 01086 0xDF, 0x70, 0x6E, 0x73, 0xF7, 0xC9, 0xAF, 0x86 }, 01087 { 0x5D, 0xF6, 0x78, 0xDD, 0x17, 0xBA, 0x4E, 0x75, 01088 0xB6, 0x17, 0x68, 0xC6, 0xAD, 0xEF, 0x7C, 0x7B }, 01089 { 0x48, 0x04, 0xE1, 0x81, 0x8F, 0xE6, 0x29, 0x75, 01090 0x19, 0xA3, 0xE8, 0x8C, 0x57, 0x31, 0x04, 0x13 } 01091 }; 01092 01093 static const unsigned char aes_test_cbc_enc[3][16] = 01094 { 01095 { 0x8A, 0x05, 0xFC, 0x5E, 0x09, 0x5A, 0xF4, 0x84, 01096 0x8A, 0x08, 0xD3, 0x28, 0xD3, 0x68, 0x8E, 0x3D }, 01097 { 0x7B, 0xD9, 0x66, 0xD5, 0x3A, 0xD8, 0xC1, 0xBB, 01098 0x85, 0xD2, 0xAD, 0xFA, 0xE8, 0x7B, 0xB1, 0x04 }, 01099 { 0xFE, 0x3C, 0x53, 0x65, 0x3E, 0x2F, 0x45, 0xB5, 01100 0x6F, 0xCD, 0x88, 0xB2, 0xCC, 0x89, 0x8F, 0xF0 } 01101 }; 01102 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 01103 01104 #if defined(MBEDTLS_CIPHER_MODE_CFB) 01105 /* 01106 * AES-CFB128 test vectors from: 01107 * 01108 * http://csrc.nist.gov/publications/nistpubs/800-38a/sp800-38a.pdf 01109 */ 01110 static const unsigned char aes_test_cfb128_key[3][32] = 01111 { 01112 { 0x2B, 0x7E, 0x15, 0x16, 0x28, 0xAE, 0xD2, 0xA6, 01113 0xAB, 0xF7, 0x15, 0x88, 0x09, 0xCF, 0x4F, 0x3C }, 01114 { 0x8E, 0x73, 0xB0, 0xF7, 0xDA, 0x0E, 0x64, 0x52, 01115 0xC8, 0x10, 0xF3, 0x2B, 0x80, 0x90, 0x79, 0xE5, 01116 0x62, 0xF8, 0xEA, 0xD2, 0x52, 0x2C, 0x6B, 0x7B }, 01117 { 0x60, 0x3D, 0xEB, 0x10, 0x15, 0xCA, 0x71, 0xBE, 01118 0x2B, 0x73, 0xAE, 0xF0, 0x85, 0x7D, 0x77, 0x81, 01119 0x1F, 0x35, 0x2C, 0x07, 0x3B, 0x61, 0x08, 0xD7, 01120 0x2D, 0x98, 0x10, 0xA3, 0x09, 0x14, 0xDF, 0xF4 } 01121 }; 01122 01123 static const unsigned char aes_test_cfb128_iv[16] = 01124 { 01125 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 01126 0x08, 0x09, 0x0A, 0x0B, 0x0C, 0x0D, 0x0E, 0x0F 01127 }; 01128 01129 static const unsigned char aes_test_cfb128_pt[64] = 01130 { 01131 0x6B, 0xC1, 0xBE, 0xE2, 0x2E, 0x40, 0x9F, 0x96, 01132 0xE9, 0x3D, 0x7E, 0x11, 0x73, 0x93, 0x17, 0x2A, 01133 0xAE, 0x2D, 0x8A, 0x57, 0x1E, 0x03, 0xAC, 0x9C, 01134 0x9E, 0xB7, 0x6F, 0xAC, 0x45, 0xAF, 0x8E, 0x51, 01135 0x30, 0xC8, 0x1C, 0x46, 0xA3, 0x5C, 0xE4, 0x11, 01136 0xE5, 0xFB, 0xC1, 0x19, 0x1A, 0x0A, 0x52, 0xEF, 01137 0xF6, 0x9F, 0x24, 0x45, 0xDF, 0x4F, 0x9B, 0x17, 01138 0xAD, 0x2B, 0x41, 0x7B, 0xE6, 0x6C, 0x37, 0x10 01139 }; 01140 01141 static const unsigned char aes_test_cfb128_ct[3][64] = 01142 { 01143 { 0x3B, 0x3F, 0xD9, 0x2E, 0xB7, 0x2D, 0xAD, 0x20, 01144 0x33, 0x34, 0x49, 0xF8, 0xE8, 0x3C, 0xFB, 0x4A, 01145 0xC8, 0xA6, 0x45, 0x37, 0xA0, 0xB3, 0xA9, 0x3F, 01146 0xCD, 0xE3, 0xCD, 0xAD, 0x9F, 0x1C, 0xE5, 0x8B, 01147 0x26, 0x75, 0x1F, 0x67, 0xA3, 0xCB, 0xB1, 0x40, 01148 0xB1, 0x80, 0x8C, 0xF1, 0x87, 0xA4, 0xF4, 0xDF, 01149 0xC0, 0x4B, 0x05, 0x35, 0x7C, 0x5D, 0x1C, 0x0E, 01150 0xEA, 0xC4, 0xC6, 0x6F, 0x9F, 0xF7, 0xF2, 0xE6 }, 01151 { 0xCD, 0xC8, 0x0D, 0x6F, 0xDD, 0xF1, 0x8C, 0xAB, 01152 0x34, 0xC2, 0x59, 0x09, 0xC9, 0x9A, 0x41, 0x74, 01153 0x67, 0xCE, 0x7F, 0x7F, 0x81, 0x17, 0x36, 0x21, 01154 0x96, 0x1A, 0x2B, 0x70, 0x17, 0x1D, 0x3D, 0x7A, 01155 0x2E, 0x1E, 0x8A, 0x1D, 0xD5, 0x9B, 0x88, 0xB1, 01156 0xC8, 0xE6, 0x0F, 0xED, 0x1E, 0xFA, 0xC4, 0xC9, 01157 0xC0, 0x5F, 0x9F, 0x9C, 0xA9, 0x83, 0x4F, 0xA0, 01158 0x42, 0xAE, 0x8F, 0xBA, 0x58, 0x4B, 0x09, 0xFF }, 01159 { 0xDC, 0x7E, 0x84, 0xBF, 0xDA, 0x79, 0x16, 0x4B, 01160 0x7E, 0xCD, 0x84, 0x86, 0x98, 0x5D, 0x38, 0x60, 01161 0x39, 0xFF, 0xED, 0x14, 0x3B, 0x28, 0xB1, 0xC8, 01162 0x32, 0x11, 0x3C, 0x63, 0x31, 0xE5, 0x40, 0x7B, 01163 0xDF, 0x10, 0x13, 0x24, 0x15, 0xE5, 0x4B, 0x92, 01164 0xA1, 0x3E, 0xD0, 0xA8, 0x26, 0x7A, 0xE2, 0xF9, 01165 0x75, 0xA3, 0x85, 0x74, 0x1A, 0xB9, 0xCE, 0xF8, 01166 0x20, 0x31, 0x62, 0x3D, 0x55, 0xB1, 0xE4, 0x71 } 01167 }; 01168 #endif /* MBEDTLS_CIPHER_MODE_CFB */ 01169 01170 #if defined(MBEDTLS_CIPHER_MODE_CTR) 01171 /* 01172 * AES-CTR test vectors from: 01173 * 01174 * http://www.faqs.org/rfcs/rfc3686.html 01175 */ 01176 01177 static const unsigned char aes_test_ctr_key[3][16] = 01178 { 01179 { 0xAE, 0x68, 0x52, 0xF8, 0x12, 0x10, 0x67, 0xCC, 01180 0x4B, 0xF7, 0xA5, 0x76, 0x55, 0x77, 0xF3, 0x9E }, 01181 { 0x7E, 0x24, 0x06, 0x78, 0x17, 0xFA, 0xE0, 0xD7, 01182 0x43, 0xD6, 0xCE, 0x1F, 0x32, 0x53, 0x91, 0x63 }, 01183 { 0x76, 0x91, 0xBE, 0x03, 0x5E, 0x50, 0x20, 0xA8, 01184 0xAC, 0x6E, 0x61, 0x85, 0x29, 0xF9, 0xA0, 0xDC } 01185 }; 01186 01187 static const unsigned char aes_test_ctr_nonce_counter[3][16] = 01188 { 01189 { 0x00, 0x00, 0x00, 0x30, 0x00, 0x00, 0x00, 0x00, 01190 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01 }, 01191 { 0x00, 0x6C, 0xB6, 0xDB, 0xC0, 0x54, 0x3B, 0x59, 01192 0xDA, 0x48, 0xD9, 0x0B, 0x00, 0x00, 0x00, 0x01 }, 01193 { 0x00, 0xE0, 0x01, 0x7B, 0x27, 0x77, 0x7F, 0x3F, 01194 0x4A, 0x17, 0x86, 0xF0, 0x00, 0x00, 0x00, 0x01 } 01195 }; 01196 01197 static const unsigned char aes_test_ctr_pt[3][48] = 01198 { 01199 { 0x53, 0x69, 0x6E, 0x67, 0x6C, 0x65, 0x20, 0x62, 01200 0x6C, 0x6F, 0x63, 0x6B, 0x20, 0x6D, 0x73, 0x67 }, 01201 01202 { 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 01203 0x08, 0x09, 0x0A, 0x0B, 0x0C, 0x0D, 0x0E, 0x0F, 01204 0x10, 0x11, 0x12, 0x13, 0x14, 0x15, 0x16, 0x17, 01205 0x18, 0x19, 0x1A, 0x1B, 0x1C, 0x1D, 0x1E, 0x1F }, 01206 01207 { 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 01208 0x08, 0x09, 0x0A, 0x0B, 0x0C, 0x0D, 0x0E, 0x0F, 01209 0x10, 0x11, 0x12, 0x13, 0x14, 0x15, 0x16, 0x17, 01210 0x18, 0x19, 0x1A, 0x1B, 0x1C, 0x1D, 0x1E, 0x1F, 01211 0x20, 0x21, 0x22, 0x23 } 01212 }; 01213 01214 static const unsigned char aes_test_ctr_ct[3][48] = 01215 { 01216 { 0xE4, 0x09, 0x5D, 0x4F, 0xB7, 0xA7, 0xB3, 0x79, 01217 0x2D, 0x61, 0x75, 0xA3, 0x26, 0x13, 0x11, 0xB8 }, 01218 { 0x51, 0x04, 0xA1, 0x06, 0x16, 0x8A, 0x72, 0xD9, 01219 0x79, 0x0D, 0x41, 0xEE, 0x8E, 0xDA, 0xD3, 0x88, 01220 0xEB, 0x2E, 0x1E, 0xFC, 0x46, 0xDA, 0x57, 0xC8, 01221 0xFC, 0xE6, 0x30, 0xDF, 0x91, 0x41, 0xBE, 0x28 }, 01222 { 0xC1, 0xCF, 0x48, 0xA8, 0x9F, 0x2F, 0xFD, 0xD9, 01223 0xCF, 0x46, 0x52, 0xE9, 0xEF, 0xDB, 0x72, 0xD7, 01224 0x45, 0x40, 0xA4, 0x2B, 0xDE, 0x6D, 0x78, 0x36, 01225 0xD5, 0x9A, 0x5C, 0xEA, 0xAE, 0xF3, 0x10, 0x53, 01226 0x25, 0xB2, 0x07, 0x2F } 01227 }; 01228 01229 static const int aes_test_ctr_len[3] = 01230 { 16, 32, 36 }; 01231 #endif /* MBEDTLS_CIPHER_MODE_CTR */ 01232 01233 /* 01234 * Checkup routine 01235 */ 01236 int mbedtls_aes_self_test( int verbose ) 01237 { 01238 int ret = 0, i, j, u, v; 01239 unsigned char key[32]; 01240 unsigned char buf[64]; 01241 #if defined(MBEDTLS_CIPHER_MODE_CBC) || defined(MBEDTLS_CIPHER_MODE_CFB) 01242 unsigned char iv[16]; 01243 #endif 01244 #if defined(MBEDTLS_CIPHER_MODE_CBC) 01245 unsigned char prv[16]; 01246 #endif 01247 #if defined(MBEDTLS_CIPHER_MODE_CTR) || defined(MBEDTLS_CIPHER_MODE_CFB) 01248 size_t offset; 01249 #endif 01250 #if defined(MBEDTLS_CIPHER_MODE_CTR) 01251 int len; 01252 unsigned char nonce_counter[16]; 01253 unsigned char stream_block[16]; 01254 #endif 01255 mbedtls_aes_context ctx; 01256 01257 memset( key, 0, 32 ); 01258 mbedtls_aes_init( &ctx ); 01259 01260 /* 01261 * ECB mode 01262 */ 01263 for( i = 0; i < 6; i++ ) 01264 { 01265 u = i >> 1; 01266 v = i & 1; 01267 01268 if( verbose != 0 ) 01269 mbedtls_printf( " AES-ECB-%3d (%s): ", 128 + u * 64, 01270 ( v == MBEDTLS_AES_DECRYPT ) ? "dec" : "enc" ); 01271 01272 memset( buf, 0, 16 ); 01273 01274 if( v == MBEDTLS_AES_DECRYPT ) 01275 { 01276 mbedtls_aes_setkey_dec( &ctx, key, 128 + u * 64 ); 01277 01278 for( j = 0; j < 10000; j++ ) 01279 mbedtls_aes_crypt_ecb( &ctx, v, buf, buf ); 01280 01281 if( memcmp( buf, aes_test_ecb_dec[u], 16 ) != 0 ) 01282 { 01283 if( verbose != 0 ) 01284 mbedtls_printf( "failed\n" ); 01285 01286 ret = 1; 01287 goto exit; 01288 } 01289 } 01290 else 01291 { 01292 mbedtls_aes_setkey_enc( &ctx, key, 128 + u * 64 ); 01293 01294 for( j = 0; j < 10000; j++ ) 01295 mbedtls_aes_crypt_ecb( &ctx, v, buf, buf ); 01296 01297 if( memcmp( buf, aes_test_ecb_enc[u], 16 ) != 0 ) 01298 { 01299 if( verbose != 0 ) 01300 mbedtls_printf( "failed\n" ); 01301 01302 ret = 1; 01303 goto exit; 01304 } 01305 } 01306 01307 if( verbose != 0 ) 01308 mbedtls_printf( "passed\n" ); 01309 } 01310 01311 if( verbose != 0 ) 01312 mbedtls_printf( "\n" ); 01313 01314 #if defined(MBEDTLS_CIPHER_MODE_CBC) 01315 /* 01316 * CBC mode 01317 */ 01318 for( i = 0; i < 6; i++ ) 01319 { 01320 u = i >> 1; 01321 v = i & 1; 01322 01323 if( verbose != 0 ) 01324 mbedtls_printf( " AES-CBC-%3d (%s): ", 128 + u * 64, 01325 ( v == MBEDTLS_AES_DECRYPT ) ? "dec" : "enc" ); 01326 01327 memset( iv , 0, 16 ); 01328 memset( prv, 0, 16 ); 01329 memset( buf, 0, 16 ); 01330 01331 if( v == MBEDTLS_AES_DECRYPT ) 01332 { 01333 mbedtls_aes_setkey_dec( &ctx, key, 128 + u * 64 ); 01334 01335 for( j = 0; j < 10000; j++ ) 01336 mbedtls_aes_crypt_cbc( &ctx, v, 16, iv, buf, buf ); 01337 01338 if( memcmp( buf, aes_test_cbc_dec[u], 16 ) != 0 ) 01339 { 01340 if( verbose != 0 ) 01341 mbedtls_printf( "failed\n" ); 01342 01343 ret = 1; 01344 goto exit; 01345 } 01346 } 01347 else 01348 { 01349 mbedtls_aes_setkey_enc( &ctx, key, 128 + u * 64 ); 01350 01351 for( j = 0; j < 10000; j++ ) 01352 { 01353 unsigned char tmp[16]; 01354 01355 mbedtls_aes_crypt_cbc( &ctx, v, 16, iv, buf, buf ); 01356 01357 memcpy( tmp, prv, 16 ); 01358 memcpy( prv, buf, 16 ); 01359 memcpy( buf, tmp, 16 ); 01360 } 01361 01362 if( memcmp( prv, aes_test_cbc_enc[u], 16 ) != 0 ) 01363 { 01364 if( verbose != 0 ) 01365 mbedtls_printf( "failed\n" ); 01366 01367 ret = 1; 01368 goto exit; 01369 } 01370 } 01371 01372 if( verbose != 0 ) 01373 mbedtls_printf( "passed\n" ); 01374 } 01375 01376 if( verbose != 0 ) 01377 mbedtls_printf( "\n" ); 01378 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 01379 01380 #if defined(MBEDTLS_CIPHER_MODE_CFB) 01381 /* 01382 * CFB128 mode 01383 */ 01384 for( i = 0; i < 6; i++ ) 01385 { 01386 u = i >> 1; 01387 v = i & 1; 01388 01389 if( verbose != 0 ) 01390 mbedtls_printf( " AES-CFB128-%3d (%s): ", 128 + u * 64, 01391 ( v == MBEDTLS_AES_DECRYPT ) ? "dec" : "enc" ); 01392 01393 memcpy( iv, aes_test_cfb128_iv, 16 ); 01394 memcpy( key, aes_test_cfb128_key[u], 16 + u * 8 ); 01395 01396 offset = 0; 01397 mbedtls_aes_setkey_enc( &ctx, key, 128 + u * 64 ); 01398 01399 if( v == MBEDTLS_AES_DECRYPT ) 01400 { 01401 memcpy( buf, aes_test_cfb128_ct[u], 64 ); 01402 mbedtls_aes_crypt_cfb128( &ctx, v, 64, &offset, iv, buf, buf ); 01403 01404 if( memcmp( buf, aes_test_cfb128_pt, 64 ) != 0 ) 01405 { 01406 if( verbose != 0 ) 01407 mbedtls_printf( "failed\n" ); 01408 01409 ret = 1; 01410 goto exit; 01411 } 01412 } 01413 else 01414 { 01415 memcpy( buf, aes_test_cfb128_pt, 64 ); 01416 mbedtls_aes_crypt_cfb128( &ctx, v, 64, &offset, iv, buf, buf ); 01417 01418 if( memcmp( buf, aes_test_cfb128_ct[u], 64 ) != 0 ) 01419 { 01420 if( verbose != 0 ) 01421 mbedtls_printf( "failed\n" ); 01422 01423 ret = 1; 01424 goto exit; 01425 } 01426 } 01427 01428 if( verbose != 0 ) 01429 mbedtls_printf( "passed\n" ); 01430 } 01431 01432 if( verbose != 0 ) 01433 mbedtls_printf( "\n" ); 01434 #endif /* MBEDTLS_CIPHER_MODE_CFB */ 01435 01436 #if defined(MBEDTLS_CIPHER_MODE_CTR) 01437 /* 01438 * CTR mode 01439 */ 01440 for( i = 0; i < 6; i++ ) 01441 { 01442 u = i >> 1; 01443 v = i & 1; 01444 01445 if( verbose != 0 ) 01446 mbedtls_printf( " AES-CTR-128 (%s): ", 01447 ( v == MBEDTLS_AES_DECRYPT ) ? "dec" : "enc" ); 01448 01449 memcpy( nonce_counter, aes_test_ctr_nonce_counter[u], 16 ); 01450 memcpy( key, aes_test_ctr_key[u], 16 ); 01451 01452 offset = 0; 01453 mbedtls_aes_setkey_enc( &ctx, key, 128 ); 01454 01455 if( v == MBEDTLS_AES_DECRYPT ) 01456 { 01457 len = aes_test_ctr_len[u]; 01458 memcpy( buf, aes_test_ctr_ct[u], len ); 01459 01460 mbedtls_aes_crypt_ctr( &ctx, len, &offset, nonce_counter, stream_block, 01461 buf, buf ); 01462 01463 if( memcmp( buf, aes_test_ctr_pt[u], len ) != 0 ) 01464 { 01465 if( verbose != 0 ) 01466 mbedtls_printf( "failed\n" ); 01467 01468 ret = 1; 01469 goto exit; 01470 } 01471 } 01472 else 01473 { 01474 len = aes_test_ctr_len[u]; 01475 memcpy( buf, aes_test_ctr_pt[u], len ); 01476 01477 mbedtls_aes_crypt_ctr( &ctx, len, &offset, nonce_counter, stream_block, 01478 buf, buf ); 01479 01480 if( memcmp( buf, aes_test_ctr_ct[u], len ) != 0 ) 01481 { 01482 if( verbose != 0 ) 01483 mbedtls_printf( "failed\n" ); 01484 01485 ret = 1; 01486 goto exit; 01487 } 01488 } 01489 01490 if( verbose != 0 ) 01491 mbedtls_printf( "passed\n" ); 01492 } 01493 01494 if( verbose != 0 ) 01495 mbedtls_printf( "\n" ); 01496 #endif /* MBEDTLS_CIPHER_MODE_CTR */ 01497 01498 ret = 0; 01499 01500 exit: 01501 mbedtls_aes_free( &ctx ); 01502 01503 return( ret ); 01504 } 01505 01506 #endif /* MBEDTLS_SELF_TEST */ 01507 01508 #endif /* MBEDTLS_AES_C */
Generated on Sun Jul 17 2022 08:25:18 by
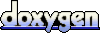