
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
add_del.cpp
Go to the documentation of this file.
00001 /* 00002 * mbed Microcontroller Library 00003 * Copyright (c) 2006-2016 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 * 00017 */ 00018 00019 /** @file add_del.cpp Test cases to add and delete key-value pairs in the CFSTORE. 00020 * 00021 * Please consult the documentation under the test-case functions for 00022 * a description of the individual test case. 00023 */ 00024 00025 #include "mbed.h" 00026 #include "cfstore_config.h" 00027 #include "Driver_Common.h" 00028 #include "cfstore_debug.h" 00029 #include "cfstore_test.h" 00030 #include "configuration_store.h" 00031 #include "utest/utest.h" 00032 #include "unity/unity.h" 00033 #include "greentea-client/test_env.h" 00034 #include "cfstore_utest.h" 00035 00036 #ifdef YOTTA_CFG_CFSTORE_UVISOR 00037 #include "uvisor-lib/uvisor-lib.h" 00038 #include "cfstore_uvisor.h" 00039 #endif /* YOTTA_CFG_CFSTORE_UVISOR */ 00040 00041 #include <stdio.h> 00042 #include <stdlib.h> 00043 #include <string.h> 00044 #include <inttypes.h> 00045 00046 using namespace utest::v1; 00047 00048 #define CFSTORE_ADD_DEL_MALLOC_SIZE 1024 00049 00050 static char cfstore_add_del_utest_msg_g[CFSTORE_UTEST_MSG_BUF_SIZE]; 00051 00052 #ifdef YOTTA_CFG_CFSTORE_UVISOR 00053 /* Create the main box ACL list for the application. 00054 * The main ACL gets inherited by all the other boxes 00055 */ 00056 CFSTORE_UVISOR_MAIN_ACL(cfstore_acl_uvisor_box_add_del_g); 00057 00058 /* Enable uVisor. */ 00059 UVISOR_SET_MODE_ACL(UVISOR_ENABLED, cfstore_acl_uvisor_box_add_del_g); 00060 #endif /* YOTTA_CFG_CFSTORE_UVISOR */ 00061 00062 static cfstore_kv_data_t cfstore_add_del_test_07_data[] = { 00063 CFSTORE_INIT_1_TABLE_MID_NODE, 00064 { NULL, NULL}, 00065 }; 00066 00067 00068 /* report whether built/configured for flash sync or async mode */ 00069 static control_t cfstore_add_del_test_00(const size_t call_count) 00070 { 00071 int32_t ret = ARM_DRIVER_ERROR; 00072 00073 (void) call_count; 00074 ret = cfstore_test_startup(); 00075 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to perform test startup (ret=%d).\n", __func__, (int) ret); 00076 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00077 return CaseNext; 00078 } 00079 00080 /** @brief 00081 * 00082 * This test case does the following: 00083 * - creates a KV. 00084 * - deletes the KV. 00085 * - checks that the deleted KV can no longer be found in the store. 00086 * 00087 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00088 */ 00089 control_t cfstore_add_del_test_01_end(const size_t call_count) 00090 { 00091 bool bfound = false; 00092 int32_t ret = ARM_DRIVER_ERROR; 00093 ARM_CFSTORE_SIZE len = 0; 00094 ARM_CFSTORE_DRIVER* drv = &cfstore_driver; 00095 ARM_CFSTORE_KEYDESC kdesc; 00096 ARM_CFSTORE_HANDLE_INIT(hkey); 00097 ARM_CFSTORE_FMODE flags; 00098 00099 CFSTORE_FENTRYLOG("%s:entered\n", __func__); 00100 (void) call_count; 00101 memset(&kdesc, 0, sizeof(kdesc)); 00102 memset(&flags, 0, sizeof(flags)); 00103 00104 kdesc.drl = ARM_RETENTION_WHILE_DEVICE_ACTIVE; 00105 len = strlen(cfstore_add_del_test_07_data[0].value); 00106 00107 ret = cfstore_test_create(cfstore_add_del_test_07_data[0].key_name, (char*) cfstore_add_del_test_07_data[0].value, &len, &kdesc); 00108 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to create KV in store (ret=%d).\n", __func__, (int) ret); 00109 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00110 00111 /* now delete KV*/ 00112 ret = drv->Open(cfstore_add_del_test_07_data[0].key_name, flags, hkey); 00113 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to Open() (ret=%d).\n", __func__, (int) ret); 00114 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00115 00116 if(hkey != NULL){ 00117 ret = drv->Delete(hkey); 00118 drv->Close(hkey); 00119 hkey = NULL; 00120 } 00121 /* check that the KV has been deleted */ 00122 /* revert to CFSTORE_LOG if more trace required */ 00123 CFSTORE_DBGLOG("LOG: WARNING: About to look for non-existent key (key_name=%s) (which will generate internal trace reporting errors if debug trace enabled).\n", cfstore_add_del_test_07_data[0].key_name); 00124 ret = cfstore_test_kv_is_found(cfstore_add_del_test_07_data[0].key_name, &bfound); 00125 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error failed to delete a key (ret=%d).\n", __func__, (int) ret); 00126 TEST_ASSERT_MESSAGE(ret == ARM_CFSTORE_DRIVER_ERROR_KEY_NOT_FOUND, cfstore_add_del_utest_msg_g); 00127 00128 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Test failed: found KV that was previously deleted (key_name=%s)\n", __func__, cfstore_add_del_test_07_data[0].key_name); 00129 TEST_ASSERT_MESSAGE(bfound == false, cfstore_add_del_utest_msg_g); 00130 00131 ret = drv->Uninitialize(); 00132 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Uninitialize() call failed.\n", __func__); 00133 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00134 return CaseNext; 00135 } 00136 00137 00138 static cfstore_kv_data_t cfstore_add_del_test_08_data[] = { 00139 CFSTORE_INIT_1_TABLE_HEAD, 00140 CFSTORE_INIT_1_TABLE_MID_NODE, 00141 CFSTORE_INIT_1_TABLE_TAIL, 00142 { NULL, NULL}, 00143 }; 00144 00145 00146 /** @brief 00147 * 00148 * This test case adds a small number of KVs (~3), and then delete them. 00149 * - add key(s) 00150 * - delete key(s) 00151 * - make sure can't find key in cfstore 00152 * - loop over the above a number of times. 00153 * 00154 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00155 */ 00156 control_t cfstore_add_del_test_02_end(const size_t call_count) 00157 { 00158 bool bResult = true; // We'll do "&=" cumulative checking. 00159 int32_t ret = ARM_DRIVER_ERROR; 00160 ARM_CFSTORE_SIZE len = 0; 00161 ARM_CFSTORE_KEYDESC kdesc; 00162 cfstore_kv_data_t* node = NULL; 00163 ARM_CFSTORE_DRIVER* drv = &cfstore_driver; 00164 00165 CFSTORE_FENTRYLOG("%s:entered\n", __func__); 00166 (void) call_count; 00167 memset(&kdesc, 0, sizeof(kdesc)); 00168 00169 /* create */ 00170 kdesc.drl = ARM_RETENTION_WHILE_DEVICE_ACTIVE; 00171 node = cfstore_add_del_test_08_data; 00172 while(node->key_name != NULL) 00173 { 00174 len = strlen(node->value); 00175 ret = cfstore_test_create(node->key_name, (char*) node->value, &len, &kdesc); 00176 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to create kv (key_name=%s.\n", __func__, node->key_name); 00177 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00178 /* revert CFSTORE_LOG for more trace */ 00179 CFSTORE_DBGLOG("Created KV successfully (key_name=\"%s\", value=\"%s\")\n", node->key_name, node->value); 00180 node++; 00181 } 00182 00183 /* test delete all */ 00184 ret = cfstore_test_delete_all(); 00185 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to delete all KVs.\n", __func__); 00186 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00187 00188 /* check there are no KVs present as expected */ 00189 node = cfstore_add_del_test_08_data; 00190 while(node->key_name != NULL) 00191 { 00192 ret = cfstore_test_kv_is_found(node->key_name, &bResult); 00193 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: found key when should not be present.\n", __func__); 00194 TEST_ASSERT_MESSAGE(ret == ARM_CFSTORE_DRIVER_ERROR_KEY_NOT_FOUND && bResult == false, cfstore_add_del_utest_msg_g); 00195 /* revert CFSTORE_LOG for more trace */ 00196 CFSTORE_DBGLOG("Found KV successfully (key_name=\"%s\")\n", node->key_name); 00197 node++; 00198 } 00199 ret = drv->Uninitialize(); 00200 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Uninitialize() call failed.\n", __func__); 00201 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00202 return CaseNext; 00203 } 00204 00205 /** @brief 00206 * 00207 * This test case adds ~50 KVs, and then delete entries at the start, 00208 * middle and end of list. 00209 * 00210 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00211 */ 00212 control_t cfstore_add_del_test_03_end(const size_t call_count) 00213 { 00214 bool bfound = false; 00215 int32_t ret = ARM_DRIVER_ERROR; 00216 ARM_CFSTORE_FMODE flags; 00217 cfstore_kv_data_t *node; 00218 ARM_CFSTORE_DRIVER* drv = &cfstore_driver; 00219 00220 CFSTORE_FENTRYLOG("%s:entered\n", __func__); 00221 (void) call_count; 00222 memset(&flags, 0, sizeof(flags)); 00223 00224 ret = cfstore_test_init_1(); 00225 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to initialise cfstore area with entries\n", __func__); 00226 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00227 00228 /* delete some keys */ 00229 node = cfstore_add_del_test_08_data; 00230 while(node->key_name != NULL) 00231 { 00232 CFSTORE_DBGLOG("%s:about to delete key (key_name=%s).\n", __func__, node->key_name); 00233 cfstore_test_delete(node->key_name); 00234 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error failed to delete a key (ret=%d).\n", __func__, (int) ret); 00235 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00236 /* revert CFSTORE_LOG for more trace */ 00237 CFSTORE_DBGLOG("Deleted KV successfully (key_name=\"%s\")\n", node->key_name); 00238 node++; 00239 } 00240 /* check the keys have been deleted */ 00241 node = cfstore_add_del_test_08_data; 00242 while(node->key_name != NULL) 00243 { 00244 ret = cfstore_test_kv_is_found(node->key_name, &bfound); 00245 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to delete a key (ret=%d).\n", __func__, (int) ret); 00246 TEST_ASSERT_MESSAGE(ret == ARM_CFSTORE_DRIVER_ERROR_KEY_NOT_FOUND, cfstore_add_del_utest_msg_g); 00247 00248 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Test failed: found KV that was previously deleted (key_name=%s)\n", __func__, node->key_name); 00249 TEST_ASSERT_MESSAGE(bfound == false, cfstore_add_del_utest_msg_g); 00250 node++; 00251 } 00252 00253 /* clean up by deleting all remaining KVs. this is not part of the test */ 00254 ret = cfstore_test_delete_all(); 00255 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error failed to delete a all KVs (ret=%d).\n", __func__, (int) ret); 00256 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00257 00258 ret = drv->Uninitialize(); 00259 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to initialize CFSTORE (ret=%d)\n", __func__, (int) ret); 00260 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00261 return CaseNext; 00262 } 00263 00264 00265 /** @brief 00266 * 00267 * This test case is as per test_03 but using delete_all() on all init_1 data. 00268 * This test case is yet to be implemented. 00269 * 00270 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00271 */ 00272 control_t cfstore_add_del_test_04(const size_t call_count) 00273 { 00274 (void) call_count; 00275 /*todo: implement test */ 00276 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Warn: Not implemented\n", __func__); 00277 CFSTORE_DBGLOG("%s: WARN: requires implementation\n", __func__); 00278 TEST_ASSERT_MESSAGE(true, cfstore_add_del_utest_msg_g); 00279 return CaseNext; 00280 } 00281 00282 /** @brief Delete an attribute after an internal realloc of the cfstore memory area 00283 * 00284 * This test case goes through the following steps: 00285 * 1. Creates attribute att_1 of size x, and write some data. This causes an internal 00286 * cfstore realloc to allocate heap memory for the attribute. 00287 * 2. Allocates some memory on the heap. Typically, this will be immediately after the 00288 * memory used by cfstore for the KV area. This means that if any cfstore reallocs are 00289 * made to increase size the memory area will have to move. 00290 * 3. Creates attribute att_2 of size y. This causes an internal cfstore realloc to move 00291 * the KV memory area to a new location. 00292 * 4. Delete att_1. This causes an internal realloc to shrink the area and tests that the 00293 * internal data structures that contain pointers to different parts of the KV area 00294 * are updated correctly. 00295 * 5. Allocates some memory on the heap. If the heap has been corrupted, this will likely trigger 00296 * a crash 00297 * 00298 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00299 */ 00300 control_t cfstore_add_del_test_05_end(const size_t call_count) 00301 { 00302 char data[] = "some test data"; 00303 char filename[] = "file1"; 00304 char filename2[] = "file2"; 00305 int32_t ret = ARM_DRIVER_ERROR; 00306 void *test_buf1 = NULL; 00307 void *test_buf2 = NULL; 00308 ARM_CFSTORE_DRIVER *cfstoreDriver = &cfstore_driver; 00309 ARM_CFSTORE_KEYDESC keyDesc1; 00310 ARM_CFSTORE_HANDLE_INIT(hkey1); 00311 ARM_CFSTORE_KEYDESC keyDesc2; 00312 ARM_CFSTORE_HANDLE_INIT(hkey2); 00313 ARM_CFSTORE_SIZE count = sizeof(data); 00314 00315 CFSTORE_FENTRYLOG("%s:entered\n", __func__); 00316 (void) call_count; 00317 00318 /* step 1 */ 00319 memset(&keyDesc1, 0, sizeof(keyDesc1)); 00320 keyDesc1.drl = ARM_RETENTION_NVM; 00321 keyDesc1.flags.read = true; 00322 keyDesc1.flags.write = true; 00323 ret = cfstoreDriver->Create(filename, 1024, &keyDesc1, hkey1); 00324 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to create attribute 1 (ret=%d)\n", __func__, (int) ret); 00325 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00326 00327 /* Write data to file */ 00328 ret = cfstoreDriver->Write(hkey1, (const char *)data, &count); 00329 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to write to attribute 1 (ret=%d)\n", __func__, (int) ret); 00330 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00331 00332 /* step 2 */ 00333 test_buf1 = malloc(CFSTORE_ADD_DEL_MALLOC_SIZE); 00334 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to allocate memory (test_buf1=%p)\n", __func__, test_buf1); 00335 TEST_ASSERT_MESSAGE(test_buf1 != NULL, cfstore_add_del_utest_msg_g); 00336 00337 /* step 3 */ 00338 memset(&keyDesc2, 0, sizeof(keyDesc2)); 00339 keyDesc2.drl = ARM_RETENTION_NVM; 00340 keyDesc2.flags.read = true; 00341 keyDesc2.flags.write = true; 00342 ret = cfstoreDriver->Create(filename2, 1024, &keyDesc2, hkey2); 00343 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to create attribute 2 (ret=%d)\n", __func__, (int) ret); 00344 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00345 00346 /* Write data to file */ 00347 count = sizeof(data); 00348 ret = cfstoreDriver->Write(hkey2, (const char *)data, &count); 00349 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to write to attribute 2 (ret=%d)\n", __func__, (int) ret); 00350 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00351 00352 /* step 4 */ 00353 ret = cfstoreDriver->Delete(hkey1); 00354 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to delete to attribute 1 (ret=%d)\n", __func__, (int) ret); 00355 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00356 00357 ret = cfstoreDriver->Close(hkey1); 00358 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to close to attribute 1 (ret=%d)\n", __func__, (int) ret); 00359 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00360 00361 /* step 5 */ 00362 test_buf2 = malloc(CFSTORE_ADD_DEL_MALLOC_SIZE); 00363 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to allocate memory (test_buf2=%p)\n", __func__, test_buf2); 00364 TEST_ASSERT_MESSAGE(test_buf2 != NULL, cfstore_add_del_utest_msg_g); 00365 00366 /* clean up */ 00367 ret = cfstoreDriver->Close(hkey2); 00368 CFSTORE_TEST_UTEST_MESSAGE(cfstore_add_del_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to close to attribute 2 (ret=%d)\n", __func__, (int) ret); 00369 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_add_del_utest_msg_g); 00370 free(test_buf2); 00371 free(test_buf1); 00372 00373 return CaseNext; 00374 } 00375 00376 /// @cond CFSTORE_DOXYGEN_DISABLE 00377 utest::v1::status_t greentea_setup(const size_t number_of_cases) 00378 { 00379 GREENTEA_SETUP(300, "default_auto"); 00380 return greentea_test_setup_handler(number_of_cases); 00381 } 00382 00383 Case cases[] = { 00384 /* 1 2 3 4 5 6 7 */ 00385 /* 1234567890123456789012345678901234567890123456789012345678901234567890 */ 00386 Case("ADD_DEL_test_00", cfstore_add_del_test_00), 00387 Case("ADD_DEL_test_01_start", cfstore_utest_default_start), 00388 Case("ADD_DEL_test_01_end", cfstore_add_del_test_01_end), 00389 Case("ADD_DEL_test_02_start", cfstore_utest_default_start), 00390 Case("ADD_DEL_test_02_end", cfstore_add_del_test_02_end), 00391 Case("ADD_DEL_test_03_start", cfstore_utest_default_start), 00392 Case("ADD_DEL_test_03_end", cfstore_add_del_test_03_end), 00393 Case("ADD_DEL_test_04", cfstore_add_del_test_04), 00394 Case("ADD_DEL_test_05_start", cfstore_utest_default_start), 00395 Case("ADD_DEL_test_05_end", cfstore_add_del_test_05_end), 00396 }; 00397 00398 00399 /* Declare your test specification with a custom setup handler */ 00400 Specification specification(greentea_setup, cases); 00401 00402 int main() 00403 { 00404 return !Harness::run(specification); 00405 } 00406 /// @endcond
Generated on Sun Jul 17 2022 08:25:18 by
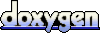