
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
TMP102.h
00001 #ifndef TMP102_H 00002 #define TMP102_H 00003 00004 #include "mbed.h" 00005 00006 //!Library for the TI TMP102 temperature sensor. 00007 /*! 00008 The TMP102 is an I2C digital temperature sensor in a small SOT563 package, with a 0.0625C resolution and 0.5C accuracy. 00009 */ 00010 class TMP102 00011 { 00012 public: 00013 //!Creates an instance of the class. 00014 /*! 00015 Connect module at I2C address addr using I2C port pins sda and scl. 00016 TMP102 00017 \param addr <table><tr><th>A0 pin connection</th><th>Address</th></tr><tr><td>GND</td><td>0x90</td></tr><tr><td>V+</td><td>0x92</td></tr><tr><td>SDA</td><td>0x94</td></tr><tr><td>SCL</td><td>0x96</td></tr></table> 00018 */ 00019 TMP102(PinName sda, PinName scl, int addr); 00020 00021 /*! 00022 Destroys instance. 00023 */ 00024 ~TMP102 (); 00025 00026 //!Reads the current temperature. 00027 /*! 00028 Reads the temperature register of the TMP102 and converts it to a useable value. 00029 */ 00030 float read(); 00031 00032 I2C m_i2c; 00033 00034 private: 00035 int m_addr; 00036 00037 }; 00038 00039 #endif
Generated on Sun Jul 17 2022 08:25:32 by
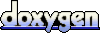