
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
PwmOut.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_PWMOUT_H 00017 #define MBED_PWMOUT_H 00018 00019 #include "platform/platform.h" 00020 00021 #if defined (DEVICE_PWMOUT) || defined(DOXYGEN_ONLY) 00022 #include "hal/pwmout_api.h" 00023 #include "platform/mbed_critical.h" 00024 #include "platform/mbed_sleep.h" 00025 00026 namespace mbed { 00027 /** \addtogroup drivers */ 00028 00029 /** A pulse-width modulation digital output 00030 * 00031 * @note Synchronization level: Interrupt safe 00032 * 00033 * Example 00034 * @code 00035 * // Fade a led on. 00036 * #include "mbed.h" 00037 * 00038 * PwmOut led(LED1); 00039 * 00040 * int main() { 00041 * while(1) { 00042 * led = led + 0.01; 00043 * wait(0.2); 00044 * if(led == 1.0) { 00045 * led = 0; 00046 * } 00047 * } 00048 * } 00049 * @endcode 00050 * @ingroup drivers 00051 */ 00052 class PwmOut { 00053 00054 public: 00055 00056 /** Create a PwmOut connected to the specified pin 00057 * 00058 * @param pin PwmOut pin to connect to 00059 */ 00060 PwmOut(PinName pin) : _deep_sleep_locked(false) { 00061 core_util_critical_section_enter(); 00062 pwmout_init(&_pwm, pin); 00063 core_util_critical_section_exit(); 00064 } 00065 00066 ~PwmOut() { 00067 core_util_critical_section_enter(); 00068 unlock_deep_sleep(); 00069 core_util_critical_section_exit(); 00070 } 00071 00072 /** Set the ouput duty-cycle, specified as a percentage (float) 00073 * 00074 * @param value A floating-point value representing the output duty-cycle, 00075 * specified as a percentage. The value should lie between 00076 * 0.0f (representing on 0%) and 1.0f (representing on 100%). 00077 * Values outside this range will be saturated to 0.0f or 1.0f. 00078 */ 00079 void write(float value) { 00080 core_util_critical_section_enter(); 00081 lock_deep_sleep(); 00082 pwmout_write(&_pwm, value); 00083 core_util_critical_section_exit(); 00084 } 00085 00086 /** Return the current output duty-cycle setting, measured as a percentage (float) 00087 * 00088 * @returns 00089 * A floating-point value representing the current duty-cycle being output on the pin, 00090 * measured as a percentage. The returned value will lie between 00091 * 0.0f (representing on 0%) and 1.0f (representing on 100%). 00092 * 00093 * @note 00094 * This value may not match exactly the value set by a previous write(). 00095 */ 00096 float read() { 00097 core_util_critical_section_enter(); 00098 float val = pwmout_read(&_pwm); 00099 core_util_critical_section_exit(); 00100 return val; 00101 } 00102 00103 /** Set the PWM period, specified in seconds (float), keeping the duty cycle the same. 00104 * 00105 * @param seconds Change the period of a PWM signal in seconds (float) without modifying the duty cycle 00106 * @note 00107 * The resolution is currently in microseconds; periods smaller than this 00108 * will be set to zero. 00109 */ 00110 void period(float seconds) { 00111 core_util_critical_section_enter(); 00112 pwmout_period(&_pwm, seconds); 00113 core_util_critical_section_exit(); 00114 } 00115 00116 /** Set the PWM period, specified in milli-seconds (int), keeping the duty cycle the same. 00117 * @param ms Change the period of a PWM signal in milli-seconds without modifying the duty cycle 00118 */ 00119 void period_ms(int ms) { 00120 core_util_critical_section_enter(); 00121 pwmout_period_ms(&_pwm, ms); 00122 core_util_critical_section_exit(); 00123 } 00124 00125 /** Set the PWM period, specified in micro-seconds (int), keeping the duty cycle the same. 00126 * @param us Change the period of a PWM signal in micro-seconds without modifying the duty cycle 00127 */ 00128 void period_us(int us) { 00129 core_util_critical_section_enter(); 00130 pwmout_period_us(&_pwm, us); 00131 core_util_critical_section_exit(); 00132 } 00133 00134 /** Set the PWM pulsewidth, specified in seconds (float), keeping the period the same. 00135 * @param seconds Change the pulse width of a PWM signal specified in seconds (float) 00136 */ 00137 void pulsewidth(float seconds) { 00138 core_util_critical_section_enter(); 00139 pwmout_pulsewidth(&_pwm, seconds); 00140 core_util_critical_section_exit(); 00141 } 00142 00143 /** Set the PWM pulsewidth, specified in milli-seconds (int), keeping the period the same. 00144 * @param ms Change the pulse width of a PWM signal specified in milli-seconds 00145 */ 00146 void pulsewidth_ms(int ms) { 00147 core_util_critical_section_enter(); 00148 pwmout_pulsewidth_ms(&_pwm, ms); 00149 core_util_critical_section_exit(); 00150 } 00151 00152 /** Set the PWM pulsewidth, specified in micro-seconds (int), keeping the period the same. 00153 * @param us Change the pulse width of a PWM signal specified in micro-seconds 00154 */ 00155 void pulsewidth_us(int us) { 00156 core_util_critical_section_enter(); 00157 pwmout_pulsewidth_us(&_pwm, us); 00158 core_util_critical_section_exit(); 00159 } 00160 00161 /** A operator shorthand for write() 00162 * \sa PwmOut::write() 00163 */ 00164 PwmOut& operator= (float value) { 00165 // Underlying call is thread safe 00166 write(value); 00167 return *this; 00168 } 00169 00170 /** A operator shorthand for write() 00171 * \sa PwmOut::write() 00172 */ 00173 PwmOut& operator= (PwmOut& rhs) { 00174 // Underlying call is thread safe 00175 write(rhs.read()); 00176 return *this; 00177 } 00178 00179 /** An operator shorthand for read() 00180 * \sa PwmOut::read() 00181 */ 00182 operator float() { 00183 // Underlying call is thread safe 00184 return read(); 00185 } 00186 00187 protected: 00188 /** Lock deep sleep only if it is not yet locked */ 00189 void lock_deep_sleep() { 00190 if (_deep_sleep_locked == false) { 00191 sleep_manager_lock_deep_sleep(); 00192 _deep_sleep_locked = true; 00193 } 00194 } 00195 00196 /** Unlock deep sleep in case it is locked */ 00197 void unlock_deep_sleep() { 00198 if (_deep_sleep_locked == true) { 00199 sleep_manager_unlock_deep_sleep(); 00200 _deep_sleep_locked = false; 00201 } 00202 } 00203 00204 pwmout_t _pwm; 00205 bool _deep_sleep_locked; 00206 }; 00207 00208 } // namespace mbed 00209 00210 #endif 00211 00212 #endif
Generated on Sun Jul 17 2022 08:25:29 by
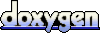