
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
LPC.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 00017 00018 http://www.nxp.com/documents/user_manual/UM10360.pdf 00019 00020 32.3.1.1 Criterion for Valid User Code 00021 The reserved Cortex-M3 exception vector location 7 (offset 0x1C in the vector table) 00022 should contain the 2's complement of the check-sum of table entries 0 through 6. This 00023 causes the checksum of the first 8 table entries to be 0. The boot loader code checksums 00024 the first 8 locations in sector 0 of the flash. If the result is 0, then execution control is 00025 transferred to the user code. 00026 """ 00027 from struct import unpack, pack 00028 00029 00030 def patch(bin_path): 00031 with open(bin_path, 'r+b') as bin: 00032 # Read entries 0 through 6 (Little Endian 32bits words) 00033 vector = [unpack('<I', bin.read(4))[0] for _ in range(7)] 00034 00035 # location 7 (offset 0x1C in the vector table) should contain the 2's 00036 # complement of the check-sum of table entries 0 through 6 00037 bin.seek(0x1C) 00038 bin.write(pack('<I', (~sum(vector) + 1) & 0xFFFFFFFF)) 00039 00040 00041 def is_patched(bin_path): 00042 with open(bin_path, 'rb') as bin: 00043 # The checksum of the first 8 table entries should be 0 00044 return (sum([unpack('<I', bin.read(4))[0] for _ in range(8)]) & 0xFFFFFFFF) == 0 00045 00046 00047 if __name__ == '__main__': 00048 bin_path = "C:/Users/emimon01/releases/emilmont/build/test/LPC1768/ARM/MBED_A1/basic.bin" 00049 patch(bin_path) 00050 assert is_patched(bin_path), "The file is not patched"
Generated on Sun Jul 17 2022 08:25:24 by
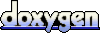