
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
FileBase.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_FILEBASE_H 00017 #define MBED_FILEBASE_H 00018 00019 typedef int FILEHANDLE; 00020 00021 #include <cstdio> 00022 #include <cstring> 00023 00024 #include "platform/platform.h" 00025 #include "platform/SingletonPtr.h" 00026 #include "platform/PlatformMutex.h" 00027 #include "platform/NonCopyable.h" 00028 00029 namespace mbed { 00030 00031 typedef enum { 00032 FilePathType, 00033 FileSystemPathType 00034 } PathType; 00035 00036 /** \addtogroup platform */ 00037 /** @{*/ 00038 /** 00039 * \defgroup platform_FileBase FileBase class 00040 * @{ 00041 */ 00042 /** Class FileBase 00043 * 00044 */ 00045 00046 class FileBase : private NonCopyable<FileBase> { 00047 public: 00048 FileBase(const char *name, PathType t); 00049 virtual ~FileBase(); 00050 00051 const char* getName(void); 00052 PathType getPathType(void); 00053 00054 static FileBase *lookup(const char *name, unsigned int len); 00055 00056 static FileBase *get(int n); 00057 00058 /* disallow copy constructor and assignment operators */ 00059 private: 00060 static FileBase *_head; 00061 static SingletonPtr<PlatformMutex> _mutex; 00062 00063 FileBase *_next; 00064 const char * const _name; 00065 const PathType _path_type; 00066 }; 00067 00068 /**@}*/ 00069 00070 /**@}*/ 00071 00072 } // namespace mbed 00073 00074 #endif 00075
Generated on Sun Jul 17 2022 08:25:23 by
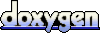