
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
EventQueue.cpp
00001 /* events 00002 * Copyright (c) 2016 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "events/EventQueue.h" 00017 00018 #include "events/mbed_events.h" 00019 #include "mbed.h" 00020 00021 00022 EventQueue::EventQueue(unsigned event_size, unsigned char *event_pointer) { 00023 if (!event_pointer) { 00024 equeue_create(&_equeue, event_size); 00025 } else { 00026 equeue_create_inplace(&_equeue, event_size, event_pointer); 00027 } 00028 } 00029 00030 EventQueue::~EventQueue() { 00031 equeue_destroy(&_equeue); 00032 } 00033 00034 void EventQueue::dispatch(int ms) { 00035 return equeue_dispatch(&_equeue, ms); 00036 } 00037 00038 void EventQueue::break_dispatch() { 00039 return equeue_break(&_equeue); 00040 } 00041 00042 unsigned EventQueue::tick() { 00043 return equeue_tick(); 00044 } 00045 00046 void EventQueue::cancel(int id) { 00047 return equeue_cancel(&_equeue, id); 00048 } 00049 00050 void EventQueue::background(Callback<void(int)> update) { 00051 _update = update; 00052 00053 if (_update) { 00054 equeue_background(&_equeue, &Callback<void(int)>::thunk, &_update); 00055 } else { 00056 equeue_background(&_equeue, 0, 0); 00057 } 00058 } 00059 00060 void EventQueue::chain(EventQueue *target) { 00061 if (target) { 00062 equeue_chain(&_equeue, &target->_equeue); 00063 } else { 00064 equeue_chain(&_equeue, 0); 00065 } 00066 }
Generated on Sun Jul 17 2022 08:25:22 by
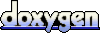