
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
DiscoveredService.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MBED_DISCOVERED_SERVICE_H__ 00018 #define MBED_DISCOVERED_SERVICE_H__ 00019 00020 #include "UUID.h " 00021 #include "GattAttribute.h" 00022 00023 /** 00024 * @addtogroup ble 00025 * @{ 00026 * @addtogroup gatt 00027 * @{ 00028 * @addtogroup client 00029 * @{ 00030 */ 00031 00032 /** 00033 * Representation of a GATT service discovered. 00034 * 00035 * The discovery procedure discovers GATT Services are discovered on distant 00036 * GATT servers, which can be initiated by calling 00037 * GattClient::launchServiceDiscovery() or GattClient::discoverServices(). The 00038 * discovery process passes instances of this class to the callback handling 00039 * service discovered. 00040 * 00041 * Discovered services are characterized by the UUID of the service discovered 00042 * and the range of the GATT attributes belonging to the service. 00043 * 00044 * The UUID can be queried by calling getUUID() while the begining of the 00045 * attribute range can be obtained through getStartHandle() and the end of the 00046 * attribute range with a call to getEndHandle(). 00047 * 00048 * The characteristics composing the service may be discovered by the function 00049 * GattClient::launchServiceDiscovery(). 00050 */ 00051 class DiscoveredService { 00052 public: 00053 /** 00054 * Get the UUID of the discovered service. 00055 * 00056 * @return A reference to the UUID of the discovered service. 00057 */ 00058 const UUID &getUUID(void) const 00059 { 00060 return uuid; 00061 } 00062 00063 /** 00064 * Get the start handle of the discovered service in the peer's GATT server. 00065 * 00066 * @return A reference to the start handle. 00067 */ 00068 const GattAttribute::Handle_t& getStartHandle(void) const 00069 { 00070 return startHandle; 00071 } 00072 00073 /** 00074 * Get the end handle of the discovered service in the peer's GATT server. 00075 * 00076 * @return A reference to the end handle. 00077 */ 00078 const GattAttribute::Handle_t& getEndHandle(void) const 00079 { 00080 return endHandle; 00081 } 00082 00083 public: 00084 /** 00085 * Construct a DiscoveredService instance. 00086 * 00087 * @important This API is not meant to be used publicly. It is meant to be 00088 * used by internal APIs of Mbed BLE. 00089 */ 00090 DiscoveredService() : 00091 uuid(UUID::ShortUUIDBytes_t(0)), 00092 startHandle(GattAttribute::INVALID_HANDLE), 00093 endHandle(GattAttribute::INVALID_HANDLE) { 00094 } 00095 00096 /** 00097 * Set information about the discovered service. 00098 * 00099 * @important This API is not meant to be used publicly. It is meant to be 00100 * used by internal APIs of Mbed BLE. 00101 * 00102 * @param[in] uuidIn The UUID of the discovered service. 00103 * @param[in] startHandleIn The start handle of the discovered service in 00104 * the peer's GATT server. 00105 * @param[in] endHandleIn The end handle of the discovered service in the 00106 * peer's GATT server. 00107 */ 00108 void setup( 00109 UUID uuidIn, 00110 GattAttribute::Handle_t startHandleIn, 00111 GattAttribute::Handle_t endHandleIn 00112 ) { 00113 uuid = uuidIn; 00114 startHandle = startHandleIn; 00115 endHandle = endHandleIn; 00116 } 00117 00118 /** 00119 * Set the start and end handle of the discovered service. 00120 * 00121 * @important This API is not meant to be used publicly. It is meant to be 00122 * used by internal APIs of Mbed BLE. 00123 * 00124 * @param[in] startHandleIn The start handle of the discovered service in 00125 * the peer's GATT server. 00126 * @param[in] endHandleIn The end handle of the discovered service in the 00127 * peer's GATT server. 00128 */ 00129 void setup( 00130 GattAttribute::Handle_t startHandleIn, 00131 GattAttribute::Handle_t endHandleIn 00132 ) { 00133 startHandle = startHandleIn; 00134 endHandle = endHandleIn; 00135 } 00136 00137 /** 00138 * Set the long UUID of the discovered service. 00139 * 00140 * @important This API is not meant to be used publicly. It is meant to be 00141 * used by internal APIs of Mbed BLE. 00142 * 00143 * @param[in] longUUID The bytes composing the long UUID of this discovered 00144 * service. 00145 * @param[in] order The byte ordering of @p longUUID. 00146 */ 00147 void setupLongUUID( 00148 UUID::LongUUIDBytes_t longUUID, 00149 UUID::ByteOrder_t order = UUID::MSB 00150 ) { 00151 uuid.setupLong(longUUID, order); 00152 } 00153 00154 00155 private: 00156 DiscoveredService(const DiscoveredService &); 00157 00158 private: 00159 /** 00160 * UUID of the service. 00161 */ 00162 UUID uuid; 00163 00164 /** 00165 * Begining of the Service Handle Range. 00166 */ 00167 GattAttribute::Handle_t startHandle; 00168 00169 /** 00170 * Service Handle Range. 00171 */ 00172 GattAttribute::Handle_t endHandle; 00173 }; 00174 00175 /** 00176 * @} 00177 * @} 00178 * @} 00179 */ 00180 00181 #endif /* MBED_DISCOVERED_SERVICE_H__ */
Generated on Sun Jul 17 2022 08:25:21 by
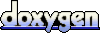