
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
CriticalSectionLock.h
00001 /* 00002 * PackageLicenseDeclared: Apache-2.0 00003 * Copyright (c) 2017 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #ifndef MBED_CRITICALSECTIONLOCK_H 00019 #define MBED_CRITICALSECTIONLOCK_H 00020 00021 #include "platform/mbed_critical.h" 00022 00023 namespace mbed { 00024 00025 /** \addtogroup platform */ 00026 /** @{*/ 00027 /** 00028 * \defgroup platform_CriticalSectionLock CriticalSectionLock functions 00029 * @{ 00030 */ 00031 00032 /** RAII object for disabling, then restoring, interrupt state 00033 * Usage: 00034 * @code 00035 * 00036 * void f() { 00037 * // some code here 00038 * { 00039 * CriticalSectionLock lock; 00040 * // Code in this block will run with interrupts disabled 00041 * } 00042 * // interrupts will be restored to their previous state 00043 * } 00044 * @endcode 00045 */ 00046 class CriticalSectionLock { 00047 public: 00048 CriticalSectionLock() 00049 { 00050 core_util_critical_section_enter(); 00051 } 00052 00053 ~CriticalSectionLock() 00054 { 00055 core_util_critical_section_exit(); 00056 } 00057 00058 /** Mark the start of a critical section 00059 * 00060 */ 00061 void lock() 00062 { 00063 core_util_critical_section_enter(); 00064 } 00065 00066 /** Mark the end of a critical section 00067 * 00068 */ 00069 void unlock() 00070 { 00071 core_util_critical_section_exit(); 00072 } 00073 }; 00074 00075 /**@}*/ 00076 00077 /**@}*/ 00078 00079 } // namespace mbed 00080 00081 #endif
Generated on Sun Jul 17 2022 08:25:21 by
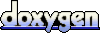