
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
C12832.h
00001 /* mbed library for the mbed Lab Board 128*32 pixel LCD 00002 * use C12832 controller 00003 * Copyright (c) 2012 Peter Drescher - DC2PD 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 * 00006 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00007 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00008 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00009 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00010 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00011 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00012 * THE SOFTWARE. 00013 */ 00014 00015 #ifndef C12832_H 00016 #define C12832_H 00017 00018 #include "mbed.h" 00019 #include "GraphicsDisplay.h" 00020 00021 00022 /** 00023 * Optional Defines: 00024 * #define debug_lcd 1 enable infos to PC_USB 00025 */ 00026 00027 // some defines for the DMA use 00028 #define DMA_CHANNEL_ENABLE 1 00029 #define DMA_TRANSFER_TYPE_M2P (1UL << 11) 00030 #define DMA_CHANNEL_TCIE (1UL << 31) 00031 #define DMA_CHANNEL_SRC_INC (1UL << 26) 00032 #define DMA_MASK_IE (1UL << 14) 00033 #define DMA_MASK_ITC (1UL << 15) 00034 #define DMA_SSP1_TX (1UL << 2) 00035 #define DMA_SSP0_TX (0) 00036 #define DMA_DEST_SSP1_TX (2UL << 6) 00037 #define DMA_DEST_SSP0_TX (0UL << 6) 00038 00039 /** 00040 * Draw mode 00041 * NORMAL 00042 * XOR set pixel by xor the screen 00043 */ 00044 enum {NORMAL,XOR}; 00045 00046 /** 00047 * Bitmap 00048 */ 00049 struct Bitmap{ 00050 int xSize; 00051 int ySize; 00052 int Byte_in_Line; 00053 char* data; 00054 }; 00055 00056 /** 00057 * The C12832 class 00058 */ 00059 class C12832 : public GraphicsDisplay 00060 { 00061 public: 00062 /** 00063 * Create a C12832 object connected to SPI1 00064 */ 00065 C12832(PinName mosi, PinName sck, PinName reset, PinName a0, PinName ncs, const char* name = "LCD"); 00066 00067 /** 00068 * Get the width of the screen in pixel 00069 * 00070 * @returns width of screen in pixel 00071 * 00072 */ 00073 virtual int width(); 00074 00075 /** 00076 * Get the height of the screen in pixel 00077 * 00078 * @returns height of screen in pixel 00079 */ 00080 virtual int height(); 00081 00082 /** 00083 * Draw a pixel at x,y black or white 00084 * 00085 * @param x horizontal position 00086 * @param y vertical position 00087 * @param color - 1 set pixel, 0 erase pixel 00088 */ 00089 virtual void pixel(int x, int y,int colour); 00090 00091 /** 00092 * Draw a circle 00093 * 00094 * @param x0,y0 center 00095 * @param r radius 00096 * @param color - 1 set pixel, 0 erase pixel 00097 */ 00098 void circle(int x, int y, int r, int colour); 00099 00100 /** 00101 * Draw a filled circle 00102 * 00103 * @param x0,y0 center 00104 * @param r radius 00105 * @param color - 1 set pixel, 0 erase pixel 00106 * 00107 * Use circle with different radius, 00108 * Can miss some pixels 00109 */ 00110 void fillcircle(int x, int y, int r, int colour); 00111 00112 /** 00113 * Draw a 1 pixel line 00114 * 00115 * @param x0,y0 start point 00116 * @param x1,y1 stop point 00117 * @param color - 1 set pixel, 0 erase pixel 00118 */ 00119 void line(int x0, int y0, int x1, int y1, int colour); 00120 00121 /** 00122 * Draw a rect 00123 * 00124 * @param x0,y0 top left corner 00125 * @param x1,y1 down right corner 00126 * @param color - 1 set pixel, 0 erase pixel 00127 */ 00128 void rect(int x0, int y0, int x1, int y1, int colour); 00129 00130 /** 00131 * Draw a filled rect 00132 * 00133 * @param x0,y0 top left corner 00134 * @param x1,y1 down right corner 00135 * @param color - 1 set pixel, 0 erase pixel 00136 */ 00137 void fillrect(int x0, int y0, int x1, int y1, int colour); 00138 00139 /** 00140 * Copy display buffer to LCD 00141 */ 00142 void copy_to_lcd(void); 00143 00144 /** 00145 * Set the orienation of the screen 00146 */ 00147 00148 void set_contrast(unsigned int o); 00149 00150 /** 00151 * Read the contrast level 00152 */ 00153 unsigned int get_contrast(void); 00154 00155 /** 00156 * Invert the screen 00157 * 00158 * @param o = 0 normal, 1 invert 00159 */ 00160 void invert(unsigned int o); 00161 00162 /** 00163 * Clear the screen 00164 */ 00165 virtual void cls(void); 00166 00167 /** 00168 * Set the drawing mode 00169 * 00170 * @param mode NORMAl or XOR 00171 */ 00172 void setmode(int mode); 00173 00174 virtual int columns(void); 00175 00176 /** 00177 * Calculate the max number of columns. 00178 * Depends on actual font size 00179 * 00180 * @returns max column 00181 */ 00182 virtual int rows(void); 00183 00184 /** 00185 * Put a char on the screen 00186 * 00187 * @param value char to print 00188 * @returns printed char 00189 */ 00190 virtual int _putc(int value); 00191 00192 /** 00193 * Draw a character on given position out of the active font to the LCD 00194 * 00195 * @param x x-position of char (top left) 00196 * @param y y-position 00197 * @param c char to print 00198 */ 00199 virtual void character(int x, int y, int c); 00200 00201 /** 00202 * Setup cursor position 00203 * 00204 * @param x x-position (top left) 00205 * @param y y-position 00206 */ 00207 virtual void locate(int x, int y); 00208 00209 /** 00210 * Setup auto update of screen 00211 * 00212 * @param up 1 = on , 0 = off 00213 * 00214 * if switched off the program has to call copy_to_lcd() 00215 * to update screen from framebuffer 00216 */ 00217 void set_auto_up(unsigned int up); 00218 00219 /** 00220 * Get status of the auto update function 00221 * 00222 * @returns if auto update is on 00223 */ 00224 unsigned int get_auto_up(void); 00225 00226 /** Vars */ 00227 SPI _spi; 00228 DigitalOut _reset; 00229 DigitalOut _A0; 00230 DigitalOut _CS; 00231 unsigned char* font; 00232 unsigned int draw_mode; 00233 00234 00235 /** 00236 * Select the font to use 00237 * 00238 * @param f pointer to font array 00239 * 00240 * font array can created with GLCD Font Creator from http://www.mikroe.com 00241 * you have to add 4 parameter at the beginning of the font array to use: 00242 * - the number of byte / char 00243 * - the vertial size in pixel 00244 * - the horizontal size in pixel 00245 * - the number of byte per vertical line 00246 * you also have to change the array to char[] 00247 */ 00248 void set_font(unsigned char* f); 00249 00250 /** 00251 * Print bitmap to buffer 00252 * 00253 * @param bm Bitmap in flash 00254 * @param x x start 00255 * @param y y start 00256 */ 00257 void print_bm(Bitmap bm, int x, int y); 00258 00259 protected: 00260 00261 /** 00262 * Draw a horizontal line 00263 * 00264 * @param x0 horizontal start 00265 * @param x1 horizontal stop 00266 * @param y vertical position 00267 * @param color - 1 set pixel, 0 erase pixel 00268 */ 00269 void hline(int x0, int x1, int y, int colour); 00270 00271 /** 00272 * Draw a vertical line 00273 * 00274 * @param x horizontal position 00275 * @param y0 vertical start 00276 * @param y1 vertical stop 00277 * @param color - 1 set pixel, 0 erase pixel 00278 */ 00279 void vline(int y0, int y1, int x, int colour); 00280 00281 /** 00282 * Init the C12832 LCD controller 00283 */ 00284 void lcd_reset(); 00285 00286 /** 00287 * Write data to the LCD controller 00288 * 00289 * @param dat data written to LCD controller 00290 */ 00291 void wr_dat(unsigned char value); 00292 00293 /** 00294 * Write a command the LCD controller 00295 * 00296 * @param cmd: command to be written 00297 */ 00298 void wr_cmd(unsigned char value); 00299 00300 void wr_cnt(unsigned char cmd); 00301 00302 unsigned int orientation; 00303 unsigned int char_x; 00304 unsigned int char_y; 00305 unsigned char buffer[512]; 00306 unsigned int contrast; 00307 unsigned int auto_up; 00308 00309 }; 00310 00311 00312 00313 00314 #endif
Generated on Sun Jul 17 2022 08:25:20 by
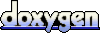