
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
AttServerMessage.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017-2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef BLE_PAL_ATT_SERVER_MESSAGE_H_ 00018 #define BLE_PAL_ATT_SERVER_MESSAGE_H_ 00019 00020 #include "ble/BLETypes.h" 00021 #include "ble/ArrayView.h " 00022 00023 namespace ble { 00024 namespace pal { 00025 00026 /** 00027 * Operation code defined for attribute operations 00028 * @note see: BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.8 00029 */ 00030 struct AttributeOpcode { 00031 enum Code { 00032 ERROR_RESPONSE = 0x01, /// Opcode of an AttErrorResponse 00033 EXCHANGE_MTU_REQUEST = 0x02, 00034 EXCHANGE_MTU_RESPONSE = 0x03, /// OpCode of an AttExchangeMTUResponse 00035 FIND_INFORMATION_REQUEST = 0x04, 00036 FIND_INFORMATION_RESPONSE = 0x05, /// OpCode of an AttFindInformationResponse 00037 FIND_BY_TYPE_VALUE_REQUEST = 0x06, 00038 FIND_BY_VALUE_TYPE_RESPONSE = 0x07, /// OpCode of an AttFindByTypeValueResponse 00039 READ_BY_TYPE_REQUEST = 0x08, 00040 READ_BY_TYPE_RESPONSE = 0x09, /// Opcode of an AttReadByTypeResponse 00041 READ_REQUEST = 0x0A, 00042 READ_RESPONSE = 0x0B, /// Opcode of an AttReadResponse 00043 READ_BLOB_REQUEST = 0x0C, 00044 READ_BLOB_RESPONSE = 0x0D, /// Opcode of an AttReadBlobResponse 00045 READ_MULTIPLE_REQUEST = 0x0E, 00046 READ_MULTIPLE_RESPONSE = 0x0F, /// Opcode of an AttReadMultipleResponse 00047 READ_BY_GROUP_TYPE_REQUEST = 0x10, 00048 READ_BY_GROUP_TYPE_RESPONSE = 0x11, /// Opcode of an AttReadByGroupTypeResponse 00049 WRITE_REQUEST = 0x12, 00050 WRITE_RESPONSE = 0x13, /// Opcode of an AttWriteResponse 00051 WRITE_COMMAND = 0x52, 00052 SIGNED_WRITE_COMMAND = 0xD2, 00053 PREPARE_WRITE_REQUEST = 0x16, 00054 PREPARE_WRITE_RESPONSE = 0x17, /// Opcode of an AttPrepareWriteResponse 00055 EXECUTE_WRITE_REQUEST = 0x18, 00056 EXECUTE_WRITE_RESPONSE = 0x19, /// Opcode of an AttExecuteWriteResponse 00057 HANDLE_VALUE_NOTIFICATION = 0x1B, 00058 HANDLE_VALUE_INDICATION = 0x1D 00059 }; 00060 00061 /** 00062 * Construct an AttributeOpcode from a Code. 00063 */ 00064 AttributeOpcode(Code value) : _value(value) { } 00065 00066 /** 00067 * Equality comparison operator between two AttributeOpcode 00068 */ 00069 friend bool operator==(AttributeOpcode lhs, AttributeOpcode rhs) { 00070 return lhs._value == rhs._value; 00071 } 00072 00073 /** 00074 * Non equality comparison operator between two AttributeOpcode 00075 */ 00076 friend bool operator!=(AttributeOpcode lhs, AttributeOpcode rhs) { 00077 return lhs._value != rhs._value; 00078 } 00079 00080 /** 00081 * implicit cast to uint8_t. 00082 * Allows AttributeOpcode to be used in switch statements. 00083 */ 00084 operator uint8_t() const { 00085 return _value; 00086 } 00087 00088 private: 00089 uint8_t _value; 00090 }; 00091 00092 00093 /** 00094 * Base class for Attribute Server Message. 00095 * The correct type of the instance can be determined with the attribute opcode. 00096 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.3.1 00097 */ 00098 struct AttServerMessage { 00099 /** 00100 * Op code used to identify the type of the attribute response. 00101 */ 00102 const AttributeOpcode opcode; 00103 00104 protected: 00105 /** 00106 * Construction of an AttResponse is reserved for descendent of the class 00107 */ 00108 AttServerMessage(AttributeOpcode opcode_) : opcode(opcode_) { } 00109 }; 00110 00111 00112 /** 00113 * Response to a request which can't be performed 00114 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.1.1 00115 * for details about error response. 00116 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.9 00117 * which details possible error response by requests. 00118 */ 00119 struct AttErrorResponse : public AttServerMessage { 00120 /** 00121 * Construct an attribute error response. 00122 * 00123 * @param request_opcode_ The Attribute opcode of the request that generated 00124 * the error. 00125 * @param handle_in_error_ The attribute handle that generated this error 00126 * response. 00127 * @param error_code The reason why the request has generated an error. 00128 */ 00129 AttErrorResponse( 00130 AttributeOpcode request_opcode_, 00131 attribute_handle_t handle_in_error_, 00132 uint8_t error_code_ 00133 ) : AttServerMessage(AttributeOpcode::ERROR_RESPONSE), 00134 request_opcode(request_opcode_), 00135 handle_in_error(handle_in_error_), error_code(error_code_) { 00136 } 00137 00138 /** 00139 * Construct an attribute error response in the case where there was no 00140 * attribute handle in the original response or if the request is not 00141 * supported. 00142 * 00143 * @param request_opcode_ The Attribute opcode of the request that generated 00144 * the error. 00145 * @param error_code The reason why the request has generated an error. 00146 */ 00147 AttErrorResponse( 00148 AttributeOpcode request_opcode_, 00149 uint8_t error_code_ 00150 ) : AttServerMessage(AttributeOpcode::ERROR_RESPONSE), 00151 request_opcode(request_opcode_), 00152 handle_in_error(0x0000), error_code(error_code_) { 00153 } 00154 00155 /** 00156 * The opcode of the request that generated this error response. 00157 */ 00158 const AttributeOpcode request_opcode; 00159 00160 /** 00161 * The attribute handle that generated this error response. 00162 * If there was no attribute handle in the original request or if the 00163 * request is not supported, then this field is equal to 0x0000. 00164 */ 00165 const attribute_handle_t handle_in_error; 00166 00167 /** 00168 * The reason why the request has generated an error response 00169 */ 00170 const uint8_t error_code; 00171 00172 /** 00173 * List of Error codes for the ATT protocol 00174 */ 00175 enum AttributeErrorCode { 00176 /** The attribute handle given was not valid on this server. */ 00177 INVALID_HANDLE = 0x01, 00178 00179 /** The attribute cannot be read. */ 00180 READ_NOT_PERMITTED = 0x02, 00181 00182 /** The attribute cannot be written. */ 00183 WRITE_NOT_PERMITTED = 0x03, 00184 00185 /** The attribute PDU was invalid. */ 00186 INVALID_PDU = 0x04, 00187 00188 /** The attribute requires authentication before it can be read or 00189 * written. 00190 */ 00191 INSUFFICIENT_AUTHENTICATION = 0x05, 00192 00193 /** Attribute server does not support the request received from the 00194 * client. 00195 */ 00196 REQUEST_NOT_SUPPORTED = 0x06, 00197 00198 /** Offset specified was past the end of the attribute. */ 00199 INVALID_OFFSET = 0x07, 00200 00201 /** The attribute requires authorization before it can be read or written. */ 00202 INSUFFICIENT_AUTHORIZATION = 0x08, 00203 00204 /** Too many prepare writes have been queued. */ 00205 PREPARE_QUEUE_FULL = 0x09, 00206 00207 /** No attribute found within the given attribute handle range. */ 00208 ATTRIBUTE_NOT_FOUND = 0x0A, 00209 00210 /** The attribute cannot be read using the Read Blob Request. */ 00211 ATTRIBUTE_NOT_LONG = 0x0B, 00212 00213 /** The Encryption Key Size used for encrypting this link is 00214 * insufficient. 00215 */ 00216 INSUFFICIENT_ENCRYPTION_KEY_SIZE = 0x0C, 00217 00218 /** The attribute value length is invalid for the operation. */ 00219 INVALID_ATTRIBUTE_VALUE_LENGTH = 0x0D, 00220 00221 /** The attribute request that was requested has encountered an error 00222 * that was unlikely, and therefore could not be completed as requested. 00223 */ 00224 UNLIKELY_ERROR = 0x0E, 00225 00226 /** The attribute requires encryption before it can be read or written. */ 00227 INSUFFICIENT_ENCRYPTION = 0x0F, 00228 00229 /** The attribute type is not a supported grouping attribute as defined 00230 * by a higher layer specification. 00231 */ 00232 UNSUPPORTED_GROUP_TYPE = 0x10, 00233 00234 /** Insufficient Resources to complete the request. */ 00235 INSUFFICIENT_RESOURCES = 0x11, 00236 00237 /* 0x12 - 0x7F => reserved for future use */ 00238 00239 /* 0x80 - 0x9F => Application Error */ 00240 00241 /* 0xA0 0xDF => Reserved for future use */ 00242 00243 /* 0xE0 - 0xFF Common Profile and service Error Codes */ 00244 00245 /** The Write Request Rejected error code is used when a requested write 00246 * operation cannot be fulfilled for reasons other than permissions. 00247 */ 00248 WRITE_REQUEST_REJECTED = 0xFC, 00249 00250 /** The Client Characteristic Configuration Descriptor Improperly 00251 * Configured error code is used when a Client Characteristic 00252 * Configuration descriptor is not configured according to the 00253 * requirements of the profile or service. 00254 */ 00255 CLIENT_CHARACTERISTIC_CONFIGURATION_DESCRIPTOR_IMPROPERLY_CONFIGURED = 0xFD, 00256 00257 /** The Procedure Already in Progress error code is used when a profile 00258 * or service request cannot be serviced because an operation that has 00259 * been previously triggered is still in progress 00260 */ 00261 PROCEDURE_ALREADY_IN_PROGRESS = 0xFE, 00262 00263 /** The Out of Range error code is used when an attribute value is out 00264 * of range as defined by a profile or service specification. 00265 */ 00266 OUT_OF_RANGE = 0xFF 00267 }; 00268 }; 00269 00270 00271 /** 00272 * The Exchange MTU Request is used by the client to inform the server of the 00273 * client’s maximum receive MTU size and request the server to respond with its 00274 * maximum rx MTU size. 00275 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.2.2 00276 */ 00277 struct AttExchangeMTUResponse : public AttServerMessage { 00278 /** 00279 * Construct an exchange mtu response containing the max rx mtu of the 00280 * server. 00281 * 00282 * @param server_rx_mtu_ The max rx mtu the server can handle. 00283 */ 00284 AttExchangeMTUResponse(uint16_t server_rx_mtu_) : 00285 AttServerMessage(AttributeOpcode::EXCHANGE_MTU_RESPONSE), 00286 server_rx_mtu(server_rx_mtu_) { 00287 } 00288 00289 /** 00290 * The max rx mtu the server can handle. 00291 */ 00292 const uint16_t server_rx_mtu; 00293 }; 00294 00295 00296 /** 00297 * The Find Information Response is sent in reply to a received Find Information 00298 * Request and contains information about this server. 00299 * 00300 * The Find Information Response contains a sequence of handle-uuid pairs in 00301 * ascending order if attribute handles. 00302 * 00303 * This class has to be subclassed by an implementation specific class defining 00304 * the member function size and the subscript operator. 00305 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.3.2 00306 */ 00307 struct AttFindInformationResponse : public AttServerMessage { 00308 00309 /** handle-uuid pair */ 00310 struct information_data_t { 00311 attribute_handle_t handle; 00312 UUID uuid; 00313 }; 00314 00315 /** 00316 * Base constructor, setup the OpCode of the response. 00317 */ 00318 AttFindInformationResponse() : 00319 AttServerMessage(AttributeOpcode::FIND_INFORMATION_RESPONSE) { 00320 } 00321 00322 /** 00323 * virtual destructor to overide if the sub class needs it. 00324 */ 00325 virtual ~AttFindInformationResponse() { } 00326 00327 /** 00328 * Returns the number of information_data_t present in the response. 00329 */ 00330 virtual size_t size() const = 0; 00331 00332 /** 00333 * Access to information_data_t elements present in the response. 00334 * @note Out of range access is undefined. 00335 */ 00336 virtual information_data_t operator[](size_t index) const = 0; 00337 }; 00338 00339 00340 /** 00341 * Find by type value responses are sent in response to find by type value 00342 * request. 00343 * 00344 * The response contains a sequence of Found Attribute Handle, Group End Handle 00345 * pair where: 00346 * - Found Attribute Handle is the handle of an attribute matching the type 00347 * and the value requested. 00348 * - Group End Handle is the end of the attribute group if the attribute found 00349 * is a grouping attribute or the same value as Found Attribute Handle if 00350 * the attribute is not a grouping attribute. 00351 * 00352 * This class should be subclassed by an implementation specific class defining 00353 * the member function size and the subscript operator. 00354 * 00355 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.3.4 00356 */ 00357 struct AttFindByTypeValueResponse : public AttServerMessage { 00358 /** 00359 * Base constructor, setup the OpCode of the response. 00360 */ 00361 AttFindByTypeValueResponse() : 00362 AttServerMessage(AttributeOpcode::FIND_BY_VALUE_TYPE_RESPONSE) { 00363 } 00364 00365 /** 00366 * virtual destructor to overide if the sub class needs it. 00367 */ 00368 virtual ~AttFindByTypeValueResponse() { } 00369 00370 /** 00371 * Returns the number of attribute_handle_range_t present in the response. 00372 */ 00373 virtual std::size_t size() const = 0; 00374 00375 /** 00376 * Access to the attribute range present in the response. 00377 * @note Out of range access is undefined. 00378 */ 00379 virtual attribute_handle_range_t operator[](size_t index) const = 0; 00380 }; 00381 00382 00383 /** 00384 * Response to a Read By Type request. 00385 * 00386 * It contains a list of handle-value pairs where: 00387 * - handle is the handle of the attribute matching the rype requested. 00388 * - value is the value of the attribute found. If the value is longer than 00389 * (mtu - 4) then it can be truncated and read blob request should be used 00390 * to read the remaining octet of the attribute. 00391 * 00392 * This class has to be subclassed by an implementation specific class defining 00393 * the member function size and the subscript operator. 00394 * 00395 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.4.2 00396 */ 00397 struct AttReadByTypeResponse : public AttServerMessage { 00398 /** 00399 * handle-value pair 00400 */ 00401 struct attribute_data_t { 00402 attribute_handle_t handle; 00403 ArrayView<const uint8_t> value; 00404 }; 00405 00406 /** 00407 * Base constructor, setup the OpCode of the response. 00408 */ 00409 AttReadByTypeResponse() : 00410 AttServerMessage(AttributeOpcode::READ_BY_TYPE_RESPONSE) { 00411 } 00412 00413 /** 00414 * virtual destructor to overide if the sub class needs it. 00415 */ 00416 virtual ~AttReadByTypeResponse() { } 00417 00418 /** 00419 * Return the number of attribute_data_t presents in the response. 00420 */ 00421 virtual size_t size() const = 0; 00422 00423 /** 00424 * Return the attribute data at index. 00425 * @note Out of range access is undefined. 00426 */ 00427 virtual attribute_data_t operator[](size_t index) const = 0; 00428 }; 00429 00430 00431 /** 00432 * The read response is sent in reply to a received Read Request and contains 00433 * the value of the attribute that has been read. 00434 * 00435 * The attribute value shall be set to the value of the attribute identified by 00436 * the attribute handle in the request. If the attribute value is longer than 00437 * (ATT_MTU-1) then the first (ATT_MTU-1) octets shall be included in this 00438 * response. 00439 * 00440 * @note The Read Blob Request would be used to read the remaining octets of a 00441 * long attribute value. 00442 * 00443 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.4.4 00444 */ 00445 struct AttReadResponse : public AttServerMessage { 00446 /** 00447 * Construct a Read Response from an array of bytes. 00448 */ 00449 AttReadResponse(ArrayView<const uint8_t> data_) : 00450 AttServerMessage(AttributeOpcode::READ_RESPONSE), _data(data_) { 00451 } 00452 00453 /** 00454 * Return the number of octets presents in the response. 00455 */ 00456 size_t size() const { 00457 return _data.size(); 00458 } 00459 00460 /** 00461 * Return the octet at the specified index. 00462 * @note Out of range access is undefined. 00463 */ 00464 uint8_t operator[](size_t index) const { 00465 return _data[index]; 00466 } 00467 00468 /** 00469 * Return the pointer to the actual data 00470 */ 00471 const uint8_t* data() const { 00472 return _data.data(); 00473 } 00474 00475 private: 00476 const ArrayView<const uint8_t> _data; 00477 }; 00478 00479 00480 /** 00481 * The Read Blob Response is sent in reply to a received Read Blob Request and 00482 * contains part of the value of the attribute that has been read. 00483 * 00484 * If the offset requested is equal to the length of the attribute then the 00485 * response contains no data and the size of the data returned is equal to 0. 00486 * 00487 * If the value of the attribute starting at the offset requested is longer than 00488 * (mtu - 1) octets then the first (mtu - 1) will be present in the response. 00489 * The remaining octets will be acquired by another Read Blob Request with an 00490 * updated index. 00491 * 00492 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.4.6 00493 */ 00494 struct AttReadBlobResponse : public AttServerMessage { 00495 /** 00496 * Construct a read blob response from the value responded. 00497 */ 00498 AttReadBlobResponse(ArrayView<const uint8_t> data_) : 00499 AttServerMessage(AttributeOpcode::READ_BLOB_RESPONSE), _data(data_) { 00500 } 00501 00502 /** 00503 * Return the number of octets presents in the response value. 00504 */ 00505 size_t size() const { 00506 return _data.size(); 00507 } 00508 00509 /** 00510 * Return the octet of the value read at the specified index. 00511 * @note Out of range access is undefined. 00512 */ 00513 uint8_t operator[](size_t index) const { 00514 return _data[index]; 00515 } 00516 00517 /** 00518 * Return the pointer to the actual data 00519 */ 00520 const uint8_t* data() const { 00521 return _data.data(); 00522 } 00523 00524 private: 00525 const ArrayView<const uint8_t> _data; 00526 }; 00527 00528 00529 /** 00530 * Response to a Read Multiple Request. It contains the values of the attributes 00531 * that have been read. 00532 * 00533 * If the set of values that has been read is longer than (mtu - 1) then only 00534 * the first (mtu - 1) octets are included in the response. 00535 * 00536 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.4.8 00537 */ 00538 struct AttReadMultipleResponse : public AttServerMessage { 00539 /** 00540 * Construct a Resd Multiple Response from the set of value received. 00541 */ 00542 AttReadMultipleResponse(ArrayView<const uint8_t> data_) : 00543 AttServerMessage(AttributeOpcode::READ_MULTIPLE_RESPONSE), _data(data_) { 00544 } 00545 00546 /** 00547 * Return the number of octets presents in the response set of value. 00548 */ 00549 size_t size() const { 00550 return _data.size(); 00551 } 00552 00553 /** 00554 * Return the octet of the set of value read at the specified index. 00555 * @note Out of range access is undefined. 00556 */ 00557 uint8_t operator[](size_t index) const { 00558 return _data[index]; 00559 } 00560 00561 private: 00562 const ArrayView<const uint8_t> _data; 00563 }; 00564 00565 00566 /** 00567 * The Read By Group Type Response is sent in reply to a received Read By 00568 * Group Type Request and contains the handles and values of the attributes that 00569 * have been read. 00570 * 00571 * The response is a list of group range-value pair where: 00572 * - group range: The range of the group found where begin is the grouping 00573 * attribute handle and end is the handle of the end of the group. 00574 * - value: The value of the grouping attribute. 00575 * 00576 * This class has to be subclassed by an implementation specific class defining 00577 * the member function size and the subscript operator. 00578 * 00579 * @note The value responded can be trucated if it doesn't fit in the response, 00580 * in that case a Read Blob Request could be used to read the remaining octets. 00581 * 00582 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.4.10 00583 */ 00584 struct AttReadByGroupTypeResponse : public AttServerMessage { 00585 /** 00586 * Data read from the grouping attribute. 00587 * It includes the range of the group and the value of the attribute. 00588 */ 00589 struct attribute_data_t { 00590 attribute_handle_range_t group_range; 00591 ArrayView<const uint8_t> value; 00592 }; 00593 00594 /** 00595 * Base constructor, setup the OpCode of the response. 00596 */ 00597 AttReadByGroupTypeResponse() : 00598 AttServerMessage(AttributeOpcode::READ_BY_GROUP_TYPE_RESPONSE) { 00599 } 00600 00601 /** 00602 * virtual destructor to overide if the sub class needs it. 00603 */ 00604 virtual ~AttReadByGroupTypeResponse() { } 00605 00606 /** 00607 * Return the number of attribute_data_t present in the response. 00608 */ 00609 virtual size_t size() const = 0; 00610 00611 /** 00612 * Return the attribute data read at the index specified. 00613 * @note Out of range access is undefined. 00614 */ 00615 virtual attribute_data_t operator[](size_t index) const = 0; 00616 }; 00617 00618 00619 /** 00620 * The Write Response is sent in reply to a valid Write Request and 00621 * acknowledges that the attribute has been successfully written. 00622 * It is just a placeholder which indicates the client that the write request 00623 * was successful. 00624 * 00625 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.5.2 00626 */ 00627 struct AttWriteResponse : public AttServerMessage { 00628 /** 00629 * Construct a write response. 00630 */ 00631 AttWriteResponse() : AttServerMessage(AttributeOpcode::WRITE_RESPONSE) { } 00632 }; 00633 00634 00635 /** 00636 * Response to a Prepare Write Request. It acknowledges the client that the 00637 * value has been successfully received and placed in the write queue. 00638 * 00639 * The response contains the same values as the one present in the request. 00640 * 00641 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.6.2 00642 */ 00643 struct AttPrepareWriteResponse : public AttServerMessage { 00644 /** 00645 * Construct a prepare write response. 00646 * @param handle_ The handle of the attribute to be written. 00647 * @param offset_: The offset of the first octet to be writen. 00648 * @param value_: The value of the attribute to be written at the offset 00649 * indicated. 00650 */ 00651 AttPrepareWriteResponse( 00652 attribute_handle_t handle_, 00653 uint16_t offset_, 00654 ArrayView<const uint8_t> value_ 00655 ) : AttServerMessage(AttributeOpcode::PREPARE_WRITE_RESPONSE), 00656 attribute_handle(handle_), 00657 offset(offset_), 00658 partial_value(value_) { 00659 } 00660 00661 /** 00662 * The handle of the attribute to be written. 00663 */ 00664 const attribute_handle_t attribute_handle; 00665 00666 /** 00667 * The offset of the first octet to be writen. 00668 */ 00669 const uint16_t offset; 00670 00671 /** 00672 * The value of the attribute to be written at the offset indicated. 00673 */ 00674 const ArrayView<const uint8_t> partial_value; 00675 }; 00676 00677 00678 /** 00679 * The Execute Write Response is sent in response to a received Execute Write 00680 * Request. 00681 * 00682 * It is just a placeholder which indicates the client that the execution of the 00683 * write request has been successfull. 00684 * 00685 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.6.4 00686 */ 00687 struct AttExecuteWriteResponse : public AttServerMessage { 00688 /** 00689 * Construct an execute write response object. 00690 */ 00691 AttExecuteWriteResponse() : 00692 AttServerMessage(AttributeOpcode::EXECUTE_WRITE_RESPONSE) { 00693 } 00694 }; 00695 00696 00697 /** 00698 * Notification of an attribute's value sent by the server. 00699 * 00700 * It contains the handle of the attribute and its value. 00701 * 00702 * If the attribute value is longer than (mtu - 3) then the value is truncated 00703 * to (mtu - 3) octets to fit in the response and the client will have to use 00704 * a read blob request to read the remaining octets of the attribute. 00705 * 00706 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.7.1 00707 */ 00708 struct AttHandleValueNotification : public AttServerMessage { 00709 /** 00710 * Construct an Handle Value Notification from the attribute handle and its 00711 * value notified. 00712 */ 00713 AttHandleValueNotification( 00714 attribute_handle_t attribute_handle, 00715 ArrayView<const uint8_t> attribute_value 00716 ) : AttServerMessage(AttributeOpcode::HANDLE_VALUE_NOTIFICATION), 00717 attribute_handle(attribute_handle), 00718 attribute_value(attribute_value) { 00719 } 00720 00721 /** 00722 * Handle of the attribute 00723 */ 00724 const attribute_handle_t attribute_handle; 00725 00726 /** 00727 * The current value of the attribute. 00728 */ 00729 const ArrayView<const uint8_t> attribute_value; 00730 }; 00731 00732 00733 /** 00734 * Indication of an attribute's value sent by the server. 00735 * 00736 * It contains the handle of the attribute and its value. The client should 00737 * respond with and handle value confirmation. 00738 * 00739 * If the attribute value is longer than (mtu - 3) then the value is truncated 00740 * to (mtu - 3) octets to fit in the response and the client will have to use 00741 * a read blob request to read the remaining octets of the attribute. 00742 * 00743 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.7.2 00744 */ 00745 struct AttHandleValueIndication : public AttServerMessage { 00746 /** 00747 * Construct an Handle Value Indication from the attribute handle and its 00748 * value indicated. 00749 */ 00750 AttHandleValueIndication( 00751 attribute_handle_t handle, ArrayView<const uint8_t> value 00752 ) : AttServerMessage(AttributeOpcode::HANDLE_VALUE_INDICATION), 00753 attribute_handle(handle), attribute_value(value) { 00754 } 00755 00756 /** 00757 * Handle of the attribute 00758 */ 00759 const attribute_handle_t attribute_handle; 00760 00761 /** 00762 * The current value of the attribute. 00763 */ 00764 const ArrayView<const uint8_t> attribute_value; 00765 }; 00766 00767 00768 } // namespace pal 00769 } // namespace ble 00770 00771 #endif /* BLE_PAL_ATT_SERVER_MESSAGE_H_ */
Generated on Sun Jul 17 2022 08:25:20 by
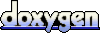