
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ArrayView.h
Go to the documentation of this file.
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017-2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef BLE_ARRAY_VIEW_H_ 00018 #define BLE_ARRAY_VIEW_H_ 00019 00020 #include <stddef.h> 00021 #include <stdint.h> 00022 00023 /** 00024 * @addtogroup ble 00025 * @{ 00026 * @addtogroup common 00027 * @{ 00028 */ 00029 00030 /** 00031 * @file 00032 */ 00033 00034 namespace ble { 00035 00036 /** 00037 * Immutable view to an array. 00038 * 00039 * Array views encapsulate the pointer to an array and its size into a single 00040 * object; however, it does not manage the lifetime of the array viewed. 00041 * You can use instances of ArrayView to replace the traditional pair of pointer 00042 * and size arguments in function calls. 00043 * 00044 * You can use the size member function to query the number of elements present 00045 * in the array, and overloads of the subscript operator allow code using 00046 * this object to access to the content of the array viewed. 00047 * 00048 * @note You can create ArrayView instances with the help of the function 00049 * template make_ArrayView() and make_const_ArrayView(). 00050 * 00051 * @tparam T type of objects held by the array. 00052 */ 00053 template<typename T> 00054 struct ArrayView { 00055 00056 /** 00057 * Construct a view to an empty array. 00058 * 00059 * @post a call to size() will return 0, and data() will return NULL. 00060 */ 00061 ArrayView() : _array(0), _size(0) { } 00062 00063 /** 00064 * Construct an array view from a pointer to a buffer and its size. 00065 * 00066 * @param array_ptr Pointer to the array data 00067 * @param array_size Number of elements of T present in the array. 00068 * 00069 * @post a call to size() will return array_size and data() will return 00070 * array_tpr. 00071 */ 00072 ArrayView(T* array_ptr, size_t array_size) : 00073 _array(array_ptr), _size(array_size) { } 00074 00075 /** 00076 * Construct an array view from the reference to an array. 00077 * 00078 * @param elements Reference to the array viewed. 00079 * 00080 * @tparam Size Number of elements of T presents in the array. 00081 * 00082 * @post a call to size() will return Size, and data() will return 00083 * a pointer to elements. 00084 */ 00085 template<size_t Size> 00086 ArrayView(T (&elements)[Size]): 00087 _array(elements), _size(Size) { } 00088 00089 /** 00090 * Return the size of the array viewed. 00091 * 00092 * @return The number of elements present in the array viewed. 00093 */ 00094 size_t size() const 00095 { 00096 return _size; 00097 } 00098 00099 /** 00100 * Access to a mutable element of the array. 00101 * 00102 * @param index Element index to access. 00103 * 00104 * @return A reference to the element at the index specified in input. 00105 * 00106 * @pre index shall be less than size(). 00107 */ 00108 T& operator[](size_t index) 00109 { 00110 return _array[index]; 00111 } 00112 00113 /** 00114 * Access to an immutable element of the array. 00115 * 00116 * @param index Element index to access. 00117 * 00118 * @return A const reference to the element at the index specified in input. 00119 * 00120 * @pre index shall be less than size(). 00121 */ 00122 const T& operator[](size_t index) const 00123 { 00124 return _array[index]; 00125 } 00126 00127 /** 00128 * Get the raw pointer to the array. 00129 * 00130 * @return The raw pointer to the array. 00131 */ 00132 T* data() 00133 { 00134 return _array; 00135 } 00136 00137 /** 00138 * Get the raw const pointer to the array. 00139 * 00140 * @return The raw pointer to the array. 00141 */ 00142 const T* data() const 00143 { 00144 return _array; 00145 } 00146 00147 /** 00148 * Equality operator. 00149 * 00150 * @param lhs Left hand side of the binary operation. 00151 * @param rhs Right hand side of the binary operation. 00152 * 00153 * @return True if arrays in input have the same size and the same content 00154 * and false otherwise. 00155 */ 00156 friend bool operator==(const ArrayView& lhs, const ArrayView& rhs) 00157 { 00158 if (lhs.size() != rhs.size()) { 00159 return false; 00160 } 00161 00162 if (lhs.data() == rhs.data()) { 00163 return true; 00164 } 00165 00166 return memcmp(lhs.data(), rhs.data(), lhs.size()) == 0; 00167 } 00168 00169 /** 00170 * Not equal operator 00171 * 00172 * @param lhs Left hand side of the binary operation. 00173 * @param rhs Right hand side of the binary operation. 00174 * 00175 * @return True if arrays in input do not have the same size or the same 00176 * content and false otherwise. 00177 */ 00178 friend bool operator!=(const ArrayView& lhs, const ArrayView& rhs) 00179 { 00180 return !(lhs == rhs); 00181 } 00182 00183 private: 00184 T* const _array; 00185 const size_t _size; 00186 }; 00187 00188 00189 /** 00190 * Generate an array view from a reference to a C/C++ array. 00191 * 00192 * @tparam T Type of elements held in elements. 00193 * @tparam Size Number of items held in elements. 00194 * 00195 * @param elements The reference to the array viewed. 00196 * 00197 * @return The ArrayView to elements. 00198 * 00199 * @note This helper avoids the typing of template parameter when ArrayView is 00200 * created 'inline'. 00201 */ 00202 template<typename T, size_t Size> 00203 ArrayView<T> make_ArrayView(T (&elements)[Size]) 00204 { 00205 return ArrayView<T>(elements); 00206 } 00207 00208 /** 00209 * Generate an array view from a C/C++ pointer and the size of the array. 00210 * 00211 * @tparam T Type of elements held in array_ptr. 00212 * 00213 * @param array_ptr The pointer to the array to viewed. 00214 * @param array_size The number of T elements in the array. 00215 * 00216 * @return The ArrayView to array_ptr with a size of array_size. 00217 * 00218 * @note This helper avoids the typing of template parameter when ArrayView is 00219 * created 'inline'. 00220 */ 00221 template<typename T> 00222 ArrayView<T> make_ArrayView(T* array_ptr, size_t array_size) 00223 { 00224 return ArrayView<T>(array_ptr, array_size); 00225 } 00226 00227 /** 00228 * Generate a const array view from a reference to a C/C++ array. 00229 * 00230 * @tparam T Type of elements held in elements. 00231 * @tparam Size Number of items held in elements. 00232 * 00233 * @param elements The array viewed. 00234 * @return The ArrayView to elements. 00235 * 00236 * @note This helper avoids the typing of template parameter when ArrayView is 00237 * created 'inline'. 00238 */ 00239 template<typename T, size_t Size> 00240 ArrayView<const T> make_const_ArrayView(T (&elements)[Size]) 00241 { 00242 return ArrayView<const T>(elements); 00243 } 00244 00245 /** 00246 * Generate a const array view from a C/C++ pointer and the size of the array. 00247 * 00248 * @tparam T Type of elements held in array_ptr. 00249 * 00250 * @param array_ptr The pointer to the array to viewed. 00251 * @param array_size The number of T elements in the array. 00252 * 00253 * @return The ArrayView to array_ptr with a size of array_size. 00254 * 00255 * @note This helper avoids the typing of template parameter when ArrayView is 00256 * created 'inline'. 00257 */ 00258 template<typename T> 00259 ArrayView<const T> make_const_ArrayView(T* array_ptr, size_t array_size) 00260 { 00261 return ArrayView<const T>(array_ptr, array_size); 00262 } 00263 00264 } // namespace ble 00265 00266 /** 00267 * @} 00268 * @} 00269 */ 00270 00271 00272 #endif /* BLE_ARRAY_VIEW_H_ */
Generated on Sun Jul 17 2022 08:25:20 by
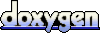