
This is the DW1000 driver and our self developed distance measurement application based on it. We do this as a semester thesis at ETH Zürich under the Automatic Control Laboratory in the Department of electrical engineering.
PC.cpp
00001 #include "PC.h" 00002 #include "mbed.h" 00003 00004 PC::PC(PinName tx, PinName rx, int baudrate) : Serial(tx, rx) 00005 { 00006 baud(baudrate); 00007 cls(); 00008 00009 command[0] = '\0'; 00010 command_char_count = 0; 00011 } 00012 00013 00014 void PC::cls() 00015 { 00016 printf("\x1B[2J"); 00017 } 00018 00019 00020 void PC::locate(int Spalte, int Zeile) 00021 { 00022 printf("\x1B[%d;%dH", Zeile + 1, Spalte + 1); 00023 } 00024 00025 void PC::readcommand(void (*executer)(char*)) 00026 { 00027 char input = getc(); // get the character from serial bus 00028 if(input == '\r') { // if return was pressed, the command must be executed 00029 command[command_char_count] = '\0'; 00030 executer(&command[0]); 00031 00032 command_char_count = 0; // reset command 00033 command[command_char_count] = '\0'; 00034 } else if (command_char_count < COMMAND_MAX_LENGHT) { 00035 command[command_char_count] = input; 00036 command_char_count++; 00037 } 00038 }
Generated on Tue Jul 12 2022 15:21:24 by
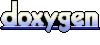