
This is the DW1000 driver and our self developed distance measurement application based on it. We do this as a semester thesis at ETH Zürich under the Automatic Control Laboratory in the Department of electrical engineering.
MMRanging.h
00001 // by Matthias Grob & Manuel Stalder - ETH Zürich - 2015 00002 00003 #ifndef MMRANGING_H 00004 #define MMRANGING_H 00005 00006 #include "mbed.h" 00007 #include "DW1000.h" 00008 00009 #define MMRANGING_TIMEUNIT_US 1/(128*499.2) // conversion between LSB of TX and RX timestamps and microseconds 00010 #define MMRANGING_TIMEUNIT_NS 1000/(128*499.2) // conversion between LSB of TX and RX timestamps and nanoseconds 00011 00012 #define MMRANGING_2POWER40 1099511627776 // decimal value of 2^40 to correct timeroverflow between timestamps 00013 00014 //#define EVENTS // to see debug output of occurring interrupt callbacks 00015 00016 class MMRanging { 00017 public: 00018 MMRanging(DW1000& DW); 00019 void requestRanging(uint8_t destination); 00020 void requestRangingAll(); 00021 //private: 00022 DW1000& dw; 00023 Timer LocalTimer; 00024 00025 void callbackRX(); 00026 void callbackTX(); 00027 void sendRangingframe(uint8_t destination, uint8_t sequence_number, uint8_t type, uint64_t time_difference_receiver); 00028 uint64_t timeDifference40Bit(uint64_t early, uint64_t late); // Method to calculate the difference between two 40-Bit timestamps correcting timer overflow reset occurring between the timestamps 00029 00030 uint8_t address; // Identifies the nodes as source and destination in rangingframes 00031 //struct __attribute__((packed, aligned(1))) rangingframe { // TODO: avoid structure padding, 32-Bit enough for time_difference_receiver => 8 Byte per frame instead of 16 00032 struct rangingframe { 00033 uint8_t source; 00034 uint8_t destination; 00035 uint8_t sequence_number; 00036 uint8_t type; 00037 uint64_t time_difference_receiver; 00038 }; 00039 00040 rangingframe TX; // buffer in class for sending a frame (not made locally because then we can recall in the interrupt what was sent) 00041 uint64_t rangingtimingsSender[10][2]; 00042 uint64_t rangingtimingsReceiver[10][2]; 00043 bool acknowledgement[10]; // flag to indicate if ranging has succeeded 00044 uint64_t tofs[10]; // Array containing time of flights for each node (index is address of node) 00045 float roundtriptimes[10]; // Array containing the round trip times to the anchors or the timeout which occured 00046 float distances[10]; // Array containing the finally calculated Distances to the anchors 00047 00048 // draft for first test 00049 bool receiver; 00050 int event_i; 00051 char event[10][20]; 00052 uint8_t counter; 00053 }; 00054 00055 #endif
Generated on Tue Jul 12 2022 15:21:24 by
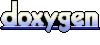