Code for our FYDP -only one IMU works right now -RTOS is working
Embed:
(wiki syntax)
Show/hide line numbers
SWSPI.cpp
00001 /* SWSPI, Software SPI library 00002 * Copyright (c) 2012-2014, David R. Van Wagner, http://techwithdave.blogspot.com 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #include <mbed.h> 00024 #include "SWSPI.h" 00025 00026 SWSPI::SWSPI(PinName mosi_pin, PinName miso_pin, PinName sclk_pin) 00027 { 00028 mosi = new DigitalOut(mosi_pin); 00029 miso = new DigitalIn(miso_pin); 00030 sclk = new DigitalOut(sclk_pin); 00031 format(8); 00032 frequency(); 00033 } 00034 00035 SWSPI::~SWSPI() 00036 { 00037 delete mosi; 00038 delete miso; 00039 delete sclk; 00040 } 00041 00042 void SWSPI::format(int bits, int mode) 00043 { 00044 this->bits = bits; 00045 this->mode = mode; 00046 polarity = (mode >> 1) & 1; 00047 phase = mode & 1; 00048 sclk->write(polarity); 00049 } 00050 00051 void SWSPI::frequency(int hz) 00052 { 00053 this->freq = hz; 00054 } 00055 00056 int SWSPI::write(int value) 00057 { 00058 int read = 0; 00059 for (int bit = bits-1; bit >= 0; --bit) 00060 { 00061 mosi->write(((value >> bit) & 0x01) != 0); 00062 00063 if (phase == 0) 00064 { 00065 if (miso->read()) 00066 read |= (1 << bit); 00067 } 00068 00069 sclk->write(!polarity); 00070 00071 wait(1.0/freq/2); 00072 00073 if (phase == 1) 00074 { 00075 if (miso->read()) 00076 read |= (1 << bit); 00077 } 00078 00079 sclk->write(polarity); 00080 00081 wait(1.0/freq/2); 00082 } 00083 00084 return read; 00085 } 00086
Generated on Wed Jul 13 2022 10:32:23 by
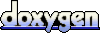