
Test program for testing ADC channels, and 2004 LED display functions of W7500ECO-based LINAC MO monitor board
Dependencies: TextLCD WIZnetInterface mbed
Fork of TCP_LED_Control-WIZwiki-W7500 by
main.cpp
00001 // Test of ADC, and LED/LCD control of LINAC MO Monitor 00002 // Hengjie Ma 00003 // BNL, NSLS-II, RF 00004 // Nov. 2, 2017 00005 // 00006 #include "mbed.h" 00007 #include "TextLCD.h" 00008 #include <stdio.h> 00009 00010 // Analog signal input channel gain scalling 00011 #define AIN_cal_CL1 1.0; // RFIN_500M 00012 #define AIN_cal_CL2 1.0; // SPB_500M 00013 #define AIN_cal_CL3 1.0; // GUN_500M 00014 #define AIN_cal_CL4 1.0; // PB_3G 00015 #define AIN_cal_CL5 1.0; // K1_3G 00016 #define AIN_cal_CL6 1.0; // K2_3G 00017 #define AIN_cal_OP1 1.0; // K3_3G 00018 #define AIN_cal_OP2 1.0; // 5V_OK 00019 #define AIN_cal_OP3 1.0; // 15V_OK 00020 #define AIN_cal_OP4 1.0; // AIN3_SPARE 00021 #define AIN_cal_OP5 1.0; // AIN6_SPARE 00022 #define AIN_cal_OP6 1.0; // AIN7_SPARE 00023 00024 const char * msg[] = { 00025 "RFIN500M ", 00026 "SPB500M ", 00027 "GUN500M ", 00028 "PB_3G ", 00029 "K1_3G ", 00030 "K2_3G ", 00031 "K3_3G ", 00032 "5V_OK ", 00033 "15V_OK ", 00034 "AIN3_xxx ", 00035 "AIN6_xxx ", 00036 "AIN7_xxx " 00037 }; 00038 00039 char trailer[16] = "me too !!!"; // test string 00040 00041 // I/O pin assignment 00042 DigitalOut myled(LED1); // on-board blue LED, an idiot light for TCP sanity check 00043 // Primary group of analog inputs 00044 AnalogIn AIN0(P30); // AIN0, RFIN_500M/K3_3G 00045 AnalogIn AIN1(P29); // AIN1, SPB_500M/5V_OK 00046 AnalogIn AIN2(P28); // AIN2, GUN_500M/15V_OK 00047 AnalogIn AIN3(P27); // AIN3, PB_3G/AIN3_SPARE 00048 AnalogIn AIN6(P26); // AIN6, K1_3G/AIN6_SPARE 00049 AnalogIn AIN7(P25); // AIN7, K2_3G/AIN7_SPARE 00050 DigitalOut MUX_CTL(P11); // PA11, 00051 00052 // TextLCD lcd(P9, P10, P5,P6, P7, P8, TextLCD::LCD20x4D, NC, NC, TextLCD::US2066_3V3); // RS, E, D4-D7, LCDType=LCD16x2, BL=NC, E2=NC, LCDTCtrl=US2066 00053 TextLCD lcd(P9, P10, P5,P8, P7, P6, TextLCD::LCD20x4D, NC, NC, TextLCD::US2066_3V3); // RS, E, D4-D7, LCDType=LCD16x2, BL=NC, E2=NC, LCDTCtrl=US2066 00054 float meas[12]; 00055 int idx, row, col; 00056 00057 void adc() 00058 { 00059 // Update data 00060 MUX_CTL = 0; // group 1 00061 meas[0] = AIN0.read() * AIN_cal_CL1; 00062 meas[1] = AIN1.read() * AIN_cal_CL2; 00063 meas[2] = AIN2.read() * AIN_cal_CL3; 00064 meas[3] = AIN3.read() * AIN_cal_CL4; 00065 meas[4] = AIN6.read() * AIN_cal_CL5; 00066 meas[5] = AIN7.read() * AIN_cal_CL6; 00067 MUX_CTL = 1; // group 2 00068 meas[6] = AIN0.read() * AIN_cal_OP1; 00069 meas[7] = AIN1.read() * AIN_cal_OP2; 00070 meas[8] = AIN2.read() * AIN_cal_OP3; 00071 meas[9] = AIN3.read() * AIN_cal_OP4; 00072 meas[10] = AIN6.read() * AIN_cal_OP5; 00073 meas[11] = AIN7.read() * AIN_cal_OP6; 00074 } 00075 00076 void display() 00077 { 00078 // update 20x4 LED/LCD display, rolling 00079 lcd.cls(); 00080 for ( idx = 0; idx < 12; idx++) 00081 { 00082 // lcd.locate(col,row); 00083 printf("%s %.2f V \r\n", msg[idx], meas[idx]); // serial console 00084 lcd.printf("%s %.2f V \r\n",msg[idx], meas[idx]); // LCD/LED 00085 wait(0.1); // per line 00086 if (row < 3) 00087 { 00088 row = row + 1; 00089 lcd.locate(col,row); 00090 } 00091 else 00092 { 00093 row = 0; 00094 wait(1.0); // pause after each frame of 4 lines 00095 lcd.cls(); 00096 } 00097 } 00098 // wait(1.0); // every 4-screen, 12-line total, refresh 00099 } 00100 00101 int main (void) 00102 { 00103 row = 0; 00104 col = 0; 00105 printf("Test of ADC and LCD .....\r\n"); 00106 while(true) 00107 { 00108 // get new data 00109 adc(); 00110 // display on serial terminal and LCD 00111 display(); 00112 } 00113 } 00114 00115
Generated on Fri Jul 15 2022 01:10:20 by
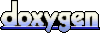