
This is for ICRS\' second generation Quadcopter
Embed:
(wiki syntax)
Show/hide line numbers
RF12B.h
00001 #ifndef _RF12B_H 00002 #define _RF12B_H 00003 00004 #include "mbed.h" 00005 #include <queue> 00006 00007 enum rfmode_t{RX, TX}; 00008 00009 class RF12B { 00010 public: 00011 /* Constructor */ 00012 RF12B(PinName SDI, 00013 PinName SDO, 00014 PinName SCK, 00015 PinName NCS, 00016 PinName NIRQ); 00017 00018 00019 00020 /* Reads a packet of data. Returns false if read failed. Use available() to check how much space to allocate for buffer */ 00021 bool read(unsigned char* data, unsigned int size); 00022 00023 /* Reads a byte of data from the receive buffer 00024 Returns 0xFF if there is no data */ 00025 unsigned char read(); 00026 00027 /* Transmits a packet of data */ 00028 void write(unsigned char* data, unsigned char length); 00029 void write(unsigned char data); /* 1-byte packet */ 00030 void write(queue<char> &data, int length = -1); /* sends a whole queue */ 00031 00032 /* Returns the packet length if data is available in the receive buffer, 0 otherwise*/ 00033 unsigned int available(); 00034 00035 protected: 00036 /* Receive FIFO buffer */ 00037 queue<unsigned char> fifo; 00038 00039 /* SPI module */ 00040 SPI spi; 00041 00042 /* Other digital pins */ 00043 DigitalOut NCS; 00044 InterruptIn NIRQ; 00045 DigitalIn NIRQ_in; 00046 DigitalOut rfled; 00047 00048 rfmode_t mode; 00049 00050 /* Initialises the RF12B module */ 00051 void init(); 00052 00053 /* Write a command to the RF Module */ 00054 unsigned int writeCmd(unsigned int cmd); 00055 00056 /* Sends a byte of data across RF */ 00057 void send(unsigned char data); 00058 00059 /* Switch module between receive and transmit modes */ 00060 void changeMode(rfmode_t mode); 00061 00062 /* Interrupt routine for data reception */ 00063 void rxISR(); 00064 00065 /* Tell the RF Module this packet is received and wait for the next */ 00066 void resetRX(); 00067 00068 /* Return the RF Module Status word */ 00069 unsigned int status(); 00070 00071 /* Calculate CRC8 */ 00072 unsigned char crc8(unsigned char crc, unsigned char data); 00073 }; 00074 00075 #endif /* _RF12B_H */
Generated on Sat Jul 16 2022 19:40:54 by
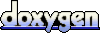