
This is some awesome robot code
Dependencies: mbed-rtos mbed QEI
Fork of ICRSEurobot13 by
VectorBinaryFunctions.h
00001 /* 00002 * Tiny Vector Matrix Library 00003 * Dense Vector Matrix Libary of Tiny size using Expression Templates 00004 * 00005 * Copyright (C) 2001 - 2007 Olaf Petzold <opetzold@users.sourceforge.net> 00006 * 00007 * This library is free software; you can redistribute it and/or 00008 * modify it under the terms of the GNU Lesser General Public 00009 * License as published by the Free Software Foundation; either 00010 * version 2.1 of the License, or (at your option) any later version. 00011 * 00012 * This library is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00015 * Lesser General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU Lesser General Public 00018 * License along with this library; if not, write to the Free Software 00019 * Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA 00020 * 00021 * $Id: VectorBinaryFunctions.h,v 1.12 2007-06-23 15:59:00 opetzold Exp $ 00022 */ 00023 00024 #ifndef TVMET_XPR_VECTOR_BINARY_FUNCTIONS_H 00025 #define TVMET_XPR_VECTOR_BINARY_FUNCTIONS_H 00026 00027 namespace tvmet { 00028 00029 00030 /********************************************************* 00031 * PART I: DECLARATION 00032 *********************************************************/ 00033 00034 00035 /* 00036 * binary_function(XprVector<E1, Sz>, XprVector<E2, Sz>) 00037 */ 00038 #define TVMET_DECLARE_MACRO(NAME) \ 00039 template<class E1, class E2, std::size_t Sz> \ 00040 inline \ 00041 XprVector< \ 00042 XprBinOp< \ 00043 Fcnl_##NAME<typename E1::value_type, typename E2::value_type>, \ 00044 XprVector<E1, Sz>, \ 00045 XprVector<E2, Sz> \ 00046 >, \ 00047 Sz \ 00048 > \ 00049 NAME(const XprVector<E1, Sz>& lhs, \ 00050 const XprVector<E2, Sz>& rhs) TVMET_CXX_ALWAYS_INLINE; 00051 00052 TVMET_DECLARE_MACRO(atan2) 00053 TVMET_DECLARE_MACRO(drem) 00054 TVMET_DECLARE_MACRO(fmod) 00055 TVMET_DECLARE_MACRO(hypot) 00056 TVMET_DECLARE_MACRO(jn) 00057 TVMET_DECLARE_MACRO(yn) 00058 TVMET_DECLARE_MACRO(pow) 00059 #if defined(TVMET_HAVE_COMPLEX) 00060 TVMET_DECLARE_MACRO(polar) 00061 #endif 00062 00063 #undef TVMET_DECLARE_MACRO 00064 00065 00066 /* 00067 * binary_function(XprVector<E, Sz>, POD) 00068 */ 00069 #define TVMET_DECLARE_MACRO(NAME, TP) \ 00070 template<class E, std::size_t Sz> \ 00071 inline \ 00072 XprVector< \ 00073 XprBinOp< \ 00074 Fcnl_##NAME<typename E::value_type, TP >, \ 00075 XprVector<E, Sz>, \ 00076 XprLiteral< TP > \ 00077 >, \ 00078 Sz \ 00079 > \ 00080 NAME(const XprVector<E, Sz>& lhs, \ 00081 TP rhs) TVMET_CXX_ALWAYS_INLINE; 00082 00083 TVMET_DECLARE_MACRO(atan2, int) 00084 TVMET_DECLARE_MACRO(drem, int) 00085 TVMET_DECLARE_MACRO(fmod, int) 00086 TVMET_DECLARE_MACRO(hypot, int) 00087 TVMET_DECLARE_MACRO(jn, int) 00088 TVMET_DECLARE_MACRO(yn, int) 00089 TVMET_DECLARE_MACRO(pow, int) 00090 00091 #if defined(TVMET_HAVE_LONG_LONG) 00092 TVMET_DECLARE_MACRO(atan2, long long int) 00093 TVMET_DECLARE_MACRO(drem, long long int) 00094 TVMET_DECLARE_MACRO(fmod, long long int) 00095 TVMET_DECLARE_MACRO(hypot, long long int) 00096 TVMET_DECLARE_MACRO(jn, long long int) 00097 TVMET_DECLARE_MACRO(yn, long long int) 00098 TVMET_DECLARE_MACRO(pow, long long int) 00099 #endif // defined(TVMET_HAVE_LONG_LONG) 00100 00101 TVMET_DECLARE_MACRO(atan2, float) 00102 TVMET_DECLARE_MACRO(drem, float) 00103 TVMET_DECLARE_MACRO(fmod, float) 00104 TVMET_DECLARE_MACRO(hypot, float) 00105 TVMET_DECLARE_MACRO(jn, float) 00106 TVMET_DECLARE_MACRO(yn, float) 00107 TVMET_DECLARE_MACRO(pow, float) 00108 00109 TVMET_DECLARE_MACRO(atan2, double) 00110 TVMET_DECLARE_MACRO(drem, double) 00111 TVMET_DECLARE_MACRO(fmod, double) 00112 TVMET_DECLARE_MACRO(hypot, double) 00113 TVMET_DECLARE_MACRO(jn, double) 00114 TVMET_DECLARE_MACRO(yn, double) 00115 TVMET_DECLARE_MACRO(pow, double) 00116 00117 #if defined(TVMET_HAVE_LONG_DOUBLE) 00118 TVMET_DECLARE_MACRO(atan2, long double) 00119 TVMET_DECLARE_MACRO(drem, long double) 00120 TVMET_DECLARE_MACRO(fmod, long double) 00121 TVMET_DECLARE_MACRO(hypot, long double) 00122 TVMET_DECLARE_MACRO(jn, long double) 00123 TVMET_DECLARE_MACRO(yn, long double) 00124 TVMET_DECLARE_MACRO(pow, long double) 00125 #endif // defined(TVMET_HAVE_LONG_DOUBLE) 00126 00127 #undef TVMET_DECLARE_MACRO 00128 00129 00130 #if defined(TVMET_HAVE_COMPLEX) 00131 /* 00132 * binary_function(XprVector<E, Sz>, std::complex<>) 00133 */ 00134 #define TVMET_DECLARE_MACRO(NAME) \ 00135 template<class E, std::size_t Sz, class T> \ 00136 inline \ 00137 XprVector< \ 00138 XprBinOp< \ 00139 Fcnl_##NAME<typename E::value_type, std::complex<T> >, \ 00140 XprVector<E, Sz>, \ 00141 XprLiteral< std::complex<T> > \ 00142 >, \ 00143 Sz \ 00144 > \ 00145 NAME(const XprVector<E, Sz>& lhs, \ 00146 const std::complex<T>& rhs) TVMET_CXX_ALWAYS_INLINE; 00147 00148 TVMET_DECLARE_MACRO(atan2) 00149 TVMET_DECLARE_MACRO(drem) 00150 TVMET_DECLARE_MACRO(fmod) 00151 TVMET_DECLARE_MACRO(hypot) 00152 TVMET_DECLARE_MACRO(jn) 00153 TVMET_DECLARE_MACRO(yn) 00154 TVMET_DECLARE_MACRO(pow) 00155 00156 #undef TVMET_DECLARE_MACRO 00157 00158 #endif // defined(TVMET_HAVE_COMPLEX) 00159 00160 00161 /********************************************************* 00162 * PART II: IMPLEMENTATION 00163 *********************************************************/ 00164 00165 00166 /* 00167 * binary_function(XprVector<E1, Sz>, XprVector<E2, Sz>) 00168 */ 00169 #define TVMET_IMPLEMENT_MACRO(NAME) \ 00170 template<class E1, class E2, std::size_t Sz> \ 00171 inline \ 00172 XprVector< \ 00173 XprBinOp< \ 00174 Fcnl_##NAME<typename E1::value_type, typename E2::value_type>, \ 00175 XprVector<E1, Sz>, \ 00176 XprVector<E2, Sz> \ 00177 >, \ 00178 Sz \ 00179 > \ 00180 NAME(const XprVector<E1, Sz>& lhs, const XprVector<E2, Sz>& rhs) { \ 00181 typedef XprBinOp< \ 00182 Fcnl_##NAME<typename E1::value_type, typename E2::value_type>, \ 00183 XprVector<E1, Sz>, \ 00184 XprVector<E2, Sz> \ 00185 > expr_type; \ 00186 return XprVector<expr_type, Sz>( \ 00187 expr_type(lhs, rhs)); \ 00188 } 00189 00190 TVMET_IMPLEMENT_MACRO(atan2) 00191 TVMET_IMPLEMENT_MACRO(drem) 00192 TVMET_IMPLEMENT_MACRO(fmod) 00193 TVMET_IMPLEMENT_MACRO(hypot) 00194 TVMET_IMPLEMENT_MACRO(jn) 00195 TVMET_IMPLEMENT_MACRO(yn) 00196 TVMET_IMPLEMENT_MACRO(pow) 00197 #if defined(TVMET_HAVE_COMPLEX) 00198 TVMET_IMPLEMENT_MACRO(polar) 00199 #endif 00200 00201 #undef TVMET_IMPLEMENT_MACRO 00202 00203 00204 /* 00205 * binary_function(XprVector<E, Sz>, POD) 00206 */ 00207 #define TVMET_IMPLEMENT_MACRO(NAME, TP) \ 00208 template<class E, std::size_t Sz> \ 00209 inline \ 00210 XprVector< \ 00211 XprBinOp< \ 00212 Fcnl_##NAME<typename E::value_type, TP >, \ 00213 XprVector<E, Sz>, \ 00214 XprLiteral< TP > \ 00215 >, \ 00216 Sz \ 00217 > \ 00218 NAME(const XprVector<E, Sz>& lhs, TP rhs) { \ 00219 typedef XprBinOp< \ 00220 Fcnl_##NAME<typename E::value_type, TP >, \ 00221 XprVector<E, Sz>, \ 00222 XprLiteral< TP > \ 00223 > expr_type; \ 00224 return XprVector<expr_type, Sz>( \ 00225 expr_type(lhs, XprLiteral< TP >(rhs))); \ 00226 } 00227 00228 TVMET_IMPLEMENT_MACRO(atan2, int) 00229 TVMET_IMPLEMENT_MACRO(drem, int) 00230 TVMET_IMPLEMENT_MACRO(fmod, int) 00231 TVMET_IMPLEMENT_MACRO(hypot, int) 00232 TVMET_IMPLEMENT_MACRO(jn, int) 00233 TVMET_IMPLEMENT_MACRO(yn, int) 00234 TVMET_IMPLEMENT_MACRO(pow, int) 00235 00236 #if defined(TVMET_HAVE_LONG_LONG) 00237 TVMET_IMPLEMENT_MACRO(atan2, long long int) 00238 TVMET_IMPLEMENT_MACRO(drem, long long int) 00239 TVMET_IMPLEMENT_MACRO(fmod, long long int) 00240 TVMET_IMPLEMENT_MACRO(hypot, long long int) 00241 TVMET_IMPLEMENT_MACRO(jn, long long int) 00242 TVMET_IMPLEMENT_MACRO(yn, long long int) 00243 TVMET_IMPLEMENT_MACRO(pow, long long int) 00244 #endif // defined(TVMET_HAVE_LONG_LONG) 00245 00246 TVMET_IMPLEMENT_MACRO(atan2, float) 00247 TVMET_IMPLEMENT_MACRO(drem, float) 00248 TVMET_IMPLEMENT_MACRO(fmod, float) 00249 TVMET_IMPLEMENT_MACRO(hypot, float) 00250 TVMET_IMPLEMENT_MACRO(jn, float) 00251 TVMET_IMPLEMENT_MACRO(yn, float) 00252 TVMET_IMPLEMENT_MACRO(pow, float) 00253 00254 TVMET_IMPLEMENT_MACRO(atan2, double) 00255 TVMET_IMPLEMENT_MACRO(drem, double) 00256 TVMET_IMPLEMENT_MACRO(fmod, double) 00257 TVMET_IMPLEMENT_MACRO(hypot, double) 00258 TVMET_IMPLEMENT_MACRO(jn, double) 00259 TVMET_IMPLEMENT_MACRO(yn, double) 00260 TVMET_IMPLEMENT_MACRO(pow, double) 00261 00262 #if defined(TVMET_HAVE_LONG_DOUBLE) 00263 TVMET_IMPLEMENT_MACRO(atan2, long double) 00264 TVMET_IMPLEMENT_MACRO(drem, long double) 00265 TVMET_IMPLEMENT_MACRO(fmod, long double) 00266 TVMET_IMPLEMENT_MACRO(hypot, long double) 00267 TVMET_IMPLEMENT_MACRO(jn, long double) 00268 TVMET_IMPLEMENT_MACRO(yn, long double) 00269 TVMET_IMPLEMENT_MACRO(pow, long double) 00270 #endif // defined(TVMET_HAVE_LONG_DOUBLE) 00271 00272 #undef TVMET_IMPLEMENT_MACRO 00273 00274 00275 #if defined(TVMET_HAVE_COMPLEX) 00276 /* 00277 * binary_function(XprVector<E, Sz>, std::complex<>) 00278 */ 00279 #define TVMET_IMPLEMENT_MACRO(NAME) \ 00280 template<class E, std::size_t Sz, class T> \ 00281 inline \ 00282 XprVector< \ 00283 XprBinOp< \ 00284 Fcnl_##NAME<typename E::value_type, std::complex<T> >, \ 00285 XprVector<E, Sz>, \ 00286 XprLiteral< std::complex<T> > \ 00287 >, \ 00288 Sz \ 00289 > \ 00290 NAME(const XprVector<E, Sz>& lhs, const std::complex<T>& rhs) { \ 00291 typedef XprBinOp< \ 00292 Fcnl_##NAME<typename E::value_type, std::complex<T> >, \ 00293 XprVector<E, Sz>, \ 00294 XprLiteral< std::complex<T> > \ 00295 > expr_type; \ 00296 return XprVector<expr_type, Sz>( \ 00297 expr_type(lhs, XprLiteral< std::complex<T> >(rhs))); \ 00298 } 00299 00300 TVMET_IMPLEMENT_MACRO(atan2) 00301 TVMET_IMPLEMENT_MACRO(drem) 00302 TVMET_IMPLEMENT_MACRO(fmod) 00303 TVMET_IMPLEMENT_MACRO(hypot) 00304 TVMET_IMPLEMENT_MACRO(jn) 00305 TVMET_IMPLEMENT_MACRO(yn) 00306 TVMET_IMPLEMENT_MACRO(pow) 00307 00308 #undef TVMET_IMPLEMENT_MACRO 00309 00310 #endif // defined(TVMET_HAVE_COMPLEX) 00311 00312 00313 } // namespace tvmet 00314 00315 #endif // TVMET_XPR_VECTOR_BINARY_FUNCTIONS_H 00316 00317 // Local Variables: 00318 // mode:C++ 00319 // tab-width:8 00320 // End:
Generated on Tue Jul 12 2022 18:57:56 by
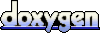