
This is some awesome robot code
Dependencies: mbed-rtos mbed QEI
Fork of ICRSEurobot13 by
system.cpp
00001 00002 #include "rtos.h" 00003 #include "mbed.h" 00004 #include "Printing.h" 00005 00006 Timer SystemTime; 00007 00008 Ticker CPUIdleMeasureTicker; 00009 volatile unsigned int nopctr = 0; 00010 const float s_per_nopcycle = 1.0f/16000000.0f; 00011 00012 float CpuUsage = 0; 00013 00014 void nopwait(int ms){ 00015 while(ms--) 00016 for (volatile int i = 0; i < 24000; i++); 00017 } 00018 00019 void PostAndResetCPUIdle(){ 00020 static int oldnopctr = 0; 00021 int deltanop = nopctr - oldnopctr; 00022 oldnopctr = nopctr; 00023 CpuUsage = 1.0f - (s_per_nopcycle * deltanop); 00024 Printing::updateval(10,CpuUsage); 00025 } 00026 00027 void measureCPUidle (void const*){ 00028 00029 osThreadSetPriority (osThreadGetId(), osPriorityIdle); 00030 Printing::registerID(10, 1); 00031 00032 CPUIdleMeasureTicker.attach(PostAndResetCPUIdle, 1); 00033 00034 while(1) 00035 nopctr++; 00036 }
Generated on Tue Jul 12 2022 18:57:56 by
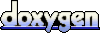