
This is some awesome robot code
Dependencies: mbed-rtos mbed QEI
Fork of ICRSEurobot13 by
globals.h
00001 00002 #ifndef GLOBALS_H 00003 #define GLOBALS_H 00004 00005 //#define TEAM_RED 00006 #define TEAM_BLUE 00007 00008 #include "mbed.h" 00009 00010 const float KALMAN_PREDICT_PERIOD = 0.05; //seconds 00011 00012 #define ENABLE_GLOBAL_ENCODERS 00013 00014 const float ENCODER_M_PER_TICK = 1.0f/1198.0f; 00015 const float ENCODER_WHEELBASE = 0.068; 00016 const float TURRET_FWD_PLACEMENT = -0.042; 00017 00018 //Robot movement constants 00019 const float fwdvarperunit = 0.0001; //1 std dev = 7% //TODO: measrue this!! 00020 const float varperang = 0.0003; //around 3 degree stddev per 180 turn //TODO: measrue this!! 00021 const float xyvarpertime = 0.000025; //(very poorly) accounts for hitting things 00022 const float angvarpertime = 0.0001; 00023 00024 const float MOTORCONTROLLER_FILTER_K = 0.5;// TODO: tune this 00025 const float MOTOR_MAX_POWER = 0.5f; 00026 00027 /* 00028 PINOUT Sensors 00029 5: RF:SDI 00030 6 SDO 00031 7 SCK 00032 8 NCS 00033 9 NIRQ 00034 10-15 6 echo pins 00035 16 trig 00036 17 IRin 00037 18-20 unused 00038 21 stepper step 00039 22-27 unused 00040 28 Serial TX 00041 29-30 unused 00042 00043 00044 PINOUT Main 00045 5: Lower arm servo 00046 6: Upper arm servo 00047 00048 14: Serial RX 00049 15: Cake distance sensor 00050 16: Fwd distance sensor 00051 00052 20: color sensor in 00053 21-24: Motors PWM IN 1-4 00054 25-26: Encoders 00055 27-28: Encoders 00056 29: Color sensor RED LED 00057 30: Color sensor BLUE LED 00058 00059 */ 00060 00061 const PinName P_SERVO_LOWER_ARM = p25; 00062 const PinName P_SERVO_UPPER_ARM = p26; 00063 00064 const PinName P_SERIAL_RX = p14; 00065 const PinName P_DISTANCE_SENSOR = p15; 00066 const PinName P_FWD_DISTANCE_SENSOR = p16; 00067 00068 const PinName P_COLOR_SENSOR_IN_UPPER = p20; 00069 const PinName P_COLOR_SENSOR_IN_LOWER = p19; 00070 00071 const PinName P_MOT_LEFT_A = p22; 00072 const PinName P_MOT_LEFT_B = p21; 00073 const PinName P_MOT_RIGHT_A = p24; 00074 const PinName P_MOT_RIGHT_B = p23; 00075 00076 const PinName P_ENC_RIGHT_A = p28;//p26; 00077 const PinName P_ENC_RIGHT_B = p27;//p25; 00078 const PinName P_ENC_LEFT_A = p29;//p27; 00079 const PinName P_ENC_LEFT_B = p30;//p28; 00080 00081 const PinName P_COLOR_SENSOR_RED_UPPER = p13;//p29; 00082 const PinName P_COLOR_SENSOR_BLUE_UPPER = p12;//p30; 00083 const PinName P_COLOR_SENSOR_RED_LOWER = p11; 00084 const PinName P_COLOR_SENSOR_BLUE_LOWER = p10; 00085 00086 const PinName P_START_CORD = p17; 00087 00088 const PinName P_BALLOON = p8; 00089 00090 00091 00092 //a type which is a pointer to a rtos thread function 00093 typedef void (*tfuncptr_t)(void const *argument); 00094 00095 //Solving for sonar bias is done by entering the following into wolfram alpha 00096 //(a-f)^2 = x^2 + y^2; (b-f)^2 = (x-3)^2 + y^2; (c-f)^2 = (x-1.5)^2+(y-2)^2: solve for x,y,f 00097 //where a, b, c are the measured distances, and f is the bias 00098 00099 //const float sonartimebias = 0; // TODO: measure and stick in the coprocessor code 00100 00101 struct pos { 00102 float x; 00103 float y; 00104 }; 00105 00106 extern pos beaconpos[3]; 00107 00108 const float PI = 3.14159265359; 00109 00110 typedef struct Waypoint 00111 { 00112 float x; 00113 float y; 00114 float theta; 00115 float pos_threshold; 00116 float angle_threshold; 00117 float angle_exponent; //temp hack 00118 } Waypoint; 00119 00120 typedef struct State 00121 { 00122 float x; 00123 float y; 00124 float theta; 00125 } State; 00126 00127 #endif //GLOBALS_H
Generated on Tue Jul 12 2022 18:57:56 by
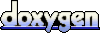