
This is some awesome robot code
Dependencies: mbed-rtos mbed QEI
Fork of ICRSEurobot13 by
VectorEval.h
00001 /* 00002 * Tiny Vector Matrix Library 00003 * Dense Vector Matrix Libary of Tiny size using Expression Templates 00004 * 00005 * Copyright (C) 2001 - 2007 Olaf Petzold <opetzold@users.sourceforge.net> 00006 * 00007 * This library is free software; you can redistribute it and/or 00008 * modify it under the terms of the GNU Lesser General Public 00009 * License as published by the Free Software Foundation; either 00010 * version 2.1 of the License, or (at your option) any later version. 00011 * 00012 * This library is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00015 * Lesser General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU Lesser General Public 00018 * License along with this library; if not, write to the Free Software 00019 * Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA 00020 * 00021 * $Id: VectorEval.h,v 1.18 2007-06-23 15:58:58 opetzold Exp $ 00022 */ 00023 00024 #ifndef TVMET_VECTOR_EVAL_H 00025 #define TVMET_VECTOR_EVAL_H 00026 00027 namespace tvmet { 00028 00029 00030 /******************************************************************** 00031 * functions all_elements/any_elements 00032 ********************************************************************/ 00033 00034 00035 /** 00036 * \fn bool all_elements(const XprVector<E, Sz>& e) 00037 * \brief check on statements for all elements 00038 * \ingroup _unary_function 00039 * This is for use with boolean operators like 00040 * \par Example: 00041 * \code 00042 * all_elements(vector > 0) { 00043 * // true branch 00044 * } else { 00045 * // false branch 00046 * } 00047 * \endcode 00048 * \sa \ref compare 00049 */ 00050 template<class E, std::size_t Sz> 00051 inline 00052 bool all_elements(const XprVector<E, Sz>& e) { 00053 return meta::Vector<Sz>::all_elements(e); 00054 } 00055 00056 00057 /** 00058 * \fn bool any_elements(const XprVector<E, Sz>& e) 00059 * \brief check on statements for any elements 00060 * \ingroup _unary_function 00061 * This is for use with boolean operators like 00062 * \par Example: 00063 * \code 00064 * any_elements(vector > 0) { 00065 * // true branch 00066 * } else { 00067 * // false branch 00068 * } 00069 * \endcode 00070 * \sa \ref compare 00071 */ 00072 template<class E, std::size_t Sz> 00073 inline 00074 bool any_elements(const XprVector<E, Sz>& e) { 00075 return meta::Vector<Sz>::any_elements(e); 00076 } 00077 00078 00079 /* 00080 * trinary evaluation functions with vectors and xpr of 00081 * XprVector<E1, Sz> ? Vector<T2, Sz> : Vector<T3, Sz> 00082 * XprVector<E1, Sz> ? Vector<T2, Sz> : XprVector<E3, Sz> 00083 * XprVector<E1, Sz> ? XprVector<E2, Sz> : Vector<T3, Sz> 00084 * XprVector<E1, Sz> ? XprVector<E2, Sz> : XprVector<E3, Sz> 00085 */ 00086 00087 /** 00088 * eval(const XprVector<E1, Sz>& e1, const Vector<T2, Sz>& v2, const Vector<T3, Sz>& v3) 00089 * \brief Evals the vector expressions. 00090 * \ingroup _trinary_function 00091 * This eval is for the a?b:c syntax, since it's not allowed to overload 00092 * these operators. 00093 */ 00094 template<class E1, class T2, class T3, std::size_t Sz> 00095 inline 00096 XprVector< 00097 XprEval< 00098 XprVector<E1, Sz>, 00099 VectorConstReference<T2, Sz>, 00100 VectorConstReference<T3, Sz> 00101 >, 00102 Sz 00103 > 00104 eval(const XprVector<E1, Sz>& e1, const Vector<T2, Sz>& v2, const Vector<T3, Sz>& v3) { 00105 typedef XprEval< 00106 XprVector<E1, Sz>, 00107 VectorConstReference<T2, Sz>, 00108 VectorConstReference<T3, Sz> 00109 > expr_type; 00110 return XprVector<expr_type, Sz>( 00111 expr_type(e1, v2.const_ref(), v3.const_ref())); 00112 } 00113 00114 00115 /** 00116 * eval(const XprVector<E1, Sz>& e1, const Vector<T2, Sz>& v2, const XprVector<E3, Sz>& e3) 00117 * \brief Evals the vector expressions. 00118 * \ingroup _trinary_function 00119 * This eval is for the a?b:c syntax, since it's not allowed to overload 00120 * these operators. 00121 */ 00122 template<class E1, class T2, class E3, std::size_t Sz> 00123 inline 00124 XprVector< 00125 XprEval< 00126 XprVector<E1, Sz>, 00127 VectorConstReference<T2, Sz>, 00128 XprVector<E3, Sz> 00129 >, 00130 Sz 00131 > 00132 eval(const XprVector<E1, Sz>& e1, const Vector<T2, Sz>& v2, const XprVector<E3, Sz>& e3) { 00133 typedef XprEval< 00134 XprVector<E1, Sz>, 00135 VectorConstReference<T2, Sz>, 00136 XprVector<E3, Sz> 00137 > expr_type; 00138 return XprVector<expr_type, Sz>( 00139 expr_type(e1, v2.const_ref(), e3)); 00140 } 00141 00142 00143 /** 00144 * eval(const XprVector<E1, Sz>& e1, const XprVector<E2, Sz>& e2, const Vector<T3, Sz>& v3) 00145 * \brief Evals the vector expressions. 00146 * \ingroup _trinary_function 00147 * This eval is for the a?b:c syntax, since it's not allowed to overload 00148 * these operators. 00149 */ 00150 template<class E1, class E2, class T3, std::size_t Sz> 00151 inline 00152 XprVector< 00153 XprEval< 00154 XprVector<E1, Sz>, 00155 XprVector<E2, Sz>, 00156 VectorConstReference<T3, Sz> 00157 >, 00158 Sz 00159 > 00160 eval(const XprVector<E1, Sz>& e1, const XprVector<E2, Sz>& e2, const Vector<T3, Sz>& v3) { 00161 typedef XprEval< 00162 XprVector<E1, Sz>, 00163 XprVector<E2, Sz>, 00164 VectorConstReference<T3, Sz> 00165 > expr_type; 00166 return XprVector<expr_type, Sz>( 00167 expr_type(e1, e2, v3.const_ref())); 00168 } 00169 00170 00171 /** 00172 * eval(const XprVector<E1, Sz>& e1, const XprVector<E2, Sz>& e2, const XprVector<E3, Sz>& e3) 00173 * \brief Evals the vector expressions. 00174 * \ingroup _trinary_function 00175 * This eval is for the a?b:c syntax, since it's not allowed to overload 00176 * these operators. 00177 */ 00178 template<class E1, class E2, class E3, std::size_t Sz> 00179 inline 00180 XprVector< 00181 XprEval< 00182 XprVector<E1, Sz>, 00183 XprVector<E2, Sz>, 00184 XprVector<E3, Sz> 00185 >, 00186 Sz 00187 > 00188 eval(const XprVector<E1, Sz>& e1, const XprVector<E2, Sz>& e2, const XprVector<E3, Sz>& e3) { 00189 typedef XprEval< 00190 XprVector<E1, Sz>, 00191 XprVector<E2, Sz>, 00192 XprVector<E3, Sz> 00193 > expr_type; 00194 return XprVector<expr_type, Sz>(expr_type(e1, e2, e3)); 00195 } 00196 00197 00198 /* 00199 * trinary evaluation functions with vectors, xpr of and POD 00200 * 00201 * XprVector<E, Sz> ? POD1 : POD2 00202 * XprVector<E1, Sz> ? POD : XprVector<E3, Sz> 00203 * XprVector<E1, Sz> ? XprVector<E2, Sz> : POD 00204 */ 00205 #define TVMET_IMPLEMENT_MACRO(POD) \ 00206 template<class E, std::size_t Sz> \ 00207 inline \ 00208 XprVector< \ 00209 XprEval< \ 00210 XprVector<E, Sz>, \ 00211 XprLiteral< POD >, \ 00212 XprLiteral< POD > \ 00213 >, \ 00214 Sz \ 00215 > \ 00216 eval(const XprVector<E, Sz>& e, POD x2, POD x3) { \ 00217 typedef XprEval< \ 00218 XprVector<E, Sz>, \ 00219 XprLiteral< POD >, \ 00220 XprLiteral< POD > \ 00221 > expr_type; \ 00222 return XprVector<expr_type, Sz>( \ 00223 expr_type(e, XprLiteral< POD >(x2), XprLiteral< POD >(x3))); \ 00224 } \ 00225 \ 00226 template<class E1, class E3, std::size_t Sz> \ 00227 inline \ 00228 XprVector< \ 00229 XprEval< \ 00230 XprVector<E1, Sz>, \ 00231 XprLiteral< POD >, \ 00232 XprVector<E3, Sz> \ 00233 >, \ 00234 Sz \ 00235 > \ 00236 eval(const XprVector<E1, Sz>& e1, POD x2, const XprVector<E3, Sz>& e3) { \ 00237 typedef XprEval< \ 00238 XprVector<E1, Sz>, \ 00239 XprLiteral< POD >, \ 00240 XprVector<E3, Sz> \ 00241 > expr_type; \ 00242 return XprVector<expr_type, Sz>( \ 00243 expr_type(e1, XprLiteral< POD >(x2), e3)); \ 00244 } \ 00245 \ 00246 template<class E1, class E2, std::size_t Sz> \ 00247 inline \ 00248 XprVector< \ 00249 XprEval< \ 00250 XprVector<E1, Sz>, \ 00251 XprVector<E2, Sz>, \ 00252 XprLiteral< POD > \ 00253 >, \ 00254 Sz \ 00255 > \ 00256 eval(const XprVector<E1, Sz>& e1, const XprVector<E2, Sz>& e2, POD x3) { \ 00257 typedef XprEval< \ 00258 XprVector<E1, Sz>, \ 00259 XprVector<E2, Sz>, \ 00260 XprLiteral< POD > \ 00261 > expr_type; \ 00262 return XprVector<expr_type, Sz>( \ 00263 expr_type(e1, e2, XprLiteral< POD >(x3))); \ 00264 } 00265 00266 TVMET_IMPLEMENT_MACRO(int) 00267 00268 #if defined(TVMET_HAVE_LONG_LONG) 00269 TVMET_IMPLEMENT_MACRO(long long int) 00270 #endif // defined(TVMET_HAVE_LONG_LONG) 00271 00272 TVMET_IMPLEMENT_MACRO(float) 00273 TVMET_IMPLEMENT_MACRO(double) 00274 00275 #if defined(TVMET_HAVE_LONG_DOUBLE) 00276 TVMET_IMPLEMENT_MACRO(long double) 00277 #endif // defined(TVMET_HAVE_LONG_DOUBLE) 00278 00279 #undef TVMET_IMPLEMENT_MACRO 00280 00281 00282 /* 00283 * trinary evaluation functions with vectors, xpr of and complex<> types 00284 * 00285 * XprVector<E, Sz> e, std::complex<T> z2, std::complex<T> z3 00286 * XprVector<E1, Sz> e1, std::complex<T> z2, XprVector<E3, Sz> e3 00287 * XprVector<E1, Sz> e1, XprVector<E2, Sz> e2, std::complex<T> z3 00288 */ 00289 #if defined(TVMET_HAVE_COMPLEX) 00290 00291 00292 /** 00293 * eval(const XprVector<E, Sz>& e, std::complex<T> z2, std::complex<T> z3) 00294 * \brief Evals the vector expressions. 00295 * \ingroup _trinary_function 00296 * This eval is for the a?b:c syntax, since it's not allowed to overload 00297 * these operators. 00298 */ 00299 template<class E, std::size_t Sz, class T> 00300 inline 00301 XprVector< 00302 XprEval< 00303 XprVector<E, Sz>, 00304 XprLiteral< std::complex<T> >, 00305 XprLiteral< std::complex<T> > 00306 >, 00307 Sz 00308 > 00309 eval(const XprVector<E, Sz>& e, std::complex<T> z2, std::complex<T> z3) { 00310 typedef XprEval< 00311 XprVector<E, Sz>, 00312 XprLiteral< std::complex<T> >, 00313 XprLiteral< std::complex<T> > 00314 > expr_type; 00315 return XprVector<expr_type, Sz>( 00316 expr_type(e, XprLiteral< std::complex<T> >(z2), XprLiteral< std::complex<T> >(z3))); 00317 } 00318 00319 /** 00320 * eval(const XprVector<E1, Sz>& e1, std::complex<T> z2, const XprVector<E3, Sz>& e3) 00321 * \brief Evals the vector expressions. 00322 * \ingroup _trinary_function 00323 * This eval is for the a?b:c syntax, since it's not allowed to overload 00324 * these operators. 00325 */ 00326 template<class E1, class E3, std::size_t Sz, class T> 00327 inline 00328 XprVector< 00329 XprEval< 00330 XprVector<E1, Sz>, 00331 XprLiteral< std::complex<T> >, 00332 XprVector<E3, Sz> 00333 >, 00334 Sz 00335 > 00336 eval(const XprVector<E1, Sz>& e1, std::complex<T> z2, const XprVector<E3, Sz>& e3) { 00337 typedef XprEval< 00338 XprVector<E1, Sz>, 00339 XprLiteral< std::complex<T> >, 00340 XprVector<E3, Sz> 00341 > expr_type; 00342 return XprVector<expr_type, Sz>( 00343 expr_type(e1, XprLiteral< std::complex<T> >(z2), e3)); 00344 } 00345 00346 /** 00347 * eval(const XprVector<E1, Sz>& e1, const XprVector<E2, Sz>& e2, std::complex<T> z3) 00348 * \brief Evals the vector expressions. 00349 * \ingroup _trinary_function 00350 * This eval is for the a?b:c syntax, since it's not allowed to overload 00351 * these operators. 00352 */ 00353 template<class E1, class E2, std::size_t Sz, class T> 00354 inline 00355 XprVector< 00356 XprEval< 00357 XprVector<E1, Sz>, 00358 XprVector<E2, Sz>, 00359 XprLiteral< std::complex<T> > 00360 >, 00361 Sz 00362 > 00363 eval(const XprVector<E1, Sz>& e1, const XprVector<E2, Sz>& e2, std::complex<T> z3) { 00364 typedef XprEval< 00365 XprVector<E1, Sz>, 00366 XprVector<E2, Sz>, 00367 XprLiteral< std::complex<T> > 00368 > expr_type; 00369 return XprVector<expr_type, Sz>( 00370 expr_type(e1, e2, XprLiteral< std::complex<T> >(z3))); 00371 } 00372 #endif // defined(TVMET_HAVE_COMPLEX) 00373 00374 00375 } // namespace tvmet 00376 00377 #endif // TVMET_VECTOR_EVAL_H 00378 00379 // Local Variables: 00380 // mode:C++ 00381 // tab-width:8 00382 // End:
Generated on Tue Jul 12 2022 18:57:56 by
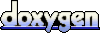