
This is some awesome robot code
Dependencies: mbed-rtos mbed QEI
Fork of ICRSEurobot13 by
TvmetBase.h
00001 /* 00002 * Tiny Vector Matrix Library 00003 * Dense Vector Matrix Libary of Tiny size using Expression Templates 00004 * 00005 * Copyright (C) 2001 - 2007 Olaf Petzold <opetzold@users.sourceforge.net> 00006 * 00007 * This library is free software; you can redistribute it and/or 00008 * modify it under the terms of the GNU Lesser General Public 00009 * License as published by the Free Software Foundation; either 00010 * version 2.1 of the License, or (at your option) any later version. 00011 * 00012 * This library is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00015 * Lesser General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU Lesser General Public 00018 * License along with this library; if not, write to the Free Software 00019 * Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA 00020 * 00021 * $Id: TvmetBase.h,v 1.17 2007-06-23 15:58:58 opetzold Exp $ 00022 */ 00023 00024 #ifndef TVMET_BASE_H 00025 #define TVMET_BASE_H 00026 00027 #include <iostream>//<iosfwd> // io streams forward declaration 00028 #include <typeinfo> // rtti: used by Xpr.h level printing 00029 #include <cmath> // unary and binary math 00030 #include <cstdlib> // labs 00031 00032 #if defined(WIN32) && defined(_MSC_VER) && (_MSC_VER == 1310) 00033 #include <string> // operator<<(ostream) here defined 00034 #endif 00035 00036 #if defined(__APPLE_CC__) 00037 // Mac OS X builds seems to miss these functions inside cmath 00038 extern "C" int isnan(double); 00039 extern "C" int isinf(double); 00040 #endif 00041 00042 namespace tvmet { 00043 00044 00045 /** 00046 * \class TvmetBase TvmetBase.h "tvmet/TvmetBase.h" 00047 * \brief Base class 00048 * Used for static polymorph call of print_xpr 00049 */ 00050 template<class E> class TvmetBase { }; 00051 00052 00053 /** 00054 * \class IndentLevel TvmetBase.h "tvmet/TvmetBase.h" 00055 * \brief Prints the level indent. 00056 */ 00057 class IndentLevel : public TvmetBase< IndentLevel > 00058 { 00059 public: 00060 IndentLevel(std::size_t level) : m_level(level) { } 00061 00062 std::ostream& print_xpr(std::ostream& os) const { 00063 for(std::size_t i = 0; i != m_level; ++i) os << " "; 00064 return os; 00065 } 00066 00067 private: 00068 std::size_t m_level; 00069 }; 00070 00071 00072 /** 00073 * \fn operator<<(std::ostream& os, const TvmetBase<E>& e) 00074 * \brief overloaded ostream operator using static polymorphic. 00075 * \ingroup _binary_operator 00076 */ 00077 template<class E> 00078 inline 00079 std::ostream& operator<<(std::ostream& os, const TvmetBase<E>& e) { 00080 static_cast<const E&>(e).print_xpr(os); 00081 return os; 00082 } 00083 00084 00085 /** 00086 * \class dispatch TvmetBase.h "tvmet/TvmetBase.h" 00087 * \brief Class helper to distuingish between e.g. meta 00088 * and loop strategy used. 00089 */ 00090 template<bool> struct dispatch; 00091 00092 /** 00093 * \class dispatch<true> TvmetBase.h "tvmet/TvmetBase.h" 00094 * \brief specialized. 00095 */ 00096 template<> struct dispatch<true> { }; 00097 00098 /** 00099 * \class dispatch<false> TvmetBase.h "tvmet/TvmetBase.h" 00100 * \brief specialized. 00101 */ 00102 template<> struct dispatch<false> { }; 00103 00104 00105 } // namespace tvmet 00106 00107 #endif // TVMET_BASE_H 00108 00109 // Local Variables: 00110 // mode:C++ 00111 // tab-width:8 00112 // End:
Generated on Tue Jul 12 2022 18:57:56 by
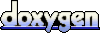