
This is some awesome robot code
Dependencies: mbed-rtos mbed QEI
Fork of ICRSEurobot13 by
Timer.h
00001 /* 00002 * Tiny Vector Matrix Library 00003 * Dense Vector Matrix Libary of Tiny size using Expression Templates 00004 * 00005 * Copyright (C) 2001 - 2007 Olaf Petzold <opetzold@users.sourceforge.net> 00006 * 00007 * This library is free software; you can redistribute it and/or 00008 * modify it under the terms of the GNU Lesser General Public 00009 * License as published by the Free Software Foundation; either 00010 * version 2.1 of the License, or (at your option) any later version. 00011 * 00012 * This library is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00015 * Lesser General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU Lesser General Public 00018 * License along with this library; if not, write to the Free Software 00019 * Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA 00020 * 00021 * $Id: Timer.h,v 1.9 2007-06-23 15:58:59 opetzold Exp $ 00022 */ 00023 00024 #ifndef TVMET_UTIL_TIMER_H 00025 #define TVMET_UTIL_TIMER_H 00026 00027 #if defined(TVMET_HAVE_SYS_TIME_H) && defined(TVMET_HAVE_UNISTD_H) 00028 # include <sys/time.h> 00029 # include <sys/resource.h> 00030 # include <unistd.h> 00031 #else 00032 # include <ctime> 00033 #endif 00034 00035 namespace tvmet { 00036 00037 namespace util { 00038 00039 /** 00040 \class Timer Timer.h "tvmet/util/Timer.h" 00041 \brief A quick& dirty portable timer, measures elapsed time. 00042 00043 It is recommended that implementations measure wall clock rather than CPU 00044 time since the intended use is performance measurement on systems where 00045 total elapsed time is more important than just process or CPU time. 00046 00047 The accuracy of timings depends on the accuracy of timing information 00048 provided by the underlying platform, and this varies from platform to 00049 platform. 00050 */ 00051 00052 class Timer 00053 { 00054 Timer(const Timer&); 00055 Timer& operator=(const Timer&); 00056 00057 public: // types 00058 typedef double time_t; 00059 00060 public: 00061 /** starts the timer immediatly. */ 00062 Timer() { m_start_time = getTime(); } 00063 00064 /** restarts the timer */ 00065 void restart() { m_start_time = getTime(); } 00066 00067 /** return elapsed time in seconds */ 00068 time_t elapsed() const { return (getTime() - m_start_time); } 00069 00070 private: 00071 time_t getTime() const { 00072 #if defined(TVMET_HAVE_SYS_TIME_H) && defined(TVMET_HAVE_UNISTD_H) 00073 getrusage(RUSAGE_SELF, &m_rusage); 00074 time_t sec = m_rusage.ru_utime.tv_sec; // user, no system time 00075 time_t usec = m_rusage.ru_utime.tv_usec; // user, no system time 00076 return sec + usec/1e6; 00077 #else 00078 return static_cast<time_t>(std::clock()) / static_cast<time_t>(CLOCKS_PER_SEC); 00079 #endif 00080 } 00081 00082 private: 00083 #if defined(TVMET_HAVE_SYS_TIME_H) && defined(TVMET_HAVE_UNISTD_H) 00084 mutable struct rusage m_rusage; 00085 #endif 00086 time_t m_start_time; 00087 }; 00088 00089 } // namespace util 00090 00091 } // namespace tvmet 00092 00093 #endif // TVMET_UTIL_TIMER_H 00094 00095 // Local Variables: 00096 // mode:C++ 00097 // tab-width:8 00098 // End:
Generated on Tue Jul 12 2022 18:57:56 by
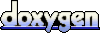