
This is some awesome robot code
Dependencies: mbed-rtos mbed QEI
Fork of ICRSEurobot13 by
Kalman.h
00001 #ifndef KALMAN_H 00002 #define KALMAN_H 00003 00004 #include "globals.h" 00005 #include "rtos.h" 00006 00007 namespace Kalman 00008 { 00009 00010 //Accessor function to get the state as one consistent struct 00011 State getState(); 00012 00013 //Main loops (to be attached as a thread in main) 00014 void predictloop(void const*); 00015 void updateloop(void const*); 00016 00017 void start_predict_ticker(Thread* predict_thread_ptr_in); 00018 00019 enum measurement_t {SONAR0 = 0, SONAR1, SONAR2, IR0, IR1, IR2}; 00020 const measurement_t maxmeasure = IR2; 00021 00022 //Call this to run an update 00023 void runupdate(measurement_t type, float value, float variance); 00024 00025 extern float RawReadings[maxmeasure+1]; 00026 00027 extern bool Kalman_inited; 00028 00029 //Initialises the kalman filter 00030 void KalmanInit(); 00031 00032 } 00033 00034 #endif //KALMAN_H
Generated on Tue Jul 12 2022 18:57:56 by
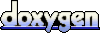