
This is some awesome robot code
Dependencies: mbed-rtos mbed QEI
Fork of ICRSEurobot13 by
Colour.h
00001 00002 // Eurobot13 Colour.h 00003 #ifndef COLOUR_H 00004 #define COLOUR_H 00005 00006 #include "mbed.h" 00007 #include "globals.h" 00008 #include "math.h" 00009 00010 #define BUFF_SIZE 10 00011 #define SNR_THRESHOLD_DB 4 00012 00013 #define UPPERARM_CORRECTION 2.310f 00014 #define LOWERARM_CORRECTION 1.000f 00015 00016 00017 enum ColourEnum {BLUE=0, RED, WHITE, BLACK}; 00018 enum ArmEnum {UPPER=0, LOWER}; 00019 00020 class Colour{ 00021 public: 00022 00023 Colour( 00024 PinName blue_led, 00025 PinName red_led, 00026 PinName pt, 00027 ArmEnum arm); 00028 00029 ColourEnum getColour(); 00030 00031 00032 private: 00033 Ticker ticker; 00034 DigitalOut blue_led; 00035 DigitalOut red_led; 00036 AnalogIn pt; 00037 ArmEnum arm; 00038 00039 float red_correction_factor; 00040 float colour; 00041 float SNR; 00042 void Blink(); 00043 00044 int togglecolour; 00045 float blue; 00046 float blue_buff[BUFF_SIZE]; 00047 float red; 00048 float red_buff[BUFF_SIZE]; 00049 float noise; 00050 float noise_buff[BUFF_SIZE]; 00051 00052 int buff_pointer; 00053 00054 }; 00055 00056 #endif
Generated on Tue Jul 12 2022 18:57:56 by
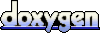