
This is some awesome robot code
Dependencies: mbed-rtos mbed QEI
Fork of ICRSEurobot13 by
Arm.h
00001 #ifndef EUROBOT_ACTUATORS_ARMS_ARM_H_ 00002 #define EUROBOT_ACTUATORS_ARMS_ARM_H_ 00003 00004 #include "mbed.h" 00005 00006 namespace arm 00007 { 00008 00009 class Arm; 00010 00011 extern Arm lower_arm; 00012 extern Arm upper_arm; 00013 00014 00015 class Arm 00016 { 00017 public: 00018 Arm (PwmOut pwm_in, float period_in, float min_pos_in, float max_pos_in) 00019 : pwm_(pwm_in), period_(period_in), min_pos_(min_pos_in), max_pos_(max_pos_in) 00020 { 00021 pwm_.period(period_); 00022 } 00023 00024 void go_up() 00025 { 00026 pwm_.pulsewidth_us(max_pos_*1000); 00027 } 00028 00029 void go_down() 00030 { 00031 pwm_.pulsewidth_us(min_pos_*1000); 00032 } 00033 00034 private: 00035 PwmOut pwm_; 00036 float period_; 00037 float min_pos_; 00038 float max_pos_; 00039 00040 }; 00041 00042 } //namespace 00043 00044 #endif
Generated on Tue Jul 12 2022 18:57:56 by
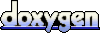